Convert void* to double
Solution 1
void *num
is a pointer. double n
is a variable.
You can cast void*
to double*
:
double *p = (double *)num;
Then you dereference it:
double n = *p;
Or in one line:
double n = *(double *)num;
Solution 2
First you want to convert the void* to a double*. Then you need to dereference the pointer to assign it to a double.
void *calc_fib( void *num)
{
double n;
n = *(double*)num;
...
Solution 3
You can't portably cast the double
to a pointer - for a start, the pointer type may not have enough bits.
If n
won't be changed in main
after calling pthread_create
, you can pass a pointer to the n
variable in main
itself (i.e. (void*)&n
, then use *(double*)num
inside calc_fib
).
If n
might change - perhaps you've a loop calculating a variety of n values to pass to different threads you spawn - then you must avoid a race condition where the new thread might not have read the value before the main thread replaces it. You could use a mutex for this - it means there's a fixed amount of memory needed independent of the number of threads - but the code's relatively verbose and it further serialises and slows down the launching of the threads. A typically better approach is to put each value into a separate memory address. You could use an array with as many values as you'll have new threads, or allocate memory on the heap for each double
then let the threads read then release that memory....
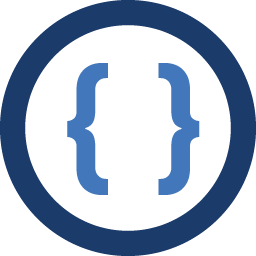
Admin
Updated on August 13, 2022Comments
-
Admin over 1 year
I am attempting to use the
pthread
library to calculaten
fibonacci numbers wheren
can be from range0-1000
. I am running in to a weird error when I try to typecast myvoid*
to adouble
.In my main here is my call to my calculate fibonacci function:
pthread_create(&tid, &attr, calc_fib, (void *)n);
In my
calc_fib
function I am attempting to typecast with:void *calc_fib( void *num) { double n; n = (double)num; ...
However, when I attempt to do this I get the following errors:
In function ‘calc_fib’: error: pointer value used where a floating point value was expected In function ‘main’: error: cannot convert to a pointer type
Am I not able to typecast from
void*
todouble
in C or am I just doing it wrong?