Convert WCHAR[260] to std::string
17,800
Solution 1
Your call to WideCharToMultiByte
looks correct, provided ch
is a
sufficiently large buffer. After than, however, you want to assign the
buffer (ch
) to the string (or use it to construct a string), not
pe32.szExeFile
.
Solution 2
For your first example you can just do:
std::wstring s(pe32.szExeFile);
and for second:
char DefChar = ' ';
WideCharToMultiByte(CP_ACP,0,pe32.szExeFile,-1, ch,260,&DefChar, NULL);
std::wstring s(pe32.szExeFile);
as std::wstring
has a char*
ctor
Solution 3
#ifndef __STRINGCAST_H__
#define __STRINGCAST_H__
#include <vector>
#include <string>
#include <cstring>
#include <cwchar>
#include <cassert>
template<typename Td>
Td string_cast(const wchar_t* pSource, unsigned int codePage = CP_ACP);
#endif // __STRINGCAST_H__
template<>
std::string string_cast( const wchar_t* pSource, unsigned int codePage )
{
assert(pSource != 0);
size_t sourceLength = std::wcslen(pSource);
if(sourceLength > 0)
{
int length = ::WideCharToMultiByte(codePage, 0, pSource, sourceLength, NULL, 0, NULL, NULL);
if(length == 0)
return std::string();
std::vector<char> buffer( length );
::WideCharToMultiByte(codePage, 0, pSource, sourceLength, &buffer[0], length, NULL, NULL);
return std::string(buffer.begin(), buffer.end());
}
else
return std::string();
}
and use this template as followed
PWSTR CurWorkDir;
std::string CurWorkLogFile;
CurWorkDir = new WCHAR[length];
CurWorkLogFile = string_cast<std::string>(CurWorkDir);
....
delete [] CurWorkDir;
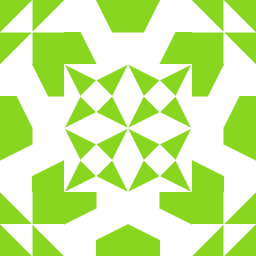
Author by
user1334943
Updated on June 13, 2022Comments
-
user1334943 7 months
I have gotten a WCHAR[MAX_PATH] from (PROCESSENTRY32) pe32.szExeFile on Windows. The following do not work:
std::string s; s = pe32.szExeFile; // compile error. cast (const char*) doesnt work either
and
std::string s; char DefChar = ' '; WideCharToMultiByte(CP_ACP,0,pe32.szExeFile,-1, ch,260,&DefChar, NULL); s = pe32.szExeFile;
-
user1334943 over 10 yearsNeither work same error. error: C2664: 'std::basic_string<_Elem,_Traits,_Ax>::basic_string(std::basic_string<_Elem,_Traits,_Ax>::_Has_debug_it)' : cannot convert parameter 1 from 'WCHAR [260]' to 'std::basic_string<_Elem,_Traits,_Ax>
-
EdChum over 10 yearssorry should've used wstring version, should compile now
-
EdChum over 10 years@user1334943 does it work with
std::wstring s(&pe32.szExeFile)
?