Converting a String to DateTime
Solution 1
Since you are handling 24-hour based time and you have a comma separating the seconds fraction, I recommend that you specify a custom format:
DateTime myDate = DateTime.ParseExact("2009-05-08 14:40:52,531", "yyyy-MM-dd HH:mm:ss,fff",
System.Globalization.CultureInfo.InvariantCulture);
Solution 2
You have basically two options for this. DateTime.Parse()
and DateTime.ParseExact()
.
The first is very forgiving in terms of syntax and will parse dates in many different formats. It is good for user input which may come in different formats.
ParseExact will allow you to specify the exact format of your date string to use for parsing. It is good to use this if your string is always in the same format. This way, you can easily detect any deviations from the expected data.
You can parse user input like this:
DateTime enteredDate = DateTime.Parse(enteredString);
If you have a specific format for the string, you should use the other method:
DateTime loadedDate = DateTime.ParseExact(loadedString, "d", null);
"d"
stands for the short date pattern (see MSDN for more info) and null
specifies that the current culture should be used for parsing the string.
Solution 3
try this
DateTime myDate = DateTime.Parse(dateString);
a better way would be this:
DateTime myDate;
if (!DateTime.TryParse(dateString, out myDate))
{
// handle parse failure
}
Solution 4
DateTime dateTime = DateTime.Parse(dateTimeStr);
Solution 5
Nobody seems to implemented an extension method. With the help of @CMS's answer:
Working and improved full source example is here: Gist Link
namespace ExtensionMethods {
using System;
using System.Globalization;
public static class DateTimeExtensions {
public static DateTime ToDateTime(this string s,
string format = "ddMMyyyy", string cultureString = "tr-TR") {
try {
var r = DateTime.ParseExact(
s: s,
format: format,
provider: CultureInfo.GetCultureInfo(cultureString));
return r;
} catch (FormatException) {
throw;
} catch (CultureNotFoundException) {
throw; // Given Culture is not supported culture
}
}
public static DateTime ToDateTime(this string s,
string format, CultureInfo culture) {
try {
var r = DateTime.ParseExact(s: s, format: format,
provider: culture);
return r;
} catch (FormatException) {
throw;
} catch (CultureNotFoundException) {
throw; // Given Culture is not supported culture
}
}
}
}
namespace SO {
using ExtensionMethods;
using System;
using System.Globalization;
class Program {
static void Main(string[] args) {
var mydate = "29021996";
var date = mydate.ToDateTime(format: "ddMMyyyy"); // {29.02.1996 00:00:00}
mydate = "2016 3";
date = mydate.ToDateTime("yyyy M"); // {01.03.2016 00:00:00}
mydate = "2016 12";
date = mydate.ToDateTime("yyyy d"); // {12.01.2016 00:00:00}
mydate = "2016/31/05 13:33";
date = mydate.ToDateTime("yyyy/d/M HH:mm"); // {31.05.2016 13:33:00}
mydate = "2016/31 Ocak";
date = mydate.ToDateTime("yyyy/d MMMM"); // {31.01.2016 00:00:00}
mydate = "2016/31 January";
date = mydate.ToDateTime("yyyy/d MMMM", cultureString: "en-US");
// {31.01.2016 00:00:00}
mydate = "11/شعبان/1437";
date = mydate.ToDateTime(
culture: CultureInfo.GetCultureInfo("ar-SA"),
format: "dd/MMMM/yyyy");
// Weird :) I supposed dd/yyyy/MMMM but that did not work !?$^&*
System.Diagnostics.Debug.Assert(
date.Equals(new DateTime(year: 2016, month: 5, day: 18)));
}
}
}
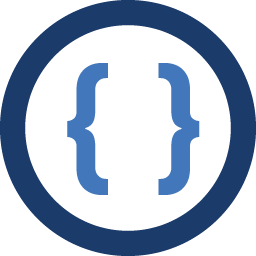
Admin
Updated on July 16, 2022Comments
-
Admin almost 2 years
How do you convert a string such as
2009-05-08 14:40:52,531
into aDateTime
? -
lc. almost 15 years(I think you meant to use a comma in the date and format strings, though, right?)
-
hamish over 9 yearsIt is only a comma because of the OPs European Locale setting, what if you take that code to another server with a US.Locale, then the fractions of a section will be a decimal not a comma on the saved string, and your solution will break. Make sure you add a check for the type of incoming datetime string for its correct Locale before applying the correct parser. I'm surprised that Microsoft doesn't already have this code prebuild somewhere else in the CLR or C#.net
-
Badhon Jain over 9 yearsYou missed the time part? I need both date & time, How can I do that?
-
Vinod Kumar almost 8 yearsunable to convert this to date time string MyString = "06/22/1916 3:20:14 PM";
-
T.Todua over 6 yearsNo one mentioned that it only works with that particular format.
-
jpaugh over 5 years24-hour time and comma as a decimal separator is not a custom locale. It shouldn't need to be handled specially.
-
Krishna over 5 yearsPity... 🙂 Coders always think fellow coders will figure out... Good thing actually... Make us think more...
-
Yousha Aleayoub over 4 years
Nobody seems to implemented an extension method
maybe because not needed... -
guneysus over 4 yearsSometimes standart library does not fit our needs. And this is why need/use helper libraries. Using the extension method way, or fluent API ise kinda prefering FP over OOP or vice versa. Neither correct nor wrong. It is choice. @YoushaAleayoub
-
Birhan Nega almost 4 yearsLooks better, can assign its value to the DateTime variable?
-
esnezz almost 2 yearsUnder the hood Convert.ToDateTime simply calls DateTime.Parse