Converting from string to int in Swift
Solution 1
A text field contains a string (in its text
property); it is not itself a string. And since that string can change, you should ask the text field for it each time you need it.
You can use a guard let
statement to unwrap all of the optionals (since each text field's text
is optional, and the Int
conversion also returns optional), like this:
class ViewController {
@IBOutlet weak var firstNumber: UITextField!
@IBOutlet weak var secondNumber: UITextField!
@IBOutlet weak var total: UILabel!
@IBOutlet weak var segmentedControlCalculate: UISegmentedControl!
@IBAction func segmentedControlAddSubtract(sender: AnyObject) {
switch segmentedControlCalculate.selectedSegmentIndex {
case 0:
operation = (+)
case 1:
operation = (-)
default:
return
}
updateTotalLabel()
}
private var operation: ((Int, Int) -> Int)?
private func updateTotalLabel() {
guard let operation = operation,
firstString = firstNumber.text,
firstInput = Int(firstString),
secondString = secondNumber.text,
secondInput = Int(secondString)
else {
total.text = "Bogus input"
return
}
let result = operation(firstInput, secondInput)
total.text = "Total = \(result)"
}
}
Solution 2
let text1 = "4"
let text2 = "3"
let a = Int(text1) //4
let b = Int(text2) //3
let c = a! + b! //7
print(a) //"Optional(4)\n"
print(c) //7
Be sure to unwrap optionals. Converting a String to an Int may not lead to an Int and hence the type is optional. OF course this is a simple example. You need to first test if a real Int was entered.
Solution 3
So you must understand that's type mismatch on string to int is more then of int to char. During the convert you may loose some bits, but as I understand we pushed to use that :) For using as integer u can just initialize a new var with constructor.
var string = "1234"
var number = Int(string)
You must to check the type of return of property inputs, if its a string. Try to isolate strings and then convert, because maybe you re converting object property but not a data :)
Solution 4
Your calculation part should be like this
@IBAction func segmentedControlAddSubtract(sender: AnyObject) {
// You should assign value in this stage, because there might still no value for textfield initially.
firstInput = Int(firstNumber.text)
secondInput = Int(secondNumber.text)
switch segmentedControlCalculate.selectedSegmentIndex {
case 0:
result = firstInput+ secondInput
case 1:
result = firstInput - secondInput
default:
return
}
updateTotalLabel()
}
You should assign your variable inside action, and not at the beginning
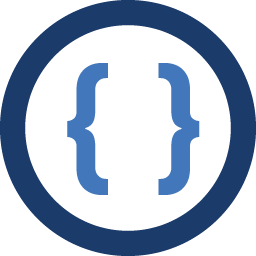
Admin
Updated on June 04, 2022Comments
-
Admin almost 2 years
So, I have looked at all the other questions regarding this and I've attempted to implement the suggested solutions, but to no avail. I have two text fields in a view controller that a user enters numbers into and is then allowed to perform either an addition or subtraction of the two numbers. Unless I'm mistaken, the text fields are strings and need to be converted to integers before the calculation can be done. I'm not having any luck with that conversion though. Any suggestions in the right direction would be much appreciated. Here is the applicable code:
let firstInput : Int? = Int(firstNumber) let secondInput : Int? = Int(secondNumber) var result = 0 @IBOutlet weak var firstNumber: UITextField! @IBOutlet weak var secondNumber: UITextField! @IBOutlet weak var segmentedControlCalculate: UISegmentedControl! @IBAction func segmentedControlAddSubtract(sender: AnyObject) { switch segmentedControlCalculate.selectedSegmentIndex { case 0: result = firstInput+ secondInput case 1: result = firstInput - secondInput default: return } updateTotalLabel() } func updateTotalLabel() { total.text = "Total = " + String(result) } @IBOutlet weak var total: UILabel! @IBAction func dismiss(sender: AnyObject) { dismissViewControllerAnimated(true, completion: nil) }