converting text file into xml using php?
10,597
Solution 1
Although I think XMLWriter is best suited for that task (like in my other answer), if you really want to do it with SimpleXML, here's how:
$fp = fopen('data.txt', 'r');
$xml = new SimpleXMLElement('<allproperty></allproperty>');
while ($line = fgetcsv($fp)) {
if (count($line) < 4) continue; // skip lines that aren't full
$node = $xml->addChild('aproperty');
$node->addChild('postcode', $line[0]);
$node->addChild('price', $line[1]);
$node->addChild('imagefilename', $line[2]);
$node->addChild('visits', $line[3]);
}
echo $xml->saveXML();
You will notice that the output is not as clean: it's because SimpleXML doesn't allow you to automatically indent tags.
Solution 2
I will suggest you to use XMLWriter instead, because it is best suited for that (and it's also built-in like SimpleXML):
$fp = fopen('data.txt', 'r');
$xml = new XMLWriter;
$xml->openURI('php://output');
$xml->setIndent(true); // makes output cleaner
$xml->startElement('allproperty');
while ($line = fgetcsv($fp)) {
if (count($line) < 4) continue; // skip lines that aren't full
$xml->startElement('aproperty');
$xml->writeElement('postcode', $line[0]);
$xml->writeElement('price', $line[1]);
$xml->writeElement('imagefilename', $line[2]);
$xml->writeElement('visits', $line[3]);
$xml->endElement();
}
$xml->endElement();
Of course, you can change the php://output
argument to a filename if you want it to output to a file.
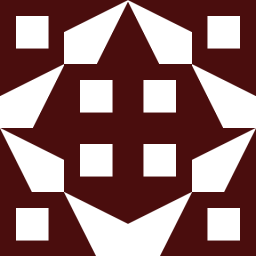
Comments
-
getaway over 1 year
data.txt
ha15rs,250,home2.gif,2 ha36gs,150,home3.gif,1 ha27se,300,home4.gif,4 ha4678,200,home5.gif,5
i want convert this textfile into xml using simplexml module using php? thanks :))
p.s. im new to this
EDIT:
<allproperty> <aproperty> <postcode></postcode> <price></price> <imagefilename></imagefilename> <visits></visits> </aproperty> <aproperty> <postcode></postcode> <price></price> <imagefilename></imagefilename> <visits></visits> </aproperty> <aproperty> <postcode></postcode> <price></price> <imagefilename></imagefilename> <visits></visits> </aproperty> </allproperty>