Copy a list of list by value and not reference
Solution 1
Because python passes lists by reference
This means that when you write "b=a" you're saying that a and b are the same object, and that when you change b you change also a, and viceversa
A way to copy a list by value:
new_list = old_list[:]
If the list contains objects and you want to copy them as well, use generic copy.deepcopy():
import copy
new_list = copy.deepcopy(old_list)
Solution 2
Since Python passes list by reference, A
and B
are the same objects. When you modify B
you are also modifying A
. This behavior can be demonstrated in a simple example:
>>> A = [1, 2, 3]
>>> def change(l):
... b = l
... b.append(4)
...
>>> A
[1, 2, 3]
>>> change(A)
>>> A
[1, 2, 3, 4]
>>>
If you need a copy of A
use slice notation:
B = A[:]
Solution 3
A looks like a reference type, not a value type. Reference types are not copied on assignment (unlike e.g. R). You can use copy.copy to make a deep copy of an element
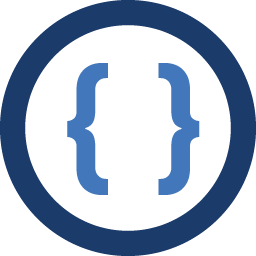
Admin
Updated on July 09, 2022Comments
-
Admin almost 2 years
To understand why I was getting an error in a program , in which I tried to find the "minor" of a determinant, I wrote a simpler program because my variables were messed up. This function below takes in a 2 * 2 matrix as an input, and returns a list containing its rows (pointless and inefficient, I know, but I'm trying to understand the theory behind this).
def alpha(A): #where A will be a 2 * 2 matrix B = A #the only purpose of B is to store the initial value of A, to retrieve it later mylist = [] for i in range(2): for j in range(2): del A[i][j] array.append(A) A = B return mylist
However, here it seems that B is assigned the value of A dynamically, in the sense that I'm not able to store the initial value of A in B to use it later. Why is that?
-
Moses Koledoye over 7 yearsYou'll need to create a
deepcopy
in this case as they have a list of lists. -
iamnicoj about 3 yearsoh boy, i've spent hours figuring out why a recursive algorithm using lists that worked perfectly in javascript was giving me all sort of crazy results in python..... copy.deepcopy almighty
-
g19fanatic almost 3 years
copy.copy
does a shallow copy...copy.deepcopy
does a deepcopy as others have said