Correct syntax to initialize static array
Solution 1
When initializing the way you are you don't need to index the values:
public static string[] PAlphCodes = new string[] {
"1593",
"1604",
"1740",
};
Solution 2
To expand upon what Kennedy answered -- you can also use
public static string[] PAlphCodes = { "1593", "1604", "1740" };
The reference manual has a listing of all the possible ways -- but the one Kennedy suggested -- and this method -- are probably the most common.
https://msdn.microsoft.com/en-us/library/aa287601(v=vs.71).aspx
Solution 3
Indeed you've used strnage syntax to initialize array as pointed out in other answers and something like static string[] PAlphCodes = new []{"1","2","3"};
would fix the problem.
On why this actually compiles (which is somewhat surprising for most people):
You can use static fields to initialize other static fields, but surprisingly you can also refer to static field inside initialization if the field itself. So there is no compile time error.
It fails at run-time first with NullReferenceException
because initialization of the array is not completed by the time it is used for first time - so PAlphCodes
is null
while array is created. But since this is part of class level initialization (as it is static filed) this exception also stops class instance creation and you get "The type initializer ...." wrapping NullReferenceException
.
Note that in most cases such construct would not even compile. I.e. using it in non-static field of local variable fails at compile time with
A field initializer cannot reference the non-static field, method, or property ...
public class PalphabetsDic
{
public string[] PAlphCodes = new string[3] {
PAlphCodes[0] = "1593", // error here and other lines
PAlphCodes[1] = "1604",
PAlphCodes[2] = "1740",
};
}
Related videos on Youtube
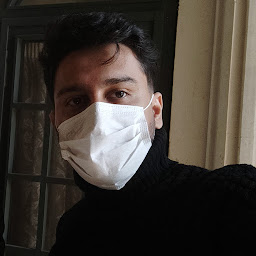
Ali Padida
True knowledge exists in knowing that you know nothing.
Updated on October 28, 2022Comments
-
Ali Padida over 1 year
I have following code definig an array
public class PalphabetsDic { public static string[] PAlphCodes = new string[3] { PAlphCodes[0] = "1593", PAlphCodes[1] = "1604", PAlphCodes[2] = "1740", }; }
When I use this array
var text = PalphabetsDic.PAlphCodes[1]
Gives error:
The type initializer for 'Dota2RTL.PalphabetsDic' threw an exception. ---> System.NullReferenceException: Object reference not set to an instance of an object.
Please can someone help me on this?
Note that What is a NullReferenceException, and how do I fix it? covers arrays, but
PAlphCodes = new string[3]
should be setting it up to be notnull
.-
Preston Guillot over 9 yearsNo, you didn't initialize the array, that's why you have a type initialization exception - the null reference exception you're showing is an inner exception of said type initialization exception. To be fair, I'm somewhat surprised your declaration of
PAlphCodes
even compiles.
-
-
debracey over 9 yearsYou can also use
string[] PAlphCodes = { "1593", "1604", "1740" }
. Both options are valid and commonplace. -
Kennedy Bushnell over 9 yearsTrue. I was trying to change his code as little as possible so he could see what wasn't needed. I should have included both forms though.
-
debracey over 9 yearsGlad to help. Also, welcome to Stack Overflow. Please remember to upvote questions/answers that you find useful, and don't forget to accept the most helpful answer by clicking the check mark.
-
Ali Padida over 9 yearsI still need 15 reputation. xD
-
Ali Padida over 9 yearsThanks a lot for the brief answer, and the modification xD.