Could someone explain me the .getClass() method in java
Solution 1
You first need to know how ==
and !=
compare the two operands. The reason why ==
and !=
cannot be used to compare reference types is that they actually compare the memory addresses of the two reference type variables.
So if I have two strings:
String x = "Hello";
String y = x;
Since x
and y
share the same memory address after the second line is executed, x == y
evaluates to true.
The same goes for the getClass()
method. The getClass()
method returns the class of the object as a Class<T>
object. The question is, why this evaluates to true:
x.getClass() == y.getClass()
The answer is simple. Because x
and y
are both of type String
. So calling getClass
will return the same instance. This means the two returned the objects share the same memory address.
"But when I compare strings with the same characters with the ==
operator, it evaluates to false!" you shouted.
This is because the strings are located at different memory addresses. But the classes that getClass
will return is always at the same memory address if the class that they represent is the same. This is due to the way ClassLoader
works. But I'm not an expert that.
You just need to know that the objects returned by getClass is at the same memory address if the classes they represent are the same.
Solution 2
The comparators ==
and !=
compare equality in the way of identity. So how this works for primitives is obvious. However it can also be used to compare objects. However most often this does not work as expected. There are some exceptions:
String
s are stored as literals, therefore if you define two instances of String
containing the same value, they use the same literal. Think of this like both instances pointing to the same memory location.
Enum
s are basically a collection of constants, therefore an enum value is either the same instance or it is not. It cannot be that the enum has the same value but is another instance.
The same is true for Class
objects, which is what you get when calling getClass()
. A Class
object is created by the ClassLoader
the first time it is the *.class file is loaded. On subsequent calls the same Class
object is used. Therefore Class
objects may be compared with ==
and !=
. However beware that if A.class
is loaded by two different ClassLoader
s, the class objects that you get returned from them are not of the same instance.
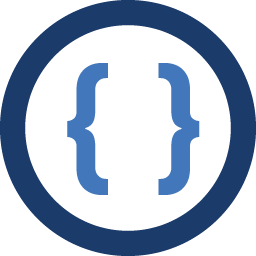
Admin
Updated on July 05, 2022Comments
-
Admin almost 2 years
I am currently taking a java class in university. This is my first programming class and I've stumbled on something that I just cannot understand. As i learned, there are two ways of comparing variables. The first is using the
==
,!=
,<
,>
,=<
,>=
signs for PRIMITIVE variables such as int,double,etc. and the second way is to use the .equals() method for reference type. Now here is my question:When I use the
.getClass()
method, I can compare two classes with the .equals() method and the==
/!=
method. Since I can use the==
/!=
signs, I'd suppose that the.getClass()
method which returns the class of an object must return a primitive type. But searching on google the only thing I found out about this method in the java API is that it returns the class of an object. It doesn't tell me the variable type it returns. How exactly does this method work. What does it return? I tried to ask my teacher but she didn't know. Thank you!