Counting up in ngFor - Angular 2
Solution 1
If you don't want to format your data structure into groups of columns (which I can understand) then you can still render columns 3 per row. For this you will need to precalculate additional helper array of row indexes, e.g. [0,1,2,3]
. Number of the elements in the array should be equal to the number of ion-row
you want to render. So in your controller constructor function you can do something like this:
this.rows = Array.from(Array(Math.ceil(this.movies.length / 3)).keys())
Then in template you will have two ngFor
loops:
<ion-row *ngFor="#i of rows" (click)="movieTapped(m)">
<ion-col *ngFor="#m of movies | slice:(i*3):(i+1)*3" width-33>
<div class="row responsive-md">
<img [src]="m.Poster" width="100%" /> {{m.Title}}
<br> Rating: {{m.imdbRating}}
<br> Rated: {{m.Rated}}
<br> Released: {{m.Year}}
</div>
</ion-col>
</ion-row>
That's it. Depending on the current row index slice pipe renders necessary items per row.
Here is simplified demo: http://plnkr.co/edit/WyEfryGccFrJvwm6jExM?p=preview
Solution 2
You don't have to do any change in the data! Ionic 2 already providing the support for it.
We can use the wrap
in <ion-row>
. Example: <ion-row wrap>
Use the wrap attribute on an
<ion-row>
element to allow items in that row to wrap. This applies theflex-wrap: wrap;
style to the<ion-row>
element.
Solution 3
I would modify the data to represent the structure you want to render (rows of 3 columns) and then render the view according to the data.
<ion-row *ngFor="let r of rows; let i = index" (click)="movieTapped(m)">
<ion-col width-33 *ngFor="let m of r; let j = index">
<div class="row responsive-md">
<img [src]="m.Poster" width="100%" />
{{m.Title}} <br>
Rating: {{m.imdbRating}}<br>
Rated: {{m.Rated}}<br>
Released: {{m.Year}}<br>
</div>
</ion-col>
</ion-row>
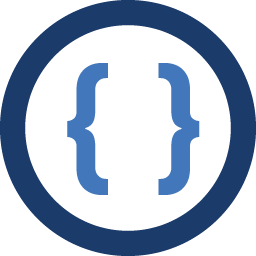
Admin
Updated on June 13, 2022Comments
-
Admin almost 2 years
I have a problem counting up the ngFor Loop in Angular 2. Im trying to develop a grid that has 3 columns and a dynamic amount of rows. I would like to loop through my array and add the elements from left to right and every three elements it should jump to the next row. Im using Ionic Framework 2.0.
My code looks like this:
<ion-row *ngFor="#m of movies; #i = index" (click)="movieTapped(m)"> <ion-col width-33> <div class="row responsive-md"> <img [src]="m.Poster" width="100%" /> {{m.Title}} <br> Rating: {{m.imdbRating}}<br> Rated: {{m.Rated}}<br> Released: {{m.Year}}<br> </div> </ion-col> <ion-col width-33> <div class="row responsive-md"> <img [src]="m.Poster" width="100%" /> </div> </ion-col> <ion-col width-33> <div class="row responsive-md"> <img [src]="m.Poster" width="100%" /> </div> </ion-col> </ion-row>
Hope you can help me out.
Cheers!
-
Admin about 8 yearsthank you so much! this is exactly what I was looking for
-
Maarten Heideman over 7 yearsNice end simple solution didn't know
-
bones.felipe almost 7 yearsThis is exactly what I needed. Good stuff.
-
Mohammed A. Al Jama about 4 yearsfor those with VueJS,
<ion-row v-for="r in rows" v-bind:key="r"> <ion-col v-for="item in items.slice((r*3), (r+1)*3)" v-bind:key="item.id"