Crash on Android 10 (InflateException in layout/abc_screen_simple line #17)
Solution 1
Update Calligraphy
to newest version to solve this problem:
Link: https://github.com/InflationX/Calligraphy/issues/35
More specifically, both Calligraphy and ViewPump need to be updated:
implementation 'io.github.inflationx:calligraphy3:3.1.1'
implementation 'io.github.inflationx:viewpump:2.0.3'
Migrating from Calligraphy 2 to 3 requires some code changes; see examples in Calligraphy 3 README.
Solution 2
You need to update calligraphy version and change code according to new version
You need to change repository in dependencies from
implementation "uk.co.chrisjenx:calligraphy:$caligraphyVersion"
to
implementation 'io.github.inflationx:calligraphy3:3.1.1'
implementation 'io.github.inflationx:viewpump:2.0.3'
You need to change usage of import from
import uk.co.chrisjenx.calligraphy.CalligraphyConfig;
to
import io.github.inflationx.calligraphy3.CalligraphyConfig;
Calligraphy config from
CalligraphyConfig.initDefault(new CalligraphyConfig.Builder()
.setDefaultFontPath(getResources().getString(R.string.bariol))
.setFontAttrId(R.attr.fontPath)
.build()))
.build());
to
ViewPump.init(ViewPump.builder()
.addInterceptor(new CalligraphyInterceptor(
new CalligraphyConfig.Builder()
.setDefaultFontPath(getResources().getString(R.string.bariol))
.setFontAttrId(R.attr.fontPath)
.build()))
.build());
I used font bariol you can change it to yours.
& newbase to
super.attachBaseContext(ViewPumpContextWrapper.wrap(newBase));
Solution 3
Please foloow this below steps.
1> First of all check build.gradle(:app) file
2> If you are using this below library:
implementation 'uk.co.chrisjenx:calligraphy:2.3.0'
3> Update this " implementation 'uk.co.chrisjenx:calligraphy:2.3.0' " library with this below library.
implementation 'io.github.inflationx:viewpump:2.0.3'
implementation 'io.github.inflationx:calligraphy3:3.1.1'
4> In project where You use this below code :
@Override
protected void attachBaseContext(Context newBase) {
super.attachBaseContext(CalligraphyContextWrapper.wrap(newBase));
}
5> Update it with this below code:
@Override
protected void attachBaseContext(Context newBase) {
super.attachBaseContext(ViewPumpContextWrapper.wrap(newBase));
}
Solution 4
Definitively your problem is the Calligraphy library, I've got the same problem and there are 2 ways to solve it:
- Go back to target version 28, and wait a possible update of the Calligraphy library. 2
- Remove the library
About the exception:
The exception error in Calligraphy comes from the use of the reflection in the library. See the last line of this exception:
E/AndroidRuntime: FATAL EXCEPTION: main
Process: com.example, PID: 8220
android.view.InflateException: Binary XML file line #17 in com.example:layout/abc_screen_simple: Binary XML file line #17 in com.example/abc_screen_simple: Error inflating class androidx.appcompat.widget.FitWindowsLinearLayout
Caused by: android.view.InflateException: Binary XML file line #17 in com.example/abc_screen_simple: Error inflating class androidx.appcompat.widget.FitWindowsLinearLayout
Caused by: java.lang.NullPointerException: Attempt to invoke virtual method 'java.lang.Object java.lang.reflect.Field.get(java.lang.Object)' on a null object reference
Android in your documentation explain that some reflection methods (non-SDK interfaces) are restricted in the API 29 platform.
Reflection via Class.getDeclaredField() or Class.getField() --------> NoSuchFieldException thrown
Reflection via Class.getDeclaredMethod(), Class.getMethod() ----> NoSuchMethodException thrown.
Reflection via Class.getDeclaredFields(), Class.getFields() --------> Non-SDK members not in results.
Reflection via Class.getDeclaredMethods(), Class.getMethods() -> Non-SDK members not in results
Source: Restrictions on non-SDK interfaces
Solution 5
if you are using calligraphy so you should migrate to calligraphy3 : https://github.com/InflationX/Calligraphy
implementation 'io.github.inflationx:calligraphy3:3.1.1'
implementation 'io.github.inflationx:viewpump:2.0.3'
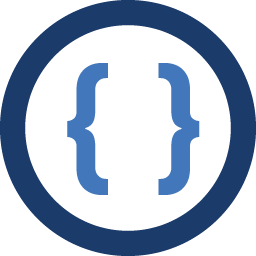
Admin
Updated on August 13, 2021Comments
-
Admin almost 3 years
My application works fine from Android 4.3 until Android 9 Pie, but my application doesn't work on Android 10 (Q API 29) and crashes. This is my logcat - why this is happening?
java.lang.RuntimeException: Unable to start activity ComponentInfo{ir.mahdi.circulars/ir.mahdi.circulars.MainActivity}: android.view.InflateException: Binary XML file line #17 in ir.mahdi.circulars:layout/abc_screen_simple: Binary XML file line #17 in ir.mahdi.circulars:layout/abc_screen_simple: Error inflating class androidx.appcompat.widget.FitWindowsLinearLayout
and this is my mainActivity.xml
<?xml version="1.0" encoding="utf-8"?> <androidx.coordinatorlayout.widget.CoordinatorLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" android:background="@color/white" android:layoutDirection="ltr" tools:context=".MainActivity"> </androidx.coordinatorlayout.widget.CoordinatorLayout>
update
apply plugin: 'com.android.application' android { compileSdkVersion 29 defaultConfig { minSdkVersion 16 targetSdkVersion 29 multiDexEnabled true testInstrumentationRunner "androidx.test.runner.AndroidJUnitRunner" } buildTypes { release { minifyEnabled false proguardFiles getDefaultProguardFile('proguard-android-optimize.txt'), 'proguard-rules.pro' } } } dependencies { implementation fileTree(dir: 'libs', include: ['*.jar']) implementation 'androidx.appcompat:appcompat:1.0.0' implementation 'com.google.android.material:material:1.0.0' implementation 'androidx.constraintlayout:constraintlayout:1.1.3' testImplementation 'junit:junit:4.12' androidTestImplementation 'androidx.test:runner:1.2.0' androidTestImplementation 'androidx.test.espresso:espresso-core:3.2.0' }
-
Konstantin Kuznetsov almost 5 yearsThis is the correct answer. implementation 'io.github.inflationx:calligraphy3:3.1.1' implementation 'io.github.inflationx:viewpump:2.0.3'
-
Jonik over 4 yearsThis is a workaround, but at some point you'll want to update
targetSdk
to 29 in any case, and you'll need to properly deal with the issue. -
Jonik over 4 years@KonstantinKuznetsov is right. The problem in our case: the Calligraphy library has changed quite a bit between 2.2.0 and 3.1.1, and it's nontrivial to update your application code. For example,
CalligraphyContextWrapper
no longer exists in 3.1.1. -
Jonik over 4 yearsUpdate: actually it is quite trivial to update to Calligraphy 3; the code snippets in the README make it straightforward. But thing is, at least in our case the Calligraphy update didn't help: still getting InflateException with
layout/abc_screen_simple
after that. -
Konstantin Kuznetsov over 4 years@Jonik hmm.. very strange. Maybe some standalone modules in your app still have old dependencies to Calligraphy? Did you try to check transitive dependencies in gradle, maybe you'll see something strange?
-
Jonik over 4 years@KonstantinKuznetsov: Thanks a lot! I checked
:app:dependencies
and saw that calligraphy3:3.1.1 was depending on viewpump:1.0.0. That was because in our build files we only had explicit dependency on Calligraphy. Adding viewpump:2.0.3 explicitly fixes this! -
Jonik over 4 yearsI took the liberty to edit the missing info into the answer.
-
Jonik over 4 yearsIn all likelihood, Calligraphy dependency has been added in a project for a reason. No need to remove it; updating to Calligraphy 3 also fixes the InflateException issue: stackoverflow.com/a/57462616/56285
-
Aleksandar Mironov over 4 yearsOnly targetSdk must be 28, compileSdk and buildTools can be 29, but this is temporarily solution
-
Gokul Rajkumar about 4 yearsThis solution was explained properly and it solved the issue
-
Viet Nguyen Trong almost 4 yearsThat saves my life.
-
Sandeep Singh over 3 yearsIt works like charm, I am thankful for this answer.
-
Shashi Shiva L over 2 yearsThanks @Nafees Khabir very clear solution.