Create a simple JAR instead of an executable JAR with Spring-Boot
Solution 1
It seem like you can disable the fat-JAR from replacing the original and getting installed into the repo by configuring the spring-boot-maven-plugin
.
Taken from http://docs.spring.io/spring-boot/docs/1.5.1.RELEASE/maven-plugin/examples/repackage-disable-attach.html:
By default, the repackage goal will replace the original artifact with the executable one. If you need to only deploy the original jar and yet be able to run your app with the regular file name, configure the plugin as follows:
<project>
...
<build>
...
<plugins>
...
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
<version>1.5.1.RELEASE</version>
<executions>
<execution>
<goals>
<goal>repackage</goal>
</goals>
<configuration>
<attach>false</attach>
</configuration>
</execution>
</executions>
...
</plugin>
...
</plugins>
...
</build>
...
</project>
This configuration will generate two artifacts: the original one and the executable counter part produced by the repackage goal. Only the original one will be installed/deployed.
Solution 2
You can just disable the spring-boot-maven plugin this way.
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
<executions>
<execution>
<configuration>
<skip>true</skip>
</configuration>
</execution>
</executions>
</plugin>
Solution 3
To create simple jar update
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
</plugin>
To
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
<configuration>
<classifier>exec</classifier>
</configuration>
</plugin>
For more details please visit below Spring URL:- https://docs.spring.io/spring-boot/docs/1.1.2.RELEASE/reference/html/howto-build.html
Solution 4
I have found the answer. I configured my application just like @judgingnotjudging explained. The difference was that I had put this, in the parent-POM:
<build>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
</plugin>
</plugins>
</build>
It was preventing the default creation of JARs in the children-modules. I could resolve this problem by including this only in the web-Module.
That way Spring-Boot builds a fat-JAR from the web-Module and a simple JAR from the core-Module.
Solution 5
You can simply change
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
to
<artifactId>maven-compiler-plugin</artifactId>
<version>3.8.0</version>
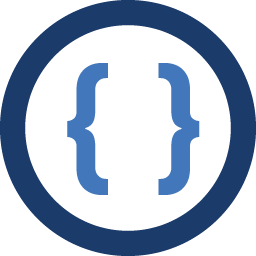
Admin
Updated on June 09, 2022Comments
-
Admin almost 2 years
I have a problem with a Multi-Module Spring-Boot Application.
I have one Module that I use for Services, the core-Module. And one module for View-Related Classes, the web-Module. The are both in a parent-Module, where I add the dependencies, like the "spring-boot-starter" and that can be used by both modules.
Now to the problem:
I want to run the web-Module with the embedded Tomcat and have the core-Module as a dependency in the web-module.
In other Spring projects I would just include the maven-jar-plugin and create a jar of the core-Module.
The problem in this Spring-Boot project is that the maven-jar-plugin is already configured, in the "spring-boot-starter". And it needs a mainClass, which only the web-module has.
Small excerpt from the "spring-boot-starter"-POM
<plugin> <groupId>org.apache.maven.plugins</groupId> <artifactId>maven-jar-plugin</artifactId> <configuration> <archive> <manifest> <mainClass>${start-class}</mainClass> <addDefaultImplementationEntries>true</addDefaultImplementationEntries> </manifest> </archive> </configuration> </plugin>
Is there a way to package the core-Module as a JAR without needing a "start-class" in that module?
-
Admin almost 8 yearsI want to use the web-module to be the fat-JAR and have the core-module as a dependency. To have the core-module as a dependency, I need to be able to create a "product" that can be installed in the .m2-directory. Maybe there's an easier way of achieving this.
-
JudgingNotJudging almost 8 years@Johannes Esseling I updated my answer to include building a multi-module project. It sounds like you're expecting to publish your core-module to the repository then retrieve it. You can avoid that by creating the project as a multi-module project.
-
Renato Back about 6 yearsPerfect solution.
-
Matthew over 3 yearsNot including the magic maven plugin seems to be a simple solution that worked for me.
-
coretechie almost 2 yearsI removed the entire plugin and it worked for me too. I ran mvn package command.