Create Button Element in JavaScript
17,848
Your script is running before the DOM has finished loading, so before your 'btn' div exists. There are a few simple solutions to this. One is moving your script tag to the bottom of the body, eg:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<title>Document</title>
</head>
<body>
<div id="btn">Button Here</div>
<script src="myapp.js"></script>
</body>
</html>
The other, better, solution is adding a line to your script to make sure the HTML has loaded before the script runs.
document.addEventListener("DOMContentLoaded", function() {
var element = document.createElement("button");
element.appendChild(document.createTextNode("Click Me!"));
var page = document.getElementById("btn");
page.appendChild(element);
console.log(element);
});
// Corrected "meta charset="UTF-8"".
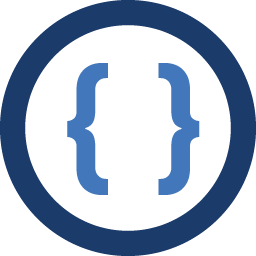
Author by
Admin
Updated on June 04, 2022Comments
-
Admin about 2 years
I'm trying to create a button element in
javascript
without usingjQuery
.I keep getting an error when I try to append it to the DOM.
"Cannot read property 'appendChild' of null"
I've looked everywhere and couldn't find out how to create a button. In the w3Schools example they are using a function on a button element that was already created in the HTML, which isn't what I'm trying to do. Here's what I have.
HTML:
<!DOCTYPE html> <html lang="en"> <head> meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <meta http-equiv="X-UA-Compatible" content="ie=edge"> <title>Document</title> <script src="myapp.js"></script> </head> <body> <div id="btn">Button Here</div> </body> </html>
myapp.js
var element = document.createElement("button"); element.appendChild(document.createTextNode("Click Me!")); var page = document.getElementById("btn"); page.appendChild(element); console.log(element);