Create directory in C++
Solution 1
The C++11 standard n3337 does not know about directories. C++17 has <filesystem>
standard header.
If your implementation is C++17 compliant (it probably is not), use std::filesystem::create_directory
Otherwise, use operating system primitives. Be aware that the notion of directory can be OS specific (i.e. different in one OS from another, perhaps even between different file systems). For Windows, study the WinAPI (so consider CreateDirectoryA). For Linux, look into its syscalls(2) (so consider mkdir(2))
Some frameworks, notably Qt, POCO, Boost, provide common wrappers above them.
Solution 2
You can use a simple mkdir from <direct.h>
to create directory
_mkdir("C:\\Data\\FolderName");
Solution 3
You can use Visual Studio's <filesystem>
There is a function create_directory
that has the signature
template<class Path>
inline bool create_directory(
const Path& Pval
);
You can find the user's Documents directory using SHGetKnownFolderPath
which has the signature
HRESULT SHGetKnownFolderPath(
_In_ REFKNOWNFOLDERID rfid,
_In_ DWORD dwFlags,
_In_opt_ HANDLE hToken,
_Out_ PWSTR *ppszPath
);
In this case the REFKNOWNFOLDERID
you want to use is FOLDERID_Documents
Note this is specific to Visual Studio. The C++ <filesystem>
library is still in the works (i.e. experimental) for now, but hopefully is coming soon!
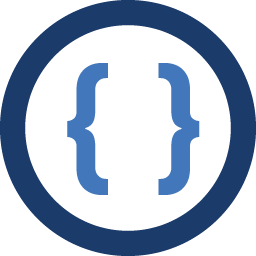
Admin
Updated on December 02, 2020Comments
-
Admin over 3 years
I'm making a simple game in DirectX and C++. But I want to create a directory in Documents for the settings etc.
But I don't know how I need to do this?
Can someone help me?