Creating a class member pointer function variable that points to a non-static class member function
Solution 1
&CreateAnotherValue
This syntax is not valid. To create a pointer-to-member, you have to name the class, even from inside other members. Try
&Foo::CreateAnotherValue
In this case you are talking the address of a qualified non-static member function, which is allowed and prevents the error about address of unqualified member function.
Of course, you then need an appropriately typed variable to store the pointer-to-member in, see Bo's answer for the correct declaration. When it comes time to call it, you will need to use the pointer-to-member-dereference operator (either .*
or ->*
), so say
(this->*_AddValue)(whatever);
The same rule applies to data, if you say &Foo::_value
, you get a pointer-to-member of type int Foo::*
. But in the data case, the unqualified name is also accepted, but with very different behavior. &_value
gives a normal pointer, type int*
, which is the address of the specific _value
member variable inside the this
instance.
Solution 2
void (*_AddValue)(int value); // Pointer to function member variable
This is not really a pointer-to-member, but a pointer to a free function.
You need to make this
void (Foo::*_AddValue)(int value); // Pointer to function member variable
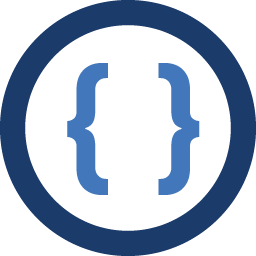
Admin
Updated on July 02, 2022Comments
-
Admin almost 2 years
The goal is to have the member variable
_AddValue
point to theCreateFirstValue
function upon class initialization and after the first invocation ofAddValue
, all future calls to it will invokeCreateAnotherValue
.Previously, I just had a single
AddValue
function with a conditional check to determine which function to call. However, I feel like that implementation is flawed because thatif
check will occur every time and it seems like a function pointer would be beneficial here.An example:
class Foo { private: int _value; void (*_AddValue)(int value); // Pointer to function member variable void CreateFirstValue(int value) { _value = value; _AddValue = &CreateAnotherValue; } void CreateAnotherValue(int value) { // This function will create values differently. _value = ...; } public: // Constructor Foo() : _value(0), _AddValue(CreateFirstValue) { } AddValue(int value) // This function is called by the user. { _AddValue(value); } };
The code above is not the actual code, just an example of what I'm trying to accomplish.
right now I'm getting an error:
argument of type void (BTree::)(int) does not match void (*)(int)
-
Admin almost 12 years@BoPersson With that change, I'm getting "btree.cpp: In constructor BTree::BTree():" "btree.cpp:9:21: error: argument of type void (BTree::)(int) does not match void (BTree::*)(int)"
-
Bo Persson almost 12 years@fhaddad - You might need to use
&Foo::CreateAnotherValue
to take the address of the member function. That would match theFoo::*
of the pointer. -
Admin almost 12 yearsThank you for the excellent explanation. I knew I had the general gist of all this right, just implementation was weird for me. Especially because our text book doesn't go into much depth with regards to function pointers. With your explanation and Bo's example, it's not compiling. Thank you!
-
Admin almost 12 yearswith your help and Ben's it's not compiling. I knew it was syntax issues. I'm still quite confused on how one understands how all this stuff works. Lots of time when I am reading the compiler errors, I don't understand what they are saying.
-
Admin almost 12 yearsComment above should say ...it's now compiling... (=
-
Admin almost 12 yearsComment above should say ...it's now compiling... (=