Creating an HTML dialog pop-up in chrome extension
Solution 1
You should be able to use the javascript for the answer you linked to open that dialog whenever you want by injecting your javascript via a content script. Google has documentation for this here: https://developer.chrome.com/extensions/content_scripts
Basically, a content script runs on whatever pages you tell it to. So you could tell it to run on all web pages (configured via the manifest). This content script would add your listener, and then append your dialog to the body tage (or wherever, body is usually safe).
The code would look something like:
$(document).on( 'keypress', 'body', function( e ){
var code = e.keyCode || e.which;
if( code = /*whatever, key shortcut listener*/ ) {
document.body.innerHTML += '<dialog>This is a dialog.<br><button>Close</button></dialog>';
var dialog = document.querySelector("dialog")
dialog.querySelector("button").addEventListener("click", function() {
dialog.close()
})
dialog.showModal()
}
});
You may want to add safety code to check for your body tag; it's on normal pages but specialty pages may error (such as chrome://*).
As long as this runs on your content script, and your content script runs on your desired pages, you can run whatever listeners/dom changers you want this way.
Solution 2
You don't need to add a permission for a public api. You only need to add a permission if the site doesn't allow cross origin requests.
Also, adding the listener for your keyboard shortcut using a web listener through a content script is not a good solution, since it requires a permission warning, and is generally not efficient. Instead, you should use Chrome's commands api with activetab. Your extension will only be started when the user hits the keyboard shortcut, and the user can customize the shortcut via the shortcuts link at the bottom of chrome://extensions.
To do this, add "permissions": [ "activeTab" ]
to your manifest. Then add your key combo:
"commands": { "showcontentdialog": { "suggested_key": { "default": "Ctrl+Shift+Y" }, "description": "show content dialog" } }
Next, setup your background page listener and inject your content script when the user hits those keys:
chrome.commands.onCommand.addListener(function(command) {
if(command.name == "showcontentdialog") {
chrome.tabs.executeScript({ file: "main.js" })
}
})
Also, you should use appendChild instead of setting innerHTML, like this:
var dialog = document.createElement("dialog")
dialog.textContent = "This is a dialog"
var button = document.createElement("button")
button.textContent = "Close"
dialog.appendChild(button)
button.addEventListener("click", function() {
dialog.close()
})
document.body.appendChild(dialog)
dialog.showModal()
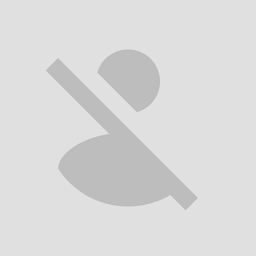
Jonathan Cox
Updated on June 14, 2022Comments
-
Jonathan Cox almost 2 years
I'm working on a Chrome extension that will pop up a dialog box in the center of the screen when the user hits a keyboard shortcut. It will then use JavaScript to asynchronously load in content from the MediaWiki API. But I'm having difficulty figuring out how to create and display a dialog with JavaScript. I don't want to use the Chrome
html-popup
browser action, because it appears off in the corner of the screen.I know how to use JavaScript to display an existing HTML dialog box, as this answer explains, but I don't know how to insert one into the DOM. I don't want to use JavaScript's
alert
function, since that opens a separate window. So is there a way to create and display an HTML modal dialog when an event triggers a JavaScript function in a chrome extension?-
Daniel Herr over 8 yearsDoes your extension require site access excluding injecting the dialog?
-
Jonathan Cox over 8 yearsI don't need access to the site the user is currently on, I just want to get the currently highlighted text using
getSelection().toString()
. I do, however, need to make a cross-domain request to the MediaWiki API. As I understand it, I can declare a permission in the chrome extension manifest to allow cross-site domain requests, though it isn't usually allowed with JS. Is that right?
-
-
Jonathan Cox over 8 yearsThanks for the answer. Some follow-up questions: What does the second parameter in the
$(document).on()
function, ('body'), do? Is it okay to remove it? Also, isn't thewhich
in line 2 only necessary for Firefox compatibility? Chrome should work fine with just keyCode, right? -
Brian over 8 yearsI didn't really focus on the event handler, from your question I assumed you had that part figured out and was just demonstrating how you'd add a dialog to a body, and used the handler as an example. The handler I have there is just listening for a keypress on the body. You'd have to write whatever the specific event you want to listen for would be. And yes, keyCode should work in Chrome.
-
Dudi Boy almost 6 yearsThe benefit of using the activeTab api, is the ability to customize the shortcut key. The liability is added JavaScript source file, and lots of source code to transfer context data between the content_script and the background script.