creating hyperlinks in python
Solution 1
You seem to be a bit confused about what a hyperlink, well, is.
A text file is a file containing text. (It's simple, but it needs to be said!) It doesn't have pictures, animations, colours, headers, or anything like that. It's just text.
Since people often want more data with their text (x should be a heading, y should be red, z should make a funny cursor when you mouse over it), there are many schemes for encoding data about text. For example, Markdown is a text format used by StackOverflow. HTML is a markup language (a way of annotating text) that uses <tag>
elements. It's useful because web browsers can take HTML pages as input and display them graphically.
A hyperlink as you describe it is a graphical element such as you might find on a website. You can't have them in a text file, because a text file is just text. But you can instruct a web browser to display a hyperlink by writing
<a href="where/you/want/the/link/to/go">text of the link</a>
If you open a file containing that in a web browser, it will display the text as a link. Note that files containing HTML are conventionally called something.html
to indicate their contents, and that there are a bunch of required tags in any HTML document (<html><head></head><body></body></html>
).
Solution 2
Creating hyperlinks in Python?
This is fairly trivial, as a hyperlink is of the format:
hyperlink_format = '<a href="{link}">{text}</a>'
One can parameterize this easily in Python. Here's several methods for doing so:
Call the .format method from the string
>>> hyperlink_format.format(link='http://foo/bar', text='linky text')
'<a href="http://foo/bar">linky text</a>'
Use a bound .format
object:
link_text = hyperlink_format.format
Usage:
>>> link_text(link='http://foo/bar', text='foo bar')
'<a href="http://foo/bar">foo bar</a>'
Make a partial function
import functools
link_text = functools.partial(hyperlink_format.format)
Usage:
>>> link_text(link='http://foo/bar', text='linky text')
'<a href="http://foo/bar">linky text</a>'
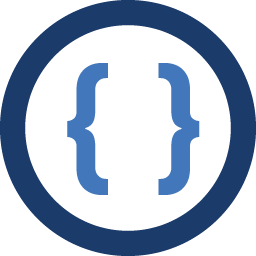
Admin
Updated on February 20, 2020Comments
-
Admin over 4 years
I have a log file in which some test commands and their status (Pass/Fail) are logged using python. Now I want that test commands should not be written as simple text but should be written as hyperlinks. So that when I click on them another files which are linked to them are opened.
For e.g:
file = open("C:/logfile.log", "w") file.write("[Command Name - '%35s'] [PASSED]\n" %(CommandName)) file.close() file1 = open("C:/TestCommand.log/", "w") file1.write("'%35s \n" %(str(parameter_val_for_test_command))) file1.close()
Now I want that the CommandName written in logfile.log should be a hyperlink for the file TestCommand.log So that when I click on the CommandName the file TestCommand.log opens.
Can you please suggest how should I do create a logfile.log with hyperlinked CommandName and then how should I link this hyperlinked CommandName to the file TestCommand.log??