(CSS) Make a background image scroll slower than everything else
Solution 1
I stumbled upon this looking for more flexibility in my parallax speed that I have created with pure CSS and I just want to point out that all these people are wrong and it is possible with pure CSS It is also possible to control the height of your element better.
You will probably have to edit your DOM/HTML a bit to have some container elements, in your case you are applying the background to the body which will restrict you a lot and doesn't seem like a good idea.
http://keithclark.co.uk/articles/pure-css-parallax-websites/
Here is how you control the height with Viewport-percentage lenghts based on screen size:
https://stanhub.com/how-to-make-div-element-100-height-of-browser-window-using-css-only/
.forefront-element {
-webkit-transform: translateZ(999px) scale(.7);
transform: translateZ(999px) scale(.7);
z-index: 1;
}
.base-element {
-webkit-transform: translateZ(0);
transform: translateZ(0);
z-index: 4;
}
.background-element {
-webkit-transform: translateZ(-999px) scale(2);
transform: translateZ(-999px) scale(2);
z-index: 3;
}
Layer speed is controlled by a combination of the perspective and the Z translation values. Elements with negative Z values will scroll slower than those with a positive value. The further the value is from 0 the more pronounced the parallax effect (i.e. translateZ(-10px) will scroll slower than translateZ(-1px)).
Here is a demo I found with a google search because I know there are a lot of non-believers out there, never say impossible:
http://keithclark.co.uk/articles/pure-css-parallax-websites/demo3/
Solution 2
I know this is very late, but I wondered wether it would not be working easier than the codes above and invested 15 mins of my live.
Here is my code:
document.getElementById("body").onscroll = function myFunction() {
var scrolltotop = document.scrollingElement.scrollTop;
var target = document.getElementById("main1");
var xvalue = "center";
var factor = 0.5;
var yvalue = scrolltotop * factor;
target.style.backgroundPosition = xvalue + " " + yvalue + "px";
}
#main1 {
background-image: url(https://images.unsplash.com/photo-1506104489822-562ca25152fe?ixlib=rb-1.2.1&ixid=eyJhcHBfaWQiOjEyMDd9);
background-position: top center;
background-attachment:scroll;
background-size: cover;
height: 80vh;
width: 100%;
}
#placeholdersoyoucanscroll{
height:100vh
}
<body id="body">
<main>
<div id="main1">
</div>
<div id="placeholdersoyoucanscroll">
</div>
</main>
</body>
Solution 3
you can use something simple like here: html:
Motion
css:
body {
min-height:4000px;
background-image: url("http://www.socialgalleryplugin.com/wp-content/uploads/2012/12/social-gallery-example-source-unknown-025.jpg");
}
h1 {margin-top:300px;}
js:
(function(){
var parallax = document.querySelectorAll("body"),
speed = 0.5;
window.onscroll = function(){
[].slice.call(parallax).forEach(function(el,i){
var windowYOffset = window.pageYOffset,
elBackgrounPos = "50% " + (windowYOffset * speed) + "px";
el.style.backgroundPosition = elBackgrounPos;
});
};
})();
Here is jsfiddle
Solution 4
If you want to apply a high background image, use this JS:
(function () {
var body = document.body,
e = document.documentElement,
scrollPercent;
$(window).unbind("scroll").scroll(function () {
scrollPercent = 100 * $(window).scrollTop() / ($(document).height() - $(window).height());
body.style.backgroundPosition = "0px " + scrollPercent + "%";
});
})();
Solution 5
I also was looking for a modern and intuitive approach to adjusting my background scroll speed. You should try out the Simple Parallax Scrolling jQuery Plug-in, inspired by Spotify.com.
Once you have it attached to your project, along with a big image to play with, this is one of many ways to set it up.
The HTML | setup your containers and basic parallax attributes on element.
<main>
<section>Some Content Above it</section>
<div class="parallax-container" data-parallax="scroll" data-position="top" style="height: 80vh;"></div>
<section>Some Content Below it</section>
</main>
The jQuery | Here, you can play with additional parameters based on the docs
function parallaxjs() {
$('.parallax-container').parallax({
imageSrc: '/<path-to-your-image>/<your-big-image>.jpg',
naturalWidth: 1071, //your image width value
naturalHeight: 500, //your image height value
bleed: 0,
});
}
(function () {
parallaxjs();
})($);
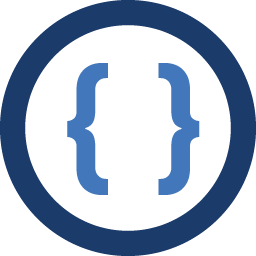
Admin
Updated on February 05, 2020Comments
-
Admin about 4 years
here is is my CSS code for the body:
body { padding: 0; margin: 0; background-image: url("../images/background.jpg"); background-repeat: no-repeat; background-color: grey; background-size: 100%; }
What I want to do is make it so that the image scrolls slower than everything else on the page to make a simple parallax effect. I've looked online and all of the examples I've seen are much more complicated than what I want.
-
ivarni over 7 yearsNot true, it's been possible with CSS for years. See here
-
ivarni over 7 yearsNot true, it's been possible with CSS for years. See here
-
CMG over 7 yearsI wanted to clarify that this is still not a good solution if you're concerned about browser compatibility. On latest Firefox/Chrome of the time of this writing, there's is a white bar that appears to the left of each container. Also, on Chrome, the background elements appear very fuzzy. While the effect occurs, the visual appearance is still lacking in comparison to JS solutions, and therefore this pure CSS solution does not work.
-
Tharaka Nuwan over 6 yearsAlso this altering the scroll effect of the mobile devices. i:e iPad and iPhone won't continue scrolling after you release the touch which feels sticky. However unlike Jquery parallax techniques, this works in all browsers including safari.
-
Eolis over 6 yearsthis is quite simple and works nicely. also unlikepure css solutions it isnt blurry and terrible looking. +1
-
Bakabaka about 5 yearsWould there be a possibility to leave the background's horizontal placement intact? I'm using this for a hero image that I need to nudge around with
background-position
depending on the screen size. Otherwise, great solution! -
klewis about 4 yearsI would upvote this answer if this included a raw javascript approach that "did not require" attaching jquery to the project.
-
jean d'arme over 3 yearsScrolling issue is still there - any option to get rid of this "stickiness"?
-
Codey over 3 yearsWorks perfect and is so much simpler than dealing with transforms.
-
Martins almost 3 yearsPerfect answer! Works like a charm. If you want to change the background image scrolling direction, replace this line
target.style.backgroundPosition = xvalue + " " + yvalue + "px";
with this codetarget.style.backgroundPosition = xvalue + " -" + yvalue + "px";
-
BarryCap almost 3 years@CMG I don’t think the white bar is due to a compatibility issue; I didn’t understand what you where talking about when you say that ‘the background elements appear very fuzzy’, because it’s not the case here; and as for browser compatibility, IE11 doesn’t support this method (but who is still using IE nowadays?), but browser compatibility for CSS 3D transforms is—I think—good enough. Browser compatibility wasn’t a bother in the question, but a CSS solution was asked.
-
Shahriar over 2 yearsThanks Tim! Changing
background-attachment: scroll
tobackground-attachment: fixed
, fixes a small lag and makes it even better.