CupertinoPicker textStyle flutter
Solution 1
Its possible to style CupertinoPicker
and CupertinoDatePicker
using theme like this:
return MaterialApp(
theme: ThemeData(
cupertinoOverrideTheme: CupertinoThemeData(
textTheme: CupertinoTextThemeData(
dateTimePickerTextStyle: TextStyle(color: Colors.blue, fontSize: 16),
pickerTextStyle: TextStyle(color: Colors.blue, fontSize: 12),
)
)
)
)
Solution 2
Wrap the CupertinoPicker() with another widget known as CupertinoTheme() as
CupertinoTheme(
data: CupertinoThemeData(
textTheme: CupertinoTextThemeData(
pickerTextStyle: TextStyle(//Your normal TextStyling)
),
),
child:CupertinoPicker(//Your Cupertino Picker)
)
Solution 3
I know this solution is verbose but it works:
final cupertinoTheme = CupertinoTheme.of(context);
// Do your customising here:
final pickerTextStyle =
cupertinoTheme.textTheme.pickerTextStyle.copyWith(fontSize: 18.0, color: Colors.cyan);
final textTheme =
cupertinoTheme.textTheme.copyWith(pickerTextStyle: pickerTextStyle);
return CupertinoTheme(
data: cupertinoTheme.copyWith(textTheme: textTheme),
child: yourPickerGoesHere,
);
CupertinoPicker
takes text style from CupertinoTheme.of(context).textTheme.pickerTextStyle
. All we do here is to update pickerTextStyle
with our settings.
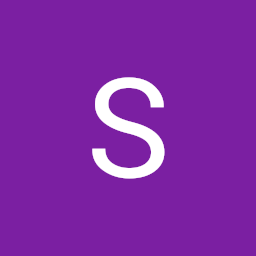
Simone
Updated on December 02, 2022Comments
-
Simone over 1 year
I'm new to flutter and I need help.
I'm creating an app where the user can select data through a CupertinoPicker.
The picker works fine, but I would like to change its style.
Currently the style is like that, but I want it to be like that.
Unfortunately I can't understand how to do it, I read this but I can't do it, I would like to change the color and size of the selected element, the color of the elements not selected and the color of the lines.
But I do not know how I can do it.
Can anyone help me understand please?
The code is this :
Container( ˙child: _showCupertinoPicker( context, book[currentPage].orari.map((orario) { return Center( child: Text(orario, style: TextStyle(color: CupertinoColors.activeBlue ))); }).toList())), . . . . _showCupertinoPicker(BuildContext context, List<Widget> orariWidget) { return CupertinoPicker( backgroundColor: Colors.white, onSelectedItemChanged: (value) {}, itemExtent: 40.0, children: orariWidget, ); }