Currency Conversion using PHP
Solution 1
After searching a lot, found this.
// Fetching JSON
$req_url = 'https://api.exchangerate-api.com/v4/latest/USD';
$response_json = file_get_contents($req_url);
// Continuing if we got a result
if(false !== $response_json) {
// Try/catch for json_decode operation
try {
// Decoding
$response_object = json_decode($response_json);
// YOUR APPLICATION CODE HERE, e.g.
$base_price = 12; // Your price in USD
$EUR_price = round(($base_price * $response_object->rates->EUR), 2);
}
catch(Exception $e) {
// Handle JSON parse error...
}
}
This is working fine. The snippet is from: https://www.exchangerate-api.com/docs/php-currency-api
Solution 2
This method is using Yahoo currency API Full tutorial : Currency Converter in PHP, Python, Javascript and jQuery
function currencyConverter($currency_from, $currency_to, $currency_input) {
$yql_base_url = "http://query.yahooapis.com/v1/public/yql";
$yql_query = 'select * from yahoo.finance.xchange where pair in ("' . $currency_from . $currency_to . '")';
$yql_query_url = $yql_base_url . "?q=" . urlencode($yql_query);
$yql_query_url .= "&format=json&env=store%3A%2F%2Fdatatables.org%2Falltableswithkeys";
$yql_session = curl_init($yql_query_url);
curl_setopt($yql_session, CURLOPT_RETURNTRANSFER, true);
$yqlexec = curl_exec($yql_session);
$yql_json = json_decode($yqlexec, true);
$currency_output = (float) $currency_input * $yql_json['query']['results']['rate']['Rate'];
return $currency_output;
}
$currency_input = 2;
//currency codes : http://en.wikipedia.org/wiki/ISO_4217
$currency_from = "USD";
$currency_to = "INR";
$currency = currencyConverter($currency_from, $currency_to, $currency_input);
echo $currency_input . ' ' . $currency_from . ' = ' . $currency . ' ' . $currency_to;
Solution 3
An example of converting EUR to USD
$url = 'http://www.webservicex.net/CurrencyConvertor.asmx/ConversionRate?FromCurrency=EUR&ToCurrency=USD';
$xml = simpleXML_load_file($url,"SimpleXMLElement",LIBXML_NOCDATA);
if($xml === FALSE)
{
//deal with error
}
else {
$rate = $xml;
}
Solution 4
My 2017 solution is a very lightweight function that gets the current exchange informations from the fixer.io
API. It also stores the exchange rate in a daily cookie to prevent further heavy web loading time. You can also choose Sessions for that or remove it:
function convertCurrency($amount, $from = 'EUR', $to = 'USD'){
if (empty($_COOKIE['exchange_rate'])) {
$Cookie = new Cookie($_COOKIE);
$curl = file_get_contents_curl('http://api.fixer.io/latest?symbols='.$from.','.$to.'');
$rate = $curl['rates'][$to];
$Cookie->exchange_rate = $rate;
} else {
$rate = $_COOKIE['exchange_rate'];
}
$output = round($amount * $rate);
return $output;
}
Example usage to convert 100 euro to pounds:
echo convertCurrency(100, 'EUR', 'GBP');
Solution 5
Use Given code for currency Converter PHP
public function convertCurrency($from, $to, $amount)
{
$url = file_get_contents('https://free.currencyconverterapi.com/api/v5/convert?q=' . $from . '_' . $to . '&compact=ultra');
$json = json_decode($url, true);
$rate = implode(" ",$json);
$total = $rate * $amount;
$rounded = round($total);
return $total;
}
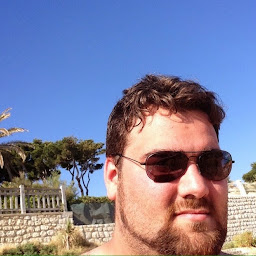
Comments
-
gregory boero.teyssier over 3 years
I'm looking for a way to convert any amount from one currency to another on a website. The user would enter something like '100' and select USD as the currency, and then chooses Australian or Canadian dollars as the currency to convert to. When he clicks the 'Convert' button, I'd like to convert that amount automatically, through some API, and show him the amount in the currency he chose to convert to.
Any ideas?
-
Doggie52 over 10 yearsThis is definitely cleaner and a more robust solution than the others mentioned here.
-
reformed almost 8 yearsiGoogle was retired on November 1, 2013. This API no longer works.
-
reformed almost 8 yearsiGoogle was retired on November 1, 2013. This API no longer works.
-
AlexioVay over 6 yearsIn 2017 and above I don't encourage to use this function since it adds up a huge page loading time. Especially if you convert multiple numbers, because this isn't an API, it downloads the whole webpage and just extracts HTML for values you're looking for. Instead take a look at my answer using the
fixer.io
API. -
Johncl about 6 years404 Not Found - this service is not longer available.
-
Johncl about 6 yearsWebsite seems to require you to register for an API key.
-
Johncl about 6 yearsIt seems its still possible to call this without a key, although it seems your assumption about its format is wrong. It always uses base currency EUR by default, and what you provide as symbols is what currencies you want rates for towards that base currency. You have to do the math yourself to go between those based on those rates.
-
Tino Costa 'El Nino' almost 6 years
-
Johncl about 5 yearsFixer is no longer free.
-
Behiry about 5 yearsno, there is still a free plan for 1.000 API Calls / month
-
Zain Farooq almost 5 years
{"success":false,"error":{"code":103,"info":"This API Function does not exist."}}
-
Behiry over 4 years@ZainFarooq Please try the updated code after getting a free account at fixer