Custom Calendar Date range swipe selection android
Solution 1
As there are many Paid Calendars, I have developed an opensource Unpaid Calendar, which can be used to select dates while swiping over them. Which looks like this
Refer the source code at SwipeDateCalendar
which also can be further modified as per requirements.
Solution 2
With the Default Material Components for Android, You can use the new MaterialDatePicker. In which you can get a single date selection as well as date range selection.
add the following to your build.gradle
implementation 'com.google.android.material:material:1.2.0 alpha02'
Call below method for open date range picker
private fun setupRangePickerDialog() {
val builder:MaterialDatePicker.Builder<*> =MaterialDatePicker.Builder.dateRangePicker()
val constraintsBuilder = CalendarConstraints.Builder()
try {
builder.setCalendarConstraints(constraintsBuilder.build())
val picker:MaterialDatePicker<*> =builder.build()
getDateRange(picker)
picker.show(supportFragmentManager, picker.toString())
} catch (e:IllegalArgumentException){
}
}
Now put below method for getting start and end date
private fun getDateRange(materialCalendarPicker:MaterialDatePicker<out Any>) {
materialCalendarPicker.addOnPositiveButtonClickListener({
selection:Any ? ->
Log.e("DateRangeText", materialCalendarPicker.headerText)})
materialCalendarPicker.addOnNegativeButtonClickListener({
dialog:View ? ->})
materialCalendarPicker.addOnCancelListener({
dialog:DialogInterface ? ->})
}
Congrats, You are done!
Solution 3
use this custom calender , in build.gradle
implementation 'com.savvi.datepicker:rangepicker:1.2.0'
and xml file
<com.savvi.rangedatepicker.CalendarPickerView
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
android:id="@+id/calendar_view"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:paddingLeft="16dp"
android:paddingRight="16dp"
android:paddingBottom="16dp"
android:scrollbarStyle="outsideOverlay"
android:clipToPadding="false"
app:tsquare_dividerColor="@color/calendar_bg"
app:tsquare_headerTextColor="@color/calendar_selected_day_bg"
/>
and java file
Calendar pastYear = Calendar.getInstance();
pastYear.add(Calendar.YEAR, -2);
Calendar nextYear = Calendar.getInstance();
nextYear.add(Calendar.DATE,1);
CalendarPickerView calendar = (CalendarPickerView) findViewById(R.id.calendar_view);
calendar.init(pastYear.getTime(), nextYear.getTime()) //
.inMode(CalendarPickerView.SelectionMode.RANGE)
.withSelectedDate(new Date());
calendar.setTypeface(Typeface.SANS_SERIF);
Solution 4
implementation 'com.google.android.material:material:1.3.0-alpha01'
use this dependency add to your build.gradle
this is on button click set this code :
MaterialDatePicker.Builder<Pair<Long, Long>> builder = MaterialDatePicker.Builder.dateRangePicker();
CalendarConstraints.Builder constraintsBuilder = new CalendarConstraints.Builder();
builder.setTheme(R.style.CustomThemeOverlay_MaterialCalendar_Fullscreen);
builder.setCalendarConstraints(constraintsBuilder.build());
MaterialDatePicker picker = builder.build();
assert getFragmentManager() != null;
picker.show(getFragmentManager(), picker.toString());
add to into your style.xml:
<style name="CustomThemeOverlay_MaterialCalendar_Fullscreen" parent="@style/ThemeOverlay.MaterialComponents.MaterialCalendar.Fullscreen">
<item name="materialCalendarStyle">@style/Custom_MaterialCalendar.Fullscreen</item>
<item name="colorPrimary">@color/colorPrimary</item>
<item name="colorOnSurface">@color/colorTextPrimary</item>
</style>
<style name="Custom_MaterialCalendar.Fullscreen" parent="@style/Widget.MaterialComponents.MaterialCalendar.Fullscreen">
<item name="android:windowFullscreen">false</item>
</style>
add into your whole project style Apptheme this :
<item name="materialCalendarStyle">@style/Widget.MaterialComponents.MaterialCalendar</item>
<item name="materialCalendarFullscreenTheme">@style/ThemeOverlay.MaterialComponents.MaterialCalendar.Fullscreen</item>
<item name="materialCalendarTheme">@style/ThemeOverlay.MaterialComponents.MaterialCalendar</item>
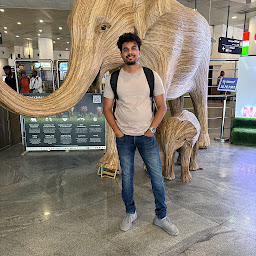
Sachin K Pissay
Updated on June 16, 2022Comments
-
Sachin K Pissay about 2 years
I need to design a custom calendar to choose dates while
swiping over them. I need some solution on how to implement swipe on the calendar and also will be helpfull if there are any libraries which support this feature.
Any type of help will be considered. thank you! The swipe date UI image is as shown
-
Priya almost 7 yearscan u give some idea on how to change the start day from Monday to sunday in your source..?
-
Priya almost 7 yearsI have done what u mentioned above, but still i not getting. Where i have changed is, GregorianCalendar cal = new GregorianCalendar(yy, lCurrentMonth, 1);cal.setFirstDayOfWeek(Calendar.MONDAY); in CalenderActivity in your code.
-
Sachin K Pissay almost 7 years@Priya If you have referred SwipeDateCalendar . I have created an instance of GregorianCalendar. Use that instance and set your preferred first day as shown.
GregorianCalendar cal = new GregorianCalendar(yy, currentMonth, 1); // changing the value to 0 - starts day from monday changing the value to 1 - starts day from sunday and so on..
-
Mr. Mad about 5 yearsHello Priya , Did your made the calenderview as you wanted as you posted above ?