Custom Google Map markers on Directions API
13,188
Change
directionsService.route(request, function(response, status) {
if (status == google.maps.DirectionsStatus.OK) {
directionsDisplay.setDirections(response);
}
});
To
directionsService.route(request, function(response, status) {
if (status == google.maps.DirectionsStatus.OK) {
directionsDisplay.setDirections(response);
var leg = response.routes[ 0 ].legs[ 0 ];
makeMarker( leg.start_location, icons.start, "title" );
makeMarker( leg.end_location, icons.end, 'title' );
}
});
And don't forget to add makeMarker()
function.
Also you will need both start and end icons
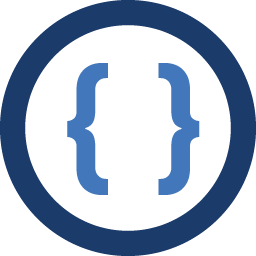
Author by
Admin
Updated on June 04, 2022Comments
-
Admin almost 2 years
I'm totally new to the Google maps api, and after putting together several examples found around the web, I'm struggling on the last hurdle of adding custom markers to the directions. Here's my code:
var directionDisplay; var directionsService = new google.maps.DirectionsService(); function initialize() { var latlng = new google.maps.LatLng([[showMapLatitude]],[[showMapLongitude]]); directionsDisplay = new google.maps.DirectionsRenderer({suppressMarkers: true}); var myOptions = { zoom: 14, center: latlng, mapTypeId: google.maps.MapTypeId.ROADMAP, mapTypeControl: false }; var stylez = [ { featureType: "all", elementType: "all", stylers: [ { saturation: -100 } ] } ]; var image = new google.maps.MarkerImage('/icon.png', new google.maps.Size(20, 33), new google.maps.Point(0,0), new google.maps.Point(10,33) ); var map = new google.maps.Map(document.getElementById("map_canvas"),myOptions); var mapType = new google.maps.StyledMapType(stylez, { name:"Grayscale" }); map.mapTypes.set('tehgrayz', mapType); map.setMapTypeId('tehgrayz'); directionsDisplay.setMap(map); directionsDisplay.setPanel(document.getElementById("directionsPanel")); var marker = new google.maps.Marker({ position: latlng, map: map, title:"[[*pagetitle]]", icon: image }); } function calcRoute() { $(".storeDetails").hide(); $(".storeAdress").hide(); $(".backtocontact").show(); var start = document.getElementById("routeStart").value; var end = "[[showMapLatitude]],[[showMapLongitude]]"; var request = { origin:start, destination:end, travelMode: google.maps.DirectionsTravelMode.DRIVING }; directionsService.route(request, function(response, status) { if (status == google.maps.DirectionsStatus.OK) { directionsDisplay.setDirections(response); } }); }
There's an example of how to do it here: Change individual markers in google maps directions api V3
But being a noob, I can't seem to drop that in the right place here, it either errors or does nothing.
-
Admin over 11 yearsThanks for the help David, however the client invariably changed their mind, and now wants the standard markers on the directions. There's a new bug however, in that the original custom marker is still visible after loading in directions. Any idea how I'd go about hiding it on directions load?
-
Admin over 11 yearsThanks for responding again David, really appreciated, but I meant on directionsDisplay, hide the original custom marker (the image var), leaving the standard Google direction markers. I've tried setting marker.map to null within the calcRoute function, but it can't find the marker var, even if I set it outside of the initialize function (which would make it global, unless I'm mistaken?).
-
david strachan over 11 yearsYou don't require a Marker Google supplies it. You need to removesupressMarkers as in answer.
-
Admin over 11 yearsSorry I'm not explaining myself. The map I have so far, shows location, with a custom marker (which the client wants), and an input box which when fired off, makes directions from the address entered, to that custom marker. When this happens, Google drops in the standard direction markers, but the custom marker is still visible as well, at a slightly different location. The client wants to lose the custom marker ONLY after the directions are displayed. Hope that makes sense. I've since removed suppress markers by the way.
-
david strachan over 11 yearsTo remove markers you need to push marker into array see
http://stackoverflow.com/questions/1544739/google-maps-api-v3-how-to-remove-all-markers
Infunction calcRoute() { // First, remove any existing markers from the map. for (i = 0; i < markerArray.length; i++) { markerArray[i].setMap(null); } // Now, clear the array itself. markerArray = [];