Custom serialization with DataContractSerializer
The DataContractAttribute and the SerializableAttribute can both be used together. The bonus here is, you don't need to use seperate serialisers either. The DataContractSerialzer is an XmlObjectSerializer, which itself supports [Serializable]. For instance:
[Serializable]
public class TestClass
{
public string Name { get; set; }
}
{
var formatter = new DataContractSerializer(typeof(TestClass));
using (var stream = new MemoryStream())
{
var instance = new TestClass { Name = "Matt" };
formatter.WriteObject(stream, instance);
stream.Seek(0, SeekOrigin.Begin);
var second = (TestClass) formatter.ReadObject(stream);
Console.WriteLine(second.Name);
}
}
OUTPUT: "Matt"
Using a just a SerializableAttribute attribute we can successfully serialise and deserialise an object using the DataContractSerializer...
Using ISerializable, we can do the same thing:
[Serializable]
public class TestClass2 : ISerializable
{
public TestClass2() { }
protected TestClass2(SerializationInfo info, StreamingContext context)
{
Name = info.GetString("name").ToUpper();
}
public void GetObjectData(SerializationInfo info, StreamingContext context)
{
info.AddValue("name", Name);
}
public string Name { get; set; }
}
{
var formatter = new DataContractSerializer(typeof(TestClass2));
using (var stream = new MemoryStream())
{
var instance = new TestClass2 { Name = "Matt" };
formatter.WriteObject(stream, instance);
stream.Seek(0, SeekOrigin.Begin);
var second = (TestClass2)formatter.ReadObject(stream);
Console.WriteLine(second.Name);
}
}
OUTPUT: "MATT"
And with a DataContractAttribute:
[DataContract, Serializable]
public class TestClass3
{
public int Age { get; set; }
[DataMember]
public string Name { get; set; }
}
{
var formatter = new DataContractSerializer(typeof(TestClass3));
using (var stream = new MemoryStream())
{
var instance = new TestClass3 { Name = "Matt", Age = 26 };
formatter.WriteObject(stream, instance);
stream.Seek(0, SeekOrigin.Begin);
var second = (TestClass3)formatter.ReadObject(stream);
Console.WriteLine(second.Name);
Console.WriteLine(second.Age);
}
}
OUTPUT: "Matt"
OUTPUT: 0
When the DataContractSerializer encounters a type with a DataContractAttribute, it will use that instead of passing serialization to its base type, which handles the SerializableAttribute and ISerializable interfaces.
If you are encountering issues, is it with serialisation, or with deserialisation, or both?
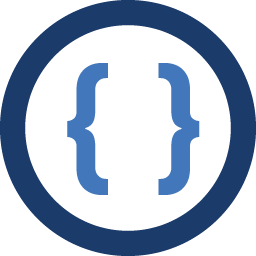
Admin
Updated on July 19, 2022Comments
-
Admin almost 2 years
I'm currently using wrapper classes for my DataSets ,in order to implement custom serialization. I would like to use
DataContractSerializer
(more like have to use it) but still support the custom serialization. The problem is that the[DataContract]
and[Serializable]
attributes don't seem to get along so well... how could I override the serialization, and support BOTH DataContract & ISerializable serialization? The code for the wrapper DataSet class is brought here:[Serializable()] [System.Runtime.InteropServices.ComVisible(false)] public class TestDatasetWrapper : TestDataSet, ISerializable { public TestDatasetWrapper() : base() {} protected TestDatasetWrapper(SerializationInfo info, StreamingContext context) { SerializationHelper.DeserializeTypedDataSet(info, this); } public override void GetObjectData(SerializationInfo info, StreamingContext context) { SerializationHelper.AddTypedDataSetObjectData(info, this); } }
Thanks!
-
dotNET almost 7 yearsAll 3 of your samples seem to be missing the function signature line.