Custom UISegmentedControl
Solution 1
I had to add this extra code, in addition to having 2 different states for the "on" and "off" positions:
- (void)viewDidLoad
{
[super viewDidLoad];
// Set set segControl background to transparent
CGRect rect = CGRectMake(0, 0, 1, 1);
UIGraphicsBeginImageContext(rect.size);
CGContextRef context = UIGraphicsGetCurrentContext();
CGContextSetFillColorWithColor(context, [[UIColor clearColor] CGColor]);
CGContextFillRect(context, rect);
UIImage *transparentImage = UIGraphicsGetImageFromCurrentImageContext();
UIGraphicsEndImageContext();
[self.segControl setBackgroundImage:transparentImage forState:UIControlStateNormal barMetrics:UIBarMetricsDefault];
[self.segControl setDividerImage:transparentImage forLeftSegmentState:UIControlStateNormal rightSegmentState:UIControlStateNormal barMetrics:UIBarMetricsDefault];
}
EDIT: Because this is getting some publicity, a cleaner solution is to use [UIImage new] instead of creating transparent images, as such:
[self.segControl setDividerImage:[UIImage new] forLeftSegmentState:UIControlStateNormal rightSegmentState:UIControlStateNormal barMetrics:UIBarMetricsDefault];
[self.segControl setBackgroundImage:[UIImage new] forState:UIControlStateNormal barMetrics:UIBarMetricsDefault];
Solution 2
You can use the methods which are described in the iOS developer libary:
Scroll down to the "Customizing Appearance" section. There are methods to set background images for several button states, button divider images, etc.
These methods are only available in iOS5 and later.
@property tintColor
– backgroundImageForState:barMetrics:
– setBackgroundImage:forState:barMetrics:
– contentPositionAdjustmentForSegmentType:barMetrics:
– setContentPositionAdjustment:forSegmentType:barMetrics:
– dividerImageForLeftSegmentState:rightSegmentState:barMetrics:
– setDividerImage:forLeftSegmentState:rightSegmentState:barMetrics:
– titleTextAttributesForState:
– setTitleTextAttributes:forState:
Solution 3
The simplest way would be to create your own control that mimics UISegmentedControl
. UISegmentedControl
just arranges a series of buttons and manages their image states for you; it doesn't do anything special.
Solution 4
I wrote something that works as @rpetrich was explaining without placing in a array and in my opinion is the easiest solution to this. Hope someone finds this useful
.h
IBOutlet UIButton *index0;
IBOutlet UIButton *index1;
IBOutlet UIButton *index2;
IBOutlet UIImageView *segMentControl;
-(IBAction)segmentSwitch:(UIButton *) buttonIndexPressed;
.m
-(IBAction)segmentSwitch:(UIButton *) buttonIndexPressed
{
if (buttonIpressed == index0)
{
[segmentControl setImage:[UIImage imageNamed:@"Seg1Sel.png"]];
NSLog(@"index 0 pushed");
index0.enabled = NO;
index1.enabled = YES;
index2.enabled = YES;
}
else if (buttonIpressed == index1)
{
[segmentControl setImage:[UIImage imageNamed:@"Seg2Sel.png"]];
NSLog(@"index 1 pushed");
index0.enabled = YES;
index1.enabled = NO;
index2.enabled = YES;
}
else if (buttonIpressed == index2)
{
[segmentControl setImage:[UIImage imageNamed:@"Seg3Sel.png"]];
NSLog(@"index 2 pushed");
index0.enabled = YES;
index1.enabled = YES;
index2.enabled = NO;
}
}
Solution 5
Yes, you you DO need 2 images (on and off) for each section of the segment bar. (4 segments... 8 images.) But it lets you set a total of 16 choices! (All with only consuming 1 row in your GUI.)
I got everything working EXCEPT... how do you hide the original segment bar graphics?
Can't set alpha to 0. (It will also hide your images.)
Can't set "tintClear" to "clear". (Not sure why it makes it black and white.)
Can't set it to "hidden"... nothing will work at all.
Can't set "background" to "clear". (The background is NOT the segmentbar graphics.)
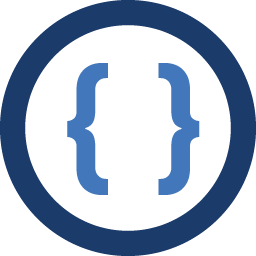
Admin
Updated on March 12, 2020Comments
-
Admin about 4 years
How do I make a custom
UISegmentedControl
?I have 2 images, 1 that should be displayed when the segment is active and the other if the segment is inactive. Can i override the style or something so i have a
UISegmentedControl
with my own images as active/inactive background?