Cypress causing type errors in jest assertions
Solution 1
I ran into this problem yesterday. It seems that what you are saying is correct, cypress and jest both declares types for expect
. In this case the cypress declaration seem to be the one that is picked up. Here's an issue from the cypress repo regarding this:
https://github.com/cypress-io/cypress/issues/1603
The solution mentioned in there worked for me. You need to exclude the jest spec files from in tsconfig.json
and then declare a tsconfig.spec.json
where they are explicitly included again.
tsconfig.json:
{
...,
"exclude": [
"**/*.spec.ts"
]
}
tsconfig.spec.json:
{
"extends": "./tsconfig.json",
"include": [
"**/*.spec.ts"
],
...
}
With this in place, both my (angular 8) app compiles fine and I can run the jest tests without issue. Here's another example mentioned in the issue with a similar fix being implemented:
Solution 2
There is an official Cypress repo that shows how to fix this exact problem https://github.com/cypress-io/cypress-and-jest-typescript-example
I wasn't able to get that method to work in one of my projects, so I am using this as a workaround:
import { expect } from '@jest/globals';
Solution 3
This worked for me in my tsconfig.json
I had to add
"include": ["src/**/*"],
Complete code here
{
"compilerOptions": {
"outDir": "./dist/",
"noImplicitAny": true,
"module": "commonjs",
"target": "es5",
"jsx": "react",
"allowJs": true,
"allowSyntheticDefaultImports" : true,
"esModuleInterop" : true,
"sourceMap": true,
"experimentalDecorators": true
},
"include": ["src/**/*"],
"exclude": [
"node_modules",
"**/*.spec.ts"
]
}
Solution 4
Finally I found the solution!
Just add "cypress"
to the exclude
property of your main tsconfig.json
:
{
...YOUR_CONFIGS,
"compilerOptions": {
...YOUR_CONFIGS,
"typeRoots": [ // THIS TO GET ALL THE TYPES
"node_modules/@types"
],
},
"exclude": ["cypress"],
}
You should add also another tsconfig.json
for your cypress tests. You can create a cypress folder and add that tsconfig.json
there.
The following is my Cypress tsconfig.json
:
{
"compilerOptions": {
"strict": true,
"baseUrl": "../node_modules",
"target": "es5",
"lib": ["es5", "dom"],
"types": ["cypress", "@testing-library/cypress"]
},
"include": ["./**/*.ts"]
}
Solution 5
The simplest way for solving this issue is to add this line to tsconfig.json:
"include": [
"src/**/*.ts"
],
I am attaching my tsconfig file for better understanding. You can see the addition in lines 3-5.
{
"compileOnSave": false,
"include": [
"src/**/*.ts"
],
"compilerOptions": {
"baseUrl": "./",
"outDir": "./dist/out-tsc",
"sourceMap": true,
"declaration": false,
"downlevelIteration": true,
"experimentalDecorators": true,
"module": "esnext",
"moduleResolution": "node",
"importHelpers": true,
"target": "es2015",
"typeRoots": [
"node_modules/@types"
],
"lib": [
"es2018",
"dom"
]
},
"angularCompilerOptions": {
"fullTemplateTypeCheck": true,
"strictInjectionParameters": true
}
}
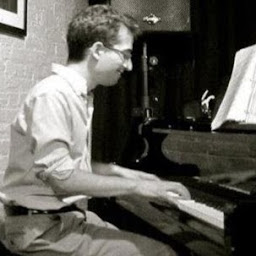
Jonathan Tuzman
I develop web and desktop applications, primarily with Typescript, React, and Redux. I've also done a good deal of work in React Native, iOS/Swift, WPF/C#, Python (Flask), Ruby on Rails, C++, and certainly others I'm forgetting. Most of my work leans toward front end development, but I really enjoy touching all parts of the stack. What excites me most is working on products that "normal" people (which is to say, not specialists in any given area) use and touch in their everyday lives, that makes their tasks and their passions easier. In my other life, I'm a professional musician, and I fell in love with coding after teaching myself Swift and building an app for audiences at my piano bar gigs. I dove deep into software development, and continue to gobble up new languages and frameworks.
Updated on July 12, 2022Comments
-
Jonathan Tuzman almost 2 years
I had been using
react-testing-library
as well as@testing-library/jest-dom/extend-expect
. I installed Cypress yesterday, and now I'm getting Typescript errors on all my jest matchers:Property 'toEqual' doesn't exist on type 'Assertion'. Did you mean 'equal'?
It looks like it's getting the type of
expect
from the wrong assertion library or something? Also,expect(...).to.equal(...)
doesn't even work either.I actually tried installing
@types/jest
and yarn appears to have succeeded but it's not listed in mypackage.json
'sdevDependencies
.Here's my
tsconfig
{ "compilerOptions": { "target": "es5", "lib": [ "dom", "dom.iterable", "esnext" ], "allowJs": true, "skipLibCheck": true, "esModuleInterop": true, "allowSyntheticDefaultImports": true, "strict": true, "noImplicitAny": false, "forceConsistentCasingInFileNames": true, "module": "esnext", "moduleResolution": "node", "resolveJsonModule": true, "isolatedModules": false, "noEmit": true, "jsx": "react", "skipDefaultLibCheck": true, "types": [ "node", "cypress", "jest" ] }, "include": [ "src" ] }
I'll also mention that all my
cy
calls in my cypress tests are getting acy is not defined
error from ESLint. -
natterstefan about 3 yearsThat was the key for me too. adding
src/**
in the roottsconfig.json
solved it after looking at the other settings in the example repo: github.com/cypress-io/cypress-and-jest-typescript-example. -
foakesm over 2 yearsDo you mean the Cypress
.spec
files? -
Johan Persson over 2 years@foakesm Sorry if it is unclear from the text above. The base
tsconfig.json
excludes the jest.spec
files, and then thetsconfig.spec.json
(that jest uses) includes them. For this to work, we're assuming you Cypress tests are not in files named*.spec.ts
. Does that answer your question? -
Rick over 2 yearsI have followed the official Cypress repo. Thanks for the link.
-
Caleb Waldner about 2 yearsGreat suggestion with the
exclude:[cypress]
, that did the trick for me.