Cython Compilation Error: dynamic module does not define module export function
Solution 1
You need to call setup.py with Python 3 (python3 setup.py build_ext
, maybe --inplace
). It's because Python 3 defines a different name for the init
function called when the module starts, and so you need to build it using Python 3 to ensure the correct name is generated.
See dynamic module does not define init function (PyInit_fuzzy) and How to specify Python 3 source in Cython's setup.py? for slightly more detail (it's bordering on a duplicate of these questions, but isn't quite in my view)
Solution 2
I experienced this and found that I had to use the same name of .pyx as the module name, e.g.
makefile:
# (default)
# INSTALL_DIR:=/usr/lib/python3.6/site-packages
# (my venv)
INSTALL_DIR:=/home/<username>/python3_venv/lib/python3.6/site-packages
all:
sudo python3 setup_myproj.py install --install-lib ${INSTALL_DIR}
setup_myproj.py
from distutils.core import setup, Extension
from Cython.Build import cythonize
ext = Extension("myproj",
sources=["myproj.pyx", "myCppProjFacade.cpp"],
<etc>
language="c++"
)
setup(name="myproj",
version="0.0.1",
ext_modules=cythonize(ext))
client module, run after installing to venv
import myproj as myCppProjWrapper
...
I also found that if the "myproj" names are different, under <python-lib-dir>/<python-vers>/site-packages
the .so and .egg-info names are different and the client fails to load it.
In addition I found that the client's environment does not need to have the cython
package installed.
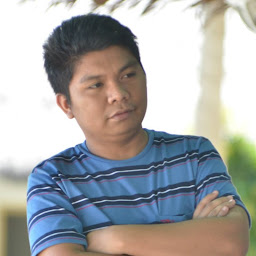
Alger Remirata
Updated on July 14, 2020Comments
-
Alger Remirata almost 4 years
I am building a package in Cython. I am using the following as the structure for
setup.py
:from distutils.core import setup from distutils.extension import Extension from Cython.Build import cythonize import numpy import scipy extensions = [ Extension("xxxxx",["xxxx/xxxxx.pyx"], include_dirs=[numpy.get_include(),"."]), Extension("nnls",["xxxxx/xxxxx.pyx"], include_dirs=[numpy.get_include(),"."]), ] setup( name='xxxxxx', version='0.0.0', description='''********''', url='xxxxxxx', author='xxxxx', author_email='xxxxx', packages=[ 'xxxxx', ], install_requires=[ 'cython', 'numpy', 'scipy', ], ext_modules=cythonize(extensions), )
However, I am getting an error upon installation in Python 3. It is working in Python 2 however, it is not compiling in Python 3 having the following error:
dynamic module does not define module export function
How can I solve this problem? Is the structure of the
setup.py
the reason why this is not compiling?