Dapper Call stored procedure and map result to class
22,098
Solution 1
You call wrong method:
public void Load(CustomTest obj, Guid RequestId)
{
using (var con = base.GetClosedConnection())
{
con.Open();
//result is list of CustomTest
var result = db.Query<CustomTest>("GetRequestTest", new {RequestId},
commandType: CommandType.StoredProcedure);
}
}
How to use dapper: https://github.com/StackExchange/dapper-dot-net
Solution 2
using (var con = base.GetClosedConnection())
{
var result = conn.Query<CustomTest>("exec [dbo].[GetRequestTest] @id", new {Id = RequestId});
}
Column names stored procedure or query returns should be same as CustomTest`s property names (e.g. Amount, Checksum). As result you will receive IEnumerable filled with appropriate data.
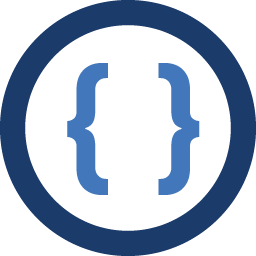
Author by
Admin
Updated on July 09, 2022Comments
-
Admin almost 2 years
I have a T-SQL stored procedure:
CREATE PROCEDURE [dbo].[GetRequestTest] @RequestId UNIQUEIDENTIFIER AS BEGIN SELECT Request.Amount, Request.Checksum FROM Request WHERE RequestId = @RequestId END
C# mapping class:
public class CustomTest : Itest { public decimal Amount {get;set;} public string Checksum { get; set; } }
I'm calling trying to invoke stored procedure by using Dapper:
public void Load(CustomTest obj, Guid RequestId) { using (var con = base.GetClosedConnection()) { con.Open(); var p = new DynamicParameters(); p.Add("@RequestId", dbType: DbType.Guid, direction: ParameterDirection.Input); var result = con.ExecuteReader("[dbo].[GetRequestTest]", param: p, commandType: CommandType.StoredProcedure); while (result.Read()) obj.Amount = (decimal)result["Amount"]; } }
But result is null
I tried to call to put SQL statement from stored procedure directly into C# code - and it works fine, but it doesn't work with stored procedure.
Any ideas - how to make it work?
-
Maximilian Peters about 6 yearsWhile this code may answer the question, it would be better to include some context, explaining how it works and when to use it. Code-only answers are not useful in the long run.
-
Alex Erygin about 6 years@MaximilianPeters, thank you for comment. Answer updated.