Dart CSV Writing
Solution 1
Use package csv
from https://pub.dartlang.org/packages/csv
If you have a List<List<dynamic>>
items that needs to convert into csv,
String csv = const ListToCsvConverter().convert(yourListOfLists);
If you want to write the csv to a file,
/// Write to a file
final directory = await getApplicationDocumentsDirectory();
final pathOfTheFileToWrite = directory.path + "/myCsvFile.csv";
File file = await File(pathOfTheFileToWrite);
file.writeAsString(csv);
Also, if you want to read a csv file directly into list<list<dynamic>>
final input = new File('a/csv/file.txt').openRead();
final fields = await input.transform(UTF8.decoder).transform(csvCodec.decoder).toList();
Solution 2
According to new packages and guidelines(2019) use following code
package csv
from https://pub.dartlang.org/packages/csv.
If you have a List<List<dynamic>>
items that needs to convert into csv,
String csv = const ListToCsvConverter().convert(yourListOfLists);
Then you can write the string to a file using file operations.
file.writeAsString('$csv');
Also, if you want to read a csv file directly into list<list<dynamic>>
final input = new File('a/csv/file.txt').openRead();
final fields = await input.transform(utf8.decoder).transform(new CsvToListConverter()).toList();
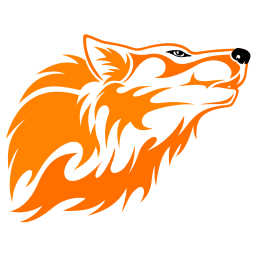
Lloyd7113
Updated on December 08, 2022Comments
-
Lloyd7113 7 minutes
Hey so I haven't really messed around with it too much, but I was wondering if there was actually a way (before I go down a neverending rabbit hole) to read and write to CSV files in Dart/Flutter? I need to write to the files, not necessarily read them, and I'm willing to go to quite extreme lengths to do so. Any library works, built-in functions are even better. Any and all help is appreciated!
-
Fethi over 2 yearswhen inserting to a CSV file, how to specify which file exactly?
-
krishnakumarcn over 2 yearsIn the above example, the file is
a/csv/file.txt
. In an actual scenario, you can get your filepath from reading external storage directory or app directory. Usepath_provider
package for getting the directory path info. @Fethi -
Fethi over 2 yearsbut in the first code you mentioned when inserting you didn't specify any path, how does it insert to a it ?
-
krishnakumarcn over 2 yearsUpdated the answer for the same @Fethi
-
Michael Tolsma about 2 yearsHow would you append more data to the existing sheet? using
mode: FileMode.append
appends to the last entry without spaces or going to a new line, and doing"$csv\n"
just gets overwritten by the next entry.