DataTable : How to hide the pagination and only show it as need?
Solution 1
Use drawCallback option to handle DT draw event and show/hide pagination control based on available pages:
$('#table_id').dataTable({
drawCallback: function(settings) {
var pagination = $(this).closest('.dataTables_wrapper').find('.dataTables_paginate');
pagination.toggle(this.api().page.info().pages > 1);
}
})
Solution 2
$('#dataTable_ListeUser').DataTable( {
//usual pager parameters//
"drawCallback": function ( settings ) {
/*show pager if only necessary
console.log(this.fnSettings());*/
if (Math.ceil((this.fnSettings().fnRecordsDisplay()) / this.fnSettings()._iDisplayLength) > 1) {
$('#dataTable_ListeUser_paginate').css("display", "block");
} else {
$('#dataTable_ListeUser_paginate').css("display", "none");
}
}
});
Solution 3
Use 'drawCallback' to solve this problem.
1.On the webpage, use developer tool to inspect the 'previous' button, then you will find a div element that wraps both the 'previous' and 'next' buttons. DataTables automatically assigned an id to this div based on your html table element's id.
For example, I have a table with id 'Atable'. In this table, DataTables automatically created a div element with id called 'Atable_paginate' to wrap the previous and next buttons.
2.For drawCallback, we write a function which checks if there is less than 1 page, if so, we hide element by utilising id.
For example, you have set there are 20 records on one page by
pageLength: 20,
which means if there are less then 20 records, we don't need to display 'previous' and 'next' buttons. So we write like below,
drawCallback: function(settings){
if($(this).find('tbody tr').length <= 20){
$('#Atable_paginate').hide();
}
}
3.The initialization of Atable should be like below
var table = $('#Atable').DataTable({
pageLength: 20,
lengthChange: false,
drawCallback: function(settings){
if($(this).find('tbody tr').length <= 20){
$('#Atable_paginate').hide();
}
}
});
4.If there are more than one table on the webpage, apply this method on each table.
Solution 4
You can use fnDrawCallback() method to hide the pagination in dataTable.
var oTable = $('#datatable_sample').dataTable({
"iDisplayLength": 10,
"fnDrawCallback": function(oSettings) {
if ($('#datatable_sample tr').length < 10) {
$('.dataTables_paginate').hide();
}
}
});
The length which you can define as per the row you want to display in the listing.
Solution 5
$(this)
did not work for me, probably because I am using TypeScript. So I used a different approach to get the JQuery element for the table wrapper and the DataTables API. This has been inspired by the answer of BitOfUniverse and tested with DataTables 1.10.
TypeScript:
'drawCallback': (settings: any) => {
// hide pager and info if the table has NO results
const api = new $.fn.dataTable.Api(settings);
const pageCount = api.page.info().pages;
const wrapper = $('#' + settings.sTableId).closest('.dataTables_wrapper');
const pagination = wrapper.find('.dataTables_paginate');
const info = wrapper.find('.dataTables_info');
pagination.toggle(pageCount > 0);
info.toggle(pageCount > 0);
}
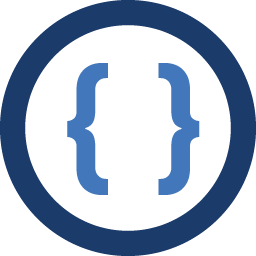
Admin
Updated on July 09, 2022Comments
-
Admin almost 2 years
I have 2 tables that are using DataTable jQuery Plug-in. I wondering if there is a way to hide my pagination on the bottom right of my table.
Note:
- Only show the pagination when I need one.
- Hide the pagination when the query results is less than 10.