Date Format in Swift
Solution 1
You have to declare 2 different NSDateFormatters
, the first to convert the string to a NSDate
and the second to print the date in your format.
Try this code:
let dateFormatterGet = NSDateFormatter()
dateFormatterGet.dateFormat = "yyyy-MM-dd HH:mm:ss"
let dateFormatterPrint = NSDateFormatter()
dateFormatterPrint.dateFormat = "MMM dd,yyyy"
let date: NSDate? = dateFormatterGet.dateFromString("2016-02-29 12:24:26")
print(dateFormatterPrint.stringFromDate(date!))
Swift 3 and higher:
From Swift 3 NSDate
class has been changed to Date
and NSDateFormatter
to DateFormatter
.
let dateFormatterGet = DateFormatter()
dateFormatterGet.dateFormat = "yyyy-MM-dd HH:mm:ss"
let dateFormatterPrint = DateFormatter()
dateFormatterPrint.dateFormat = "MMM dd,yyyy"
if let date = dateFormatterGet.date(from: "2016-02-29 12:24:26") {
print(dateFormatterPrint.string(from: date))
} else {
print("There was an error decoding the string")
}
Solution 2
This may be useful for who want to use dateformater.dateformat
;
if you want 12.09.18
you use dateformater.dateformat = "dd.MM.yy"
Wednesday, Sep 12, 2018 --> EEEE, MMM d, yyyy
09/12/2018 --> MM/dd/yyyy
09-12-2018 14:11 --> MM-dd-yyyy HH:mm
Sep 12, 2:11 PM --> MMM d, h:mm a
September 2018 --> MMMM yyyy
Sep 12, 2018 --> MMM d, yyyy
Wed, 12 Sep 2018 14:11:54 +0000 --> E, d MMM yyyy HH:mm:ss Z
2018-09-12T14:11:54+0000 --> yyyy-MM-dd'T'HH:mm:ssZ
12.09.18 --> dd.MM.yy
10:41:02.112 --> HH:mm:ss.SSS
Here are alternatives:
- era: G (AD), GGGG (Anno Domini)
- year: y (2018), yy (18), yyyy (2018)
- month: M, MM, MMM, MMMM, MMMMM
- day of month: d, dd
- day name of week: E, EEEE, EEEEE, EEEEEE
Solution 3
Swift - 5.0
let date = Date()
let format = date.getFormattedDate(format: "yyyy-MM-dd HH:mm:ss") // Set output format
extension Date {
func getFormattedDate(format: String) -> String {
let dateformat = DateFormatter()
dateformat.dateFormat = format
return dateformat.string(from: self)
}
}
Swift - 4.0
2018-02-01T19:10:04+00:00 Convert Feb 01,2018
extension Date {
static func getFormattedDate(string: String , formatter:String) -> String{
let dateFormatterGet = DateFormatter()
dateFormatterGet.dateFormat = "yyyy-MM-dd'T'HH:mm:ssZ"
let dateFormatterPrint = DateFormatter()
dateFormatterPrint.dateFormat = "MMM dd,yyyy"
let date: Date? = dateFormatterGet.date(from: "2018-02-01T19:10:04+00:00")
print("Date",dateFormatterPrint.string(from: date!)) // Feb 01,2018
return dateFormatterPrint.string(from: date!);
}
}
Solution 4
Swift 3 and higher
let dateFormatter = DateFormatter()
dateFormatter.dateStyle = .medium
dateFormatter.timeStyle = .none
dateFormatter.locale = Locale.current
print(dateFormatter.string(from: date)) // Jan 2, 2001
This is also helpful when you want to localize your App. The Locale(identifier: ) uses the ISO 639-1 Code. See also the Apple Documentation
Solution 5
Swift 3 version with the new Date
object instead NSDate
:
let dateFormatterGet = DateFormatter()
dateFormatterGet.dateFormat = "yyyy-MM-dd HH:mm:ss"
let dateFormatter = DateFormatter()
dateFormatter.dateFormat = "MMM dd,yyyy"
let date: Date? = dateFormatterGet.date(from: "2017-02-14 17:24:26")
print(dateFormatter.string(from: date!))
EDIT: after mitul-nakum suggestion
Related videos on Youtube
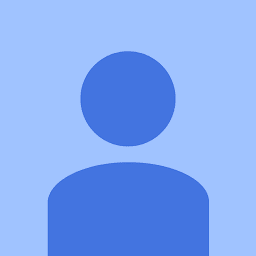
Sydney Loteria
Updated on May 07, 2022Comments
-
Sydney Loteria about 2 years
How will I convert this datetime from the date?
From this: 2016-02-29 12:24:26
to: Feb 29, 2016So far, this is my code and it returns a nil value:
let dateFormatter = NSDateFormatter() dateFormatter.dateFormat = "MM-dd-yyyy" dateFormatter.timeZone = NSTimeZone(name: "UTC") let date: NSDate? = dateFormatter.dateFromString("2016-02-29 12:24:26") print(date)
-
Sydney Loteria about 8 yearsBut how will you convert that to this kind of date format Feb 29, 2016
-
Pavlos about 7 yearsdo you know why I get nil when I try to print this?
-
Mitul Nakum over 6 yearsdateFormatterGet.dateFormat = "yyyy-MM-dd HH:mm:ss" hour formate will require capital HH, as hour is in 24 formate
-
KvnH almost 6 yearsWhat happens if the dateFormatterGet dateFormat needs to accept 2 different formats - one containing milliseconds and one without milliseconds? ie yyyy-MM-dd'T'HH:mm:ssZZZZZ and yyyy-MM-dd'T'HH:mm:ss:SSSZZZZZ
-
LorenzOliveto almost 6 yearsI think you have to declare two different DateFormatters to get the date: if the first one fails (it will return nil), use the second.
-
LorenzOliveto over 5 yearsThis can be compacted using only one date formatter with the format "EEEE\nMMMM dd".
-
Markus over 5 yearsRECTIFICATION: I have tested the code but you do not obtain the letters in uppercase.
-
LorenzOliveto over 5 yearsYes, the uppercase has to be applied to the string returned, like in your answer. The the date formatter doesn't return an uppercase string. Just add .uppercased() like this "dateFormat.string(from: Date()).uppercased()"
-
Victor Engel over 5 yearsIf you want to localize your app, just use
Locale.current
to use the user's locale. -
Devesh.452 about 5 yearsCan you please help me, what will the date format for "Tue Mar 12 2019 12:00:00 GMT-0500 (CDT)"
-
LorenzOliveto about 5 years@Devesh it should be something like this "EEE MMM d yyyy HH:mm:ss ZZZZ", check out nsdateformatter.com it's a very hanful site with all the formats supported
-
Devesh.452 about 5 years@lorenzoliveto yes I have tried all the way for this format I tried on nsdateformatter.com too, still, I am not able to get anything for "Tue Mar 12 2019 12:00:00 GMT-0500 (CDT)" this format. I am getting this format in a JSON. I am not sure wether this is valid string, can you please help me.
-
LorenzOliveto about 5 years@Devesh this should be the format you need "EEE MMM d yyyy HH:mm:ss 'GMT'Z '('z')'"
-
Neph over 4 yearsThis is a good, short solution with just a single
DateFormatter()
! Something to be aware of: TheDateFormatter
also takes the application region (set in the scheme) into account! E.g.2019-05-27 11:03:03 +0000
with the formatyyyy-MM-dd HH:mm:ss
and "Germany" as the region turns into2019-05-27 13:03:03
. This difference is caused by DST: In summer Germany is GMT+2, while in winter it's GMT+1. -
Manoj about 4 years@Devesh E MMM d yyyy HH:mm:ss 'GMT'Z will work for you
-
Piotr Labunski about 4 yearsWhile this code may answer the question, providing additional context regarding how and/or why it solves the problem would improve the answer's long-term value.
-
Muhammad Abbas about 3 yearsOne of the best answer
-
beginner992 almost 3 yearsSuperb answer! Good job.
-
simpleuser almost 3 yearsSee stackoverflow.com/a/55093100/1663987 for how to get the whole list.
-
mahan over 2 years@FontFamily to some extent you are right. I updated the URL but still no overview available.
-
Sulthan over 2 yearsThe documentation says this is available in iOS 15, not related to Swift version.