Decode gzip compressed and base64 encoded data to a readable format
17,750
Solution 1
zlib
successfully decompresses data that it has compressed previously:
>>> data = b'data'
>>> import zlib
>>> compressed = zlib.compress(data)
>>> import base64
>>> original_data = base64.b64encode(compressed).decode()
>>> zlib.decompress(base64.b64decode(original_data))
b'data'
zlib
fails to decompress (with the default settings) gzip
data:
>>> import gzip
>>> gzipped_data = base64.b64encode(gzip.compress(data)).decode()
>>> gzipped_data != original_data
True
>>> print(zlib.decompress(base64.b64decode(gzipped_data)))
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
zlib.error: Error -3 while decompressing data: incorrect header check
>>> gzip.decompress(base64.b64decode(gzipped_data))
b'data'
Solution 2
I had the same problem and solve it this way:
from zipfile import ZipFile
from tempfile import SpooledTemporaryFile
import base64
decoded_base64 = base64.b64decode(base64_zip)
with SpooledTemporaryFile() as tmp:
tmp.write(decoded_base64)
archive = ZipFile(tmp, 'r')
for file in archive.filelist:
content = archive.read(file.filename)
print("The file %s have the content %s" % (file.filename, content))
Using this your code doesn't write a file, I tested. Is very useful because you can easily read a file inside a zip file, previously received by rest for example.
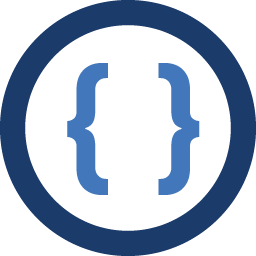
Author by
Admin
Updated on June 14, 2022Comments
-
Admin almost 2 years
Trying to decode gzip compressed and base64 encoded data to a readable format in Python3.4.
import base64 import zlib original_data = '...jU2X0NCQ19TSEEAAAABAAA=' #Data cut short. decoded64 = base64.b64decode(original_data) #format:b'\x16xe\x94...\xae\x9a\...' final_decoded = zlib.decompress(decoded64) print(final_decoded)
Been getting: "Error -2 while preparing to decompress data: inconsistent stream state." Not sure what I'm doing wrong.