Delete List Item on right swipe react-native
11,586
First thing first, Try using fat-arrow functions whenever possible, which will automatically solve your binding issues.
- You will have to use the
extraData= {this.state.activeRow}
in yourflatlist
with newly added stateactiveRow
. - I have updated your
renderItem()
.
Check out the following updated code. Hope this helps.
import * as React from 'react';
import { View, Text, TextInput,
FooterTab, Button, TouchableOpacity, ScrollView, StyleSheet,
ActivityIndicator, Header, FlatList } from 'react-native';
import { Table, Row, Rows } from 'react-native-table-component';
import { createStackNavigator } from 'react-navigation';
import { SearchBar } from 'react-native-elements';
import { Constants } from 'expo';
// You can import from local files
import AssetExample from './components/AssetExample';
// or any pure javascript modules available in npm
import { Card } from 'react-native-paper';
import Swipeout from 'react-native-swipeout';
export default class App extends React.Component {
constructor() {
super();
this.state = {
AbcSdata: null,
loading: true,
search: '',
tableData: [], qrData: '', selectedPriority: '',
selectedIssue: '', selectedReason: '', selectedTriedRestart: '',
selectedPowerLED: '', selectedBurning: '', selectedNoise: '',
rowID:'',
activeRow: null
};
this.setDate = this.setDate.bind(this);
}
swipeBtns = [{
text: 'Delete',
type: 'delete',
backgroundColor: 'red',
underlayColor: 'rgba(0, 0, 0, 1, 0.6)',
onPress: () => {
console.log("Deleting Row with Id ", this.state.activeRow);
this.deleteNote(this.state.activeRow);
}
}];
removeItem = (items, i) =>
items.slice(0, i).concat(items.slice(i + 1, items.length));
deleteNote = (rowIndex) => {
//add your custome logic to delete the array element with index.
// this will temporary delete from the state.
let filteredData = this.removeItem(this.state.AbcSdata,rowIndex);
this.setState({AbcSdata: [] },()=> {
this.setState({AbcSdata: filteredData },()=> {console.log("Row deleted.", rowIndex)});
});
};
setDate(newDate) {
}
_loadInitialState = async () => {
const { navigation } = this.props;
const qdata = navigation.getParam('data', 'NA').split(',');
var len = qdata.length;
const tData = [];
console.log(len);
for (let i = 0; i < len; i++) {
var data = qdata[i].split(':');
const entry = []
entry.push(`${data[0]}`);
entry.push(`${data[1]}`);
tData.push(entry);
}
this.setState({ tableData: tData });
console.log(this.state.tableData);
this.setState({ loading: true });
}
componentDidMount() {
//this._loadInitialState().done();
this.createViewGroup();
}
createViewGroup = async () => {
try {
const response = await fetch(
'/Dsenze/userapi/sensor/viewsensor',
{
method: 'POST',
headers: {
Accept: 'application/json',
'Content-Type': 'application/json',
},
body: JSON.stringify({
"password": 'admin',
"username": 'admin',
"startlimit": "0",
"valuelimit": "10",
}),
}
);
const responseJson = await response.json();
const { sensorData } = responseJson;
this.setState({
AbcSdata: sensorData,
loading: false,
});
} catch (e) {
console.error(e);
}
};
updateSearch = search => {
this.setState({ search });
};
keyExtractor = ({ id }) => id.toString();
keyExtractor = ({ inventory }) => inventory.toString();
onSwipeOpen(rowId, direction) {
if(typeof direction !== 'undefined'){
this.setState({activeRow:rowId});
console.log("Active Row",rowId);
}
}
renderItem = ({ item, index }) => (
<Swipeout
right={this.swipeBtns}
close={(this.state.activeRow !== index)}
rowID={index}
sectionId= {1}
autoClose = {true}
onOpen = {(secId, rowId, direction) => this.onSwipeOpen(rowId, direction)}
>
<TouchableOpacity
style={styles.item}
activeOpacity={0.4}
onPress={this.viewNote(item)} >
<Text >Id : {item.id}</Text>
<Text>Inventory : {item.inventory}</Text>
<Text>SensorType : {item.sensorType}</Text>
<Text>TypeName : {item.typeName}</Text>
</TouchableOpacity>
</Swipeout>
);
viewNote =(item) => {
this.props.navigator.push({
title: 'The Note',
component: this.ViewNote,
passProps: {
noteText: item,
noteId: this.noteId(item),
}
});
console.log("View Note Success");
}
onClickListener = (viewId) => {
if (viewId == 'tag') {
this.props.navigation.navigate('AddSensors');
}
}
renderSeparator = () => {
return (
<View
style={{
height: 1,
width: "86%",
backgroundColor: "#CED0CE",
}}
/>
);
};
render() {
const { loading, AbcSdata } = this.state;
const state = this.state;
return (
<ScrollView>
<View style={styles.container1}>
<Button full rounded
style={{ fontSize: 20, color: 'green' }}
styleDisabled={{ color: 'red' }}
onPress={() => this.onClickListener('tag')}
title="Add Sensors"
>
Add Sensors
</Button>
</View>
<View style={styles.container1}>
{this.state.loading ? (
<ActivityIndicator size="large" />
) :
(
<FlatList
data={AbcSdata}
renderItem={this.renderItem}
keyExtractor={this.keyExtractor}
extraData= {this.state.activeRow}
ItemSeparatorComponent={this.renderSeparator}
/>
)}
</View>
<View>
<Text
style={{ alignSelf: 'center', fontWeight: 'bold', color: 'black' }} >
Inventory Details
</Text>
<Table borderStyle={{ borderWidth: 2, borderColor: '#c8e1ff', padding: 10, paddingBottom: 10 }}>
<Rows data={state.tableData} textStyle={styles.text} />
</Table>
</View>
</ScrollView>
);
}
}
const styles = StyleSheet.create({
container: {
flex: 1,
justifyContent: 'center',
paddingTop: Constants.statusBarHeight,
backgroundColor: '#ecf0f1',
padding: 8,
},
paragraph: {
margin: 24,
fontSize: 18,
fontWeight: 'bold',
textAlign: 'center',
},
});
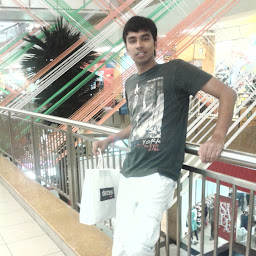
Author by
Abhigyan Gaurav
Updated on June 04, 2022Comments
-
Abhigyan Gaurav almost 2 years
// Here I am trying to delete List item from Flat List . Data is populating from JSON on Flat List now I have to delete particular list item data form list , for that I am using "Swipeout" . but getting error "Type error: undefined is not an object(evaluting this.2.viewNote.bind")
Please help . Where am going wrong and how can I do thisimport React, { Component } from 'react'; import { View, Text, TextInput, FooterTab,Button,TouchableOpacity, ScrollView, StyleSheet, ActivityIndicator ,Header,FlatList} from 'react-native'; import {Icon} from 'native-base'; import { Table, Row, Rows } from 'react-native-table-component'; import { createStackNavigator } from 'react-navigation'; import { SearchBar } from 'react-native-elements'; import Swipeout from 'react-native-swipeout'; let swipeBtns = [{ text: 'Delete', backgroundColor: 'red', underlayColor: 'rgba(0, 0, 0, 1, 0.6)', onPress: () => { this.deleteNote(item) } }]; export default class OpenApplianceIssue extends Component { constructor() { super(); this.state = { AbcSdata: null, loading: true, search: '', tableData: [], qrData: '', selectedPriority: '', selectedIssue: '', selectedReason: '', selectedTriedRestart: '', selectedPowerLED: '', selectedBurning: '', selectedNoise: '', }; this.setDate = this.setDate.bind(this); } setDate(newDate) { } _loadInitialState = async () => { const { navigation } = this.props; const qdata = navigation.getParam('data', 'NA').split(','); var len = qdata.length; const tData = []; console.log(len); for(let i=0; i<len; i++) { var data = qdata[i].split(':'); const entry = [] entry.push(`${data[0]}`); entry.push(`${data[1]}`); tData.push(entry); } this.setState({tableData: tData } ); console.log(this.state.tableData); this.setState({loading: true}); } componentDidMount() { this._loadInitialState().done(); this.createViewGroup(); } createViewGroup = async () => { try { const response = await fetch( 'http:/Dsenze/userapi/sensor/viewsensor', { method: 'POST', headers: { Accept: 'application/json', 'Content-Type': 'application/json', }, body: JSON.stringify({ "password": 'admin', "username": 'admin', "startlimit":"0", "valuelimit":"10", }), } ); const responseJson = await response.json(); const { sensorData } = responseJson; this.setState({ AbcSdata: sensorData, loading: false, }); } catch (e) { console.error(e); } }; updateSearch = search => { this.setState({ search }); }; keyExtractor = ({ id }) => id.toString(); keyExtractor = ({ inventory }) => inventory.toString(); renderItem = ({ item }) => ( <Swipeout right={swipeBtns}> <TouchableOpacity style={styles.item} activeOpacity={0.4} onPress={this.viewNote.bind(this, item)} > <Text >Id : {item.id}</Text> <Text>Inventory : {item.inventory}</Text> <Text>SensorType : {item.sensorType}</Text> <Text>TypeName : {item.typeName}</Text> </TouchableOpacity> </Swipeout> ); viewNote(item) { this.props.navigator.push({ title: 'The Note', component: ViewNote, passProps: { noteText: item, noteId: this.noteId(item), } }); } onClickListener = (viewId) => { if(viewId == 'tag') { this.props.navigation.navigate('AddSensors'); }} renderSeparator = () => { return ( <View style={{ height: 1, width: "86%", backgroundColor: "#CED0CE", }} /> ); }; render() { const { loading, AbcSdata } = this.state; const state = this.state; return ( <ScrollView> <View style={styles.container1}> <Button full rounded style={{fontSize: 20, color: 'green'}} styleDisabled={{color: 'red'}} onPress={() => this.onClickListener('tag')} title="Add Sensors" > Add Sensors </Button> </View> <View style={styles.container1}> {this.state.loading ? ( <ActivityIndicator size="large" /> ) : ( <FlatList data={AbcSdata} renderItem={this.renderItem} keyExtractor={this.keyExtractor} ItemSeparatorComponent={this.renderSeparator} /> )} </View> <View> <Text style={{alignSelf: 'center', fontWeight: 'bold', color: 'black'}} > Inventory Details </Text> <Table borderStyle={{borderWidth: 2, borderColor: '#c8e1ff', padding:10,paddingBottom: 10}}> <Rows data={state.tableData} textStyle={styles.text}/> </Table> </View> </ScrollView> ); } } const styles = StyleSheet.create( { container1: { flex: 1, alignItems: 'stretch', fontFamily: "vincHand", color: 'blue' }, header_footer_style:{ width: '100%', height: 44, backgroundColor: '#4169E1', alignItems: 'center', justifyContent: 'center', color:'#ffffff', }, Progressbar:{ justifyContent: 'center', alignItems: 'center', color: 'blue', }, ListContainer :{ borderColor: '#48BBEC', backgroundColor: '#000000', color:'red', alignSelf: 'stretch' , }, container2: { flex: 1, justifyContent: 'center', alignItems: 'stretch', paddingHorizontal: 15 }, inputBox:{ width:300, borderColor: '#48BBEC', backgroundColor: '#F8F8FF', borderRadius:25, paddingHorizontal:16, fontSize:16, color:'#000000', marginVertical:10, }, button:{ width:300, backgroundColor:'#4169E1', borderRadius:25, marginVertical:10, paddingVertical:16 }, buttonText:{ fontSize:16, fontWeight:'500', color:'#ffffff', textAlign:'center' }, textStyle:{ fontSize:16, fontWeight:'500', color:'#ffffff', textAlign:'center' }, item: { padding: 15 }, text: { fontSize: 18 }, button:{ width:300, backgroundColor:'#4169E1', borderRadius:25, marginVertical:10, paddingVertical:16 }, buttonText:{ fontSize:16, fontWeight:'500', color:'red', textAlign:'center' }, separator: { height: 2, backgroundColor: 'rgba(0,0,0,0.5)' }, container: { flex: 1, padding: 16, paddingTop: 30, backgroundColor: '#fff' }, head: { height: 40, backgroundColor: '#f1f8ff' }, text: { margin: 6 } }); Thanks .
-
Abhigyan Gaurav about 5 yearswhy ? import AssetExample from './components/AssetExample this?
-
Abhigyan Gaurav about 5 yearsand if I have to delete data from server then I need to call api on delete press, rit?
-
Nakul about 5 yearsYes you need to call delete API. Sorry for the late reply, i was out.
-
Abhigyan Gaurav about 5 yearsgetting erroe undefined is not function this.props.navigator.push
-
Nakul about 5 yearsHave you configured the React navigation correctly ? wix.github.io/react-native-navigation/#/docs/Usage
-
Abhigyan Gaurav about 5 yearsLet us continue this discussion in chat.
-
Abhigyan Gaurav about 5 yearsYes , but error, one request could you please run in your machinie