Delphi Tokyo - Function: String to Hex and Hex to String
There are a couple of problems with your code:
You are type-casting your input
AnsiString
incorrectly toPWideChar
, so you are calling the wrong overload ofHexToBin()
.PWideChar
should bePAnsiChar
instead.The
BufSize
parameter ofHexToBin()
specifies the number of bytes the output buffer expects to receive, but you are passing it the number of characters in the hex string instead.
Also, since String2Hex()
takes an AnsiString
and returns a UnicodeString
, Hex2String()
should take UnicodeString
and return an AnsiString
to match.
Try this instead:
function String2Hex(const Buffer: AnsiString): string;
begin
SetLength(Result, Length(Buffer) * 2);
BinToHex(PAnsiChar(Buffer), PChar(Result), Length(Buffer));
end;
function Hex2String(const Buffer: string): AnsiString;
begin
SetLength(Result, Length(Buffer) div 2);
HexToBin(PChar(Buffer), PAnsiChar(Result), Length(Result));
end;
var
hex: string;
str: AnsiString;
begin
hex := String2Hex('stackoverflow');
ShowMessage(hex); // shows '737461636B6F766572666C6F77'
str := Hex2String(hex);
ShowMessage(str); // shows 'stackoverflow'
end;
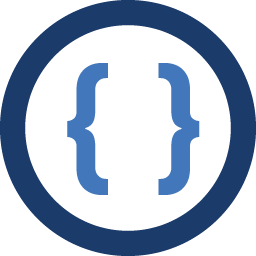
Admin
Updated on June 04, 2022Comments
-
Admin almost 2 years
The function below works perfectly to convert string to hexadecimal:
function String2Hex(const Buffer: AnsiString): string; begin SetLength(Result, Length(Buffer) * 2); BinToHex(@Buffer[1], PWideChar(@Result[1]), Length(Buffer)); end; ShowMessage(String2Hex('stackoverflow'));
This result: "737461636B6F766572666C6F77"
The problem is in the function of converting hexadecimal to string:
function Hex2String(const Buffer: AnsiString): string; begin SetLength(Result, Length(Buffer) div 2); HexToBin(PWideChar(@Buffer[1]), @Result[1], Length(Buffer)); end; ShowMessage(Hex2String('737461636B6F766572666C6F77'));
The result should be "stackoverflow" but nothing happens.
Could someone help me?
-
Sertac Akyuz over 6 yearsPoint '3' is an error in documentation. HexToBin accounts for uppercase letters since at least D7 (older version I can check is D3, which doesn't have the function at all).
-
Remy Lebeau over 6 years@SertacAkyuz: you are right, I misread the code when I checked it before posting. FYI, the support of uppercase goes all the way back to at least Delphi 5, if not 4 (but it didn't ignore non-hex characters
#58..#64,#91..#96
until Delphi 2009). -
Remy Lebeau over 6 years@SertacAkyuz: I reported the documentation error: RSP-19625
-
peiman F. about 5 yearsfiremonkey in android dont have
PAnsiChar
andAnsiString
-
Remy Lebeau about 5 years@peimanF. So just use
String
andPChar
instead. You don't needAnsiString
support to use hex strings.Bin2Hex()
andHex2Bin()
are overloaded for Unicode strings. Otherwise, install Andreas Hausladen's ByteStrings patch.