Deserializing JSON object into a C# list
58,938
So I think you're probably trying to deserialize to the wrong type. If you serialized it to RootObject and try to deserialize to List it's going to fail.
See this example code
public void TestMethod1()
{
var items = new List<Item>
{
new Item { Att1 = "ABC", Att2 = "123" },
new Item { Att1 = "EFG", Att2 = "456" },
new Item { Att1 = "HIJ", Att2 = "789" }
};
var root = new Root() { Items = items };
var itemsSerialized = JsonConvert.SerializeObject(items);
var rootSerialized = JsonConvert.SerializeObject(root);
//This works
var deserializedItemsFromItems = JsonConvert.DeserializeObject<List<Item>>(itemsSerialized);
//This works
var deserializedRootFromRoot = JsonConvert.DeserializeObject<Root>(rootSerialized);
//This will fail. YOu serialized it as root and tried to deserialize as List<Item>
var deserializedItemsFromRoot = JsonConvert.DeserializeObject<List<Item>>(rootSerialized);
//This will fail also for the same reason
var deserializedRootFromItems = JsonConvert.DeserializeObject<Root>(itemsSerialized);
}
class Root
{
public IEnumerable<Item> Items { get; set; }
}
class Item
{
public string Att1 { get; set; }
public string Att2 { get; set; }
}
Edit: Added complete code.
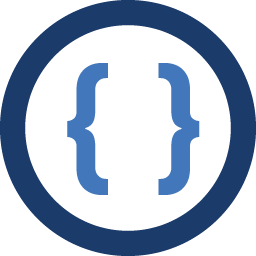
Author by
Admin
Updated on December 16, 2020Comments
-
Admin over 3 years
I'm trying to deserialize a given JSON file in C# using a tutorial by Bill Reiss. For XML data in a non-list this method works pretty well, though I would like to deserialize a JSON file with the following structure:
public class Data { public string Att1 { get; set; } public string Att2 { get; set; } public string Att3 { get; set; } public string Att4 { get; set; } } public class RootObject { public List<Data> Listname { get; set; } }
My problem is with using JSON.Net's ability to create / put data into lists, and then displaying the list on an XAML page. My idea so far (which is not working):
var resp = await client.DoRequestJsonAsync<DATACLASS>("URL"); string t = resp.ToString(); var _result = Newtonsoft.Json.JsonConvert.DeserializeObject<List<DATACLASS>>(t); XAMLELEMENT.ItemsSource = _result;
-
Admin about 11 yearsI tried your code. I added the following lines in order to Display it. Do you see the mistake (it's not working. No Error just no change)? string t = deserializedItemsFromItems.ToString(); PhoneList.ItemsSource = t; string v = deserializedRootFromRoot.ToString(); PhoneList.ItemsSource = v;
-
cgotberg about 11 yearsThe code above wouldn't work out the the box as soon as it hits var deserializedItemsFromRoot = JsonConvert.DeserializeObject<List<Item>>(rootSerialized); it's going to throw an exception.
-
cgotberg about 11 yearsAre you having a problem with the deserialization or assigning ItemsSource? Assuming you get an deserialized object back then something.ItemsSource = Root.Items.ToList(); should work.
-
Admin about 11 yearsThank you so much cgotberg. It's finally working! Your "ToList" - Hint made it.