Design Patterns: Abstract Factory vs Factory Method
Solution 1
The two patterns are certainly related!
The difference between patterns is generally in intent.
The intent of Factory Method is "Define an interface for creating an object, but let subclasses decide which class to instantiate. Factory Method lets a class defer instantiation to subclasses."
The intent of Abstract Factory is "Provide an interface for creating families of related or dependent objects without specifying their concrete classes."
Based purely on these intent statements (quoted from GoF), I would say that indeed Factory Method is in some sense a "degenerate" Abstract Factory with a family of one.
They generally tend to differ in implementation, as Factory Method is a good deal simpler than Abstract Factory.
They are related also in implementation however. As noted in the GoF book,
AbstractFactory only declares an interface for creating products. It's up to ConcreteProduct subclasses to actually create them. The most common way to do this is to define a factory method for each product.
This c2 wiki also has some interesting discussion on this topic.
Solution 2
It seems that the OP's list of (excellent) questions has been ignored. Current answers merely offer rehashed definitions. So I will attempt to address the original questions concisely.
- If the Abstract Factory has only one creator and one product, is it still the Abstract Factory pattern? (an interface for creating familes)
No. An Abstract Factory must create more than one product to make a "family of related products". The canonical GoF example creates ScrollBar()
and Window()
. The advantage (and purpose) is that the Abstract Factory can enforce a common theme across its multiple products.
- Can the Factory Method concrete creator be created from an Interface or does it have to be from a class? (classes defer instantiations to subclasses)
First, we must note that neither Java nor C# existed when the GoF wrote their book. The GoF use of the term interface is unrelated to the interface types introduced by particular languages. Therefore, the concrete creator can be created from any API. The important point in the pattern is that the API consumes its own Factory Method, so an interface with only one method cannot be a Factory Method any more than it can be an Abstract Factory.
- If the Abstract Factory can have only one creator and one product, is the only difference between the Abstract Factory and the Factory Method that the creator for the former is an Interface and the creator for the latter is a Class?
This question is no longer valid, following the answers above; however, if you are left thinking that the only difference between Abstract Factory and Factory Method is the number of products created, consider how a client consumes each of these patterns. An Abstract Factory is typically injected into its client and invoked via composition/delegation. A Factory Method must be inherited. So it all comes back to the old composition vs. inheritance debate.
But these answers have raised a fourth question!
- Since, an interface with only one method cannot be a Factory Method any more than it can be an Abstract Factory, what do we call a creational interface with only one method?
If the method is static, it is commonly called a Static Factory. If the method is non-static, it is commonly called a Simple Factory. Neither of these is a GoF pattern, but in practice they are far more commonly used!
Solution 3
In my opinion, the slight difference between the two patterns resides in the applicability, and so, as already said, in the Intent.
Let's recap the definitions (both from Wikipedia).
Abstract Factory
Provide an interface for creating families of related or dependent objects without specifying their concrete classes.
Factory Method
Define an interface for creating an object, but let the classes that implement the interface decide which class to instantiate. The Factory method lets a class defer instantiation to subclasses.
Both patterns allow to decouple the user objects from creating needed instances (run-time decoupling), and this is the common aspect. Both patterns allow to create a hierarchy of factories according to any specific needs, and this is another common aspect.
Abstract Factory allows to create several different type of instances in one sub-class, and to particularize the creations behavior in its different sub-classes; normally, Factory method declares the creation of only one type of object that can be particularized according to the sub-classing mechanism. That's the difference.
By summarizing. Let's say that Product defines the super-class of the creating objects, and that ProductA and ProductB are two different sub-classes. Therefore, the Abstract Factory method will have two methods, createProductA() and createProductB() which will be particularized (in terms of creation steps) in its specific sub-classes: the factory sub-classes particularize the creation steps for the two defined classes of objects under creation.
According to the above example, the Factory Method will be implemented differently, abstracting the creation of ProductA and ProductB in as many factories (one method per Factory), and the further specialization of the creation steps will be delegated to the hierarchy as it is built.
Solution 4
If I created an abstracted (referenced via an interface or abstract base class) Factory Class that creates objects that has only one method to create objects, then it would be a Factory Method.
If the abstracted Factory had more than 1 method to create objects, then it would be an Abstract Factory.
Let's say I make a Manager that will handle the needs of action methods for an MVC controller. If it had one method, say to create the engine objects that will be used to create view models, then it would be an factory method pattern. On the other hand if it had two methods: one to create view model engines, and another to create action model engines (or whatever you want to call the model that the action method contains consumers), then it would be an abstract factory.
public ActionResult DoSomething(SpecificActionModel model)
{
var actionModelEngine = manager.GetActionModelEngine<SpecificActionModel>();
actionModelEngine.Execute(SpecificActionModelEnum.Value);
var viewModelEngine = manager.GetViewModelEngine<SpecificViewModel>();
return View(viewModelEngine.GetViewModel(SpecificViewModelEnum.Value);
}
Solution 5
All you have to remember is that a abstract factory is a factory that can return a multiple factories. So if you had a AnimalSpeciesFactory it can return factories like this:
Mamalfactory, BirdFactory,Fishfactory,ReptileFactory. Now that you have a single factory from the AnimalSpeciesFactory, they use the factory pattern to create specific objexts. For example, imagine you got a ReptileFactory from this AnimalFactory, then you could offer to create reptile objects like: Snakes,turtles,lizards objects.
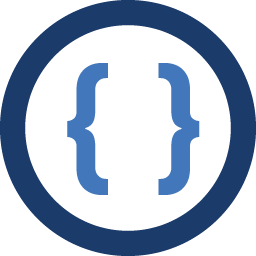
Admin
Updated on September 30, 2021Comments
-
Admin over 2 years
Note: Questions are at the end of the post.
I have read the other stackoverflow threads regarding Abstract Factory vs Factory Method. I understand the intent of each pattern. However, I am not clear on the definition.
Factory Method defines an interface for creating an object, but lets subclasses decide which of those to instantiate. A factory method lets classes defer instantiation to subclasses.
By contrast, an Abstract Factory provides an interface for creating families of related or dependent objects without specifying their concrete classes.
The Abstract Factory looks very similar to the Factory Method. I have drawn a few UML classes to illustrate my point.
Note:
- The diagram are from www.yuml.com so they are not perfectly oriented. But its a free service :).
- The diagrams may not be perfect. I am still learning the GoF design patterns.
Factory Method:
Abstract Factory (only 1 member):
Abstract Factory (more members):
Questions:
- If the Abstract Factory has only one creator and one product, is it still the Abstract Factory pattern? (an interface for creating familes)
- Can the Factory Method concrete creator be created from an Interface or does it have to be from a class? (classes defer instantiations to subclasses)
- If the Abstract Factory can have only one creator and one product, is the only difference between the Abstract Factory and the Factory Method that the creator for the former is an Interface and the creator for the latter is a Class?
-
Don Roby over 11 yearsI understand neither the comment nor the downvote. Can you elaborate?
-
Novalis over 11 yearsWell,the answers seems to me rhetorical...No real concreate example...overly broad...
-
georaldc almost 7 yearsAbout composition vs inheritance, I always wondered: isn't it possible to also do composition with the Factory Method pattern? What would prevent one from composing or injecting the right concrete factory into a client? Or is this something outside the pattern's scope already?
-
jaco0646 almost 7 years@georaldc, from the GoF (page 107) "Factory Method lets a class defer instantiation to subclasses." In other words, Factory Method uses inheritance by definition.