Detect button click from WebView in React Native
Solution 1
Add window.postMessage()
to your page file and onMessage={this.onMessage}
to your React Native WebView component.
Example:
// index.html (Web)
...
<script>
window.postMessage("Sending data from WebView");
</script>
...
// MyWebView.js (React Native)
<WebView>
...
<WebView
ref="webview"
onMessage={this.onMessage}
/>
onMessage(data) {
//Prints out data that was passed.
console.log(data);
}
Edit: Working example:
App.js
onMessage(m) {
alert(m.nativeEvent.data);
}
render() {
return(
<WebView
style={{ flex: 1 }}
source={{uri: 'http://127.0.0.1:8877'}} //my localhost
onMessage={m => this.onMessage(m)}
/>
);
}
index.html
<button>Send post message from web</button>
<script>
document.querySelector("button").onclick = function() {
console.log("Send post message");
window.postMessage("Hello React", "*");
}
</script>
Hope it helps
Solution 2
window.postMessage
doesn't work for me for current last version. And found many other solutions that maybe worked for someone in some version but not for me.
Check how I solve it with window.ReactNativeWebView.postMessage
:
"react": "16.13.1",
"react-native": "0.63.3",
"react-native-webview": "^11.0.2",
Note: that I am using html string instead of url
<WebView
bounces={false}
showsHorizontalScrollIndicator={false}
showsVerticalScrollIndicator={false}
javaScriptEnabled={true}
originWhitelist={['*']}
source={{ html }}
onLoadEnd={this.onLoadEnd}
onError={this.onError}
mixedContentMode={'never'} // security
startInLoadingState={true} // when starting this component
onMessage={onMessage}
javaScriptEnabledAndroid={true}
/>
onMessage = (message: string) => {
console.log('action result coming from the webview: ', message);
};
And in the html
string I added the script below and set the onclick
for a given html button
or a
tag:
...
<div>
<button onclick="clickYourButton([PASS SOME STRING DATA HERE])">Click</button>
</div>
...
<script type="text/javascript">
function clickYourButton(data) {
setTimeout(function () {
window.ReactNativeWebView.postMessage(data)
}, 0) // *** here ***
}
</script>
- Data to be passed to the
postMessage
func should bestring
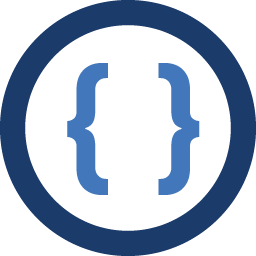
Admin
Updated on June 09, 2022Comments
-
Admin almost 2 years
Can anyone please help me on how to detect Webview button click event in React Native? As shown in the code below, I have a Button in my WebView (index.html) for which i want to detect click event from React Native and execute the method onClick. I have tried multiple ways, but couldn't succeed. Thanks so much in Advance.
my MyApp.js
import React from 'react'; import { AppRegistry, View, WebView, StyleSheet} from 'react-native' export default class MyApp extends React.Component { onClick = () => { console.log('Button Clicked'); } render() { return ( <View style={styles.container}> <WebView style={styles.webView} source={require('./index.html')}/> </View> ); } }; let styles = StyleSheet.create({ container: { flex: 1, backgroundColor: '#fff', }, webView: { backgroundColor: '#fff', height: 350, } });
my index.html
<html> <body> <Button>Click Here</Button> </body> </html>