detecting a file downloaded in selenium java
Solution 1
I had the same question and here is what I found somewhere on the Internet (maybe on stackoverflow, I cannot remember). I just added a line to delete the file so that by calling this method at the beginning of my test, I'm making sure the file does not exist anymore when trying to download it again.
public boolean isFileDownloaded(String downloadPath, String fileName) {
File dir = new File(downloadPath);
File[] dirContents = dir.listFiles();
for (int i = 0; i < dirContents.length; i++) {
if (dirContents[i].getName().equals(fileName)) {
// File has been found, it can now be deleted:
dirContents[i].delete();
return true;
}
}
return false;
}
You just have to call with this single line: isFileDownloaded("C:\Path\To\Your\Folder", "yourPdfFile.abc");
Hope this helps!
Solution 2
My solution in the end was to count the files in the download directory before and after i open the page.
I'll be glad to know if someone knows a way to find the trigger for the download
Solution 3
I am handling a similar condition in my automation.
Step1: set the download path in chrome using chrome preference
ChromeOptions options = new ChromeOptions();
HashMap<String, Object> chromePref = new HashMap<>();
chromePref.put("download.default_directory", <Directory to download file>);
options.setExperimentalOption("prefs", chromePref);
Make sure that there is no file with the expected file name in the folder where you are downloading.
Step 2: navigate to the url in chrome, the file will be automatically downloaded to the specified folder.
Step 3: check the file existence in the downloaded folder.
Solution 4
This method is working for me successfully
/**
* This method will wait until the folder is having any downloads
* @throws InterruptedException
*/
public static void waitUntilFileToDownload(String folderLocation) throws InterruptedException {
File directory = new File(folderLocation);
boolean downloadinFilePresence = false;
File[] filesList =null;
LOOP:
while(true) {
filesList = directory.listFiles();
for (File file : filesList) {
downloadinFilePresence = file.getName().contains(".crdownload");
}
if(downloadinFilePresence) {
for(;downloadinFilePresence;) {
sleep(5);
continue LOOP;
}
}else {
break;
}
}
}
Solution 5
This is working for me perfectly:
public static void waitForTheExcelFileToDownload(String fileName, int timeWait)
throws IOException, InterruptedException {
String downloadPath = getSystemDownloadPath();
File dir = new File(downloadPath);
File[] dirContents = dir.listFiles();
for (int i = 0; i < 3; i++) {
if (dirContents[i].getName().equalsIgnoreCase(fileName)) {
break;
}else {
Thread.sleep(timeWait);
}
}
}
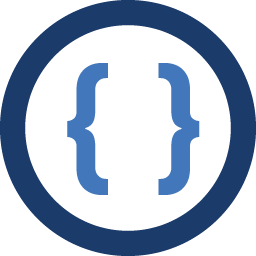
Admin
Updated on January 12, 2021Comments
-
Admin over 3 years
I wrote an automation test in selenium java that detects if the page is redirecting (the automation detects if a new page is opened, the page redirects to other page, a new tab is opened and if an alert window is opened)
Now to the problem. one of the redirects i can't find any way to detect is an automatic downloaded file (you enter a website and the website automatically downloads a file without any trigger from the user)
p.s.
I know the download process may differ in each browser, I need it to work mainly on chrome
Thanks