Detecting ctrl+v on key press
Solution 1
If you need to handle both, can be done using the similar approach
Have a look at Key Codes. You can detect what ever the key been pressed
so..
Create a list containing all the inputs you need
public List<int> KeyCodes = new List<int>() { 8, 17, 37, 39.....etc};
and use the KeyDown
event and use KeyEventArgs.SuppressKeyPress property
private void Txt1_KeyDown(object sender, KeyEventArgs e)
{
if (KeyCodes.Contains(e.KeyValue) || (e.KeyCode==Keys.V && e.Control))
e.SuppressKeyPress = false;
else
e.SuppressKeyPress=true;
}
You might need to validate the copy pasted value in leave event
, since user can paste anything!!!
Solution 2
Just look at the Modifiers propertie in you KeyDown
or KeyUp
event for Keys.Control
Modifiers Gets the modifier flags for a KeyDown or KeyUp event. The flags indicate which combination of CTRL, SHIFT, and ALT keys was pressed.
Sample
KeyDown
this.myTextBox.KeyDown += new KeyEventHandler(myTextBox_KeyDown);
private void myTextBox_KeyDown(object sender, KeyEventArgs e)
{
if (e.KeyCode == Keys.V && e.Modifiers == Keys.Control)
{
MessageBox.Show("Hello world");
}
}
or KeyUp
this.myTextBox.KeyUp += new KeyEventHandler(myTextBox_KeyUp);
private void myTextBox_KeyUp(object sender, KeyEventArgs e)
{
if (e.KeyCode == Keys.V && e.Modifiers == Keys.Control)
{
MessageBox.Show("Hello world");
}
}
Update
To allow only certain inputs you can use the KeyPress
event.
this.myTextBox.KeyPress += new KeyPressEventHandler(this.myTextBox_KeyPress);
private void myTextBox_KeyPress(object sender, KeyPressEventArgs e)
{
int i;
if (int.TryParse(e.KeyChar.ToString(), out i) ||
e.KeyChar == '(' ||
e.KeyChar == ')' ||
e.KeyChar == '.' ||
e.KeyChar == '*' ||
e.KeyChar == '+' ||
e.KeyChar == '-' ||
e.KeyChar == '/'
// add some more)
{
} else e.Handled = true; // dont allow other inputs
}
More Information
Solution 3
KeyPress
event doesn't catch "Ctrl + V" for some reason. Use KeyDown
event:
private void textBox1_KeyDown(object sender, KeyEventArgs e)
{
if (e.Control && e.KeyCode == Keys.V)
{
//Ctrl + V
}
}
or
private void textBox1_KeyDown(object sender, KeyEventArgs e)
{
if (e.KeyData == (Keys.Control | Keys.V))
{
//Ctrl + V
}
}
Solution 4
The Ctrl+V sequence can be catched by KeyPressEvent.
You just cannot check it by the following :
(Keys)e.KeyChar == Keys.V && Control.ModifierKeys == Keys.Control <br>
You must use:
(int)e.KeyChar==22 && Control.ModifierKeys == Keys.Control <br>
because the keybord generates the ASCII=22 (synchronous idle).
The following example enables TextBox to accept +- amounts with comma separator and allows pasting by Ctrl+V
protected override void OnKeyPress(KeyPressEventArgs e)
{
if (!( char.IsNumber(e.KeyChar) ||
((Keys)e.KeyChar == Keys.Back) ||
(e.KeyChar == ',') ||
(e.KeyChar == '-') ||
((int)e.KeyChar==22 && Control.ModifierKeys == Keys.Control)
))
{
e.Handled = true;
}
if (e.KeyChar == ',' && base.Text.IndexOf(',') > 0)
{
e.Handled = true;
}
base.OnKeyPress(e);
}
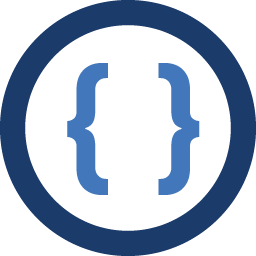
Admin
Updated on July 20, 2022Comments
-
Admin almost 2 years
I am using text box
keypress
event to handle only selected inputs. Basically thetextbox
allow user to input values where it can be calculated.i.e. you can type
(5*5)- (10/5)
.The current method check like
Convert.ToChar("*")==e.KeyChar
etc...At the moment it doesn't allow user to copy paste values.
Is there anyway that can detect the
ctrl+v
onkeypress
event?Update
What I am doing at the moment
static IEnumerable<char> ValidFiancialCharacters { get { if(_validFiancialCharacters==null) { _validFiancialCharacters = new List<char>(); _validFiancialCharacters.Add(Convert.ToChar("0")); _validFiancialCharacters.Add(Convert.ToChar("1")); _validFiancialCharacters.Add(Convert.ToChar("2")); // till 9 and _validFiancialCharacters.Add(Convert.ToChar("+")); _validFiancialCharacters.Add(Convert.ToChar("-")); _validFiancialCharacters.Add(Convert.ToChar("/")); //and some other } return _validFiancialCharacters; } } public static bool ValidateInput(KeyPressEventArgs e) { if (ValidFiancialCharacters.Any(chr => chr == e.KeyChar)) { e.Handled = false; } else { e.Handled = true; } return e.Handled; }
And in the keypress
private void txtRate_KeyPress(object sender, KeyPressEventArgs e) { NumberExtension.ValidateInput(e); }