Detecting if a specific sprite was touched on Cocos2d-iphone
Solution 1
That looks like a very difficult way to go about doing it. I havent been coding long myself but maybe the following might help you. lets say u have a nsmutablearray called enemies and you add the new enemy object to this array when ever you create one. enemy object would be a ccnode and have a ccsprite within it called _enemySprite then do the touch
-(void)ccTouchesBegan:(NSSet *)touches withEvent:(UIEvent *)event
{
NSSet *allTouches = [event allTouches];
UITouch * touch = [[allTouches allObjects] objectAtIndex:0];
//UITouch* touch = [touches anyObject];
CGPoint location = [touch locationInView: [touch view]];
location = [[CCDirector sharedDirector] convertToGL:location];
int arraysize = [enemies count];
for (int i = 0; i < arraysize; i++) {
if (CGRectContainsPoint( [[[enemies objectAtIndex:i] _enemySprite] boundingBox], location)) {
//some code to destroy ur enemy here
}
}
// NSLog(@"TOUCH DOWN");
}
hope this helps
Solution 2
Another way of doing it is that calculating distance between touch position and your sprites.. If touch is close enough to one of your sprites, you can kill it.. Something like this..
for (CCSprite *sprite in anArrayThatCOntainsAllYourSprites) {
float distance = pow(sprite.position.x - location.x, 2) + pow(sprite.position.y - location.y, 2);
distance = sqrt(distance);
if (distance <= 10) {
sprite.dead = YES;
}
}
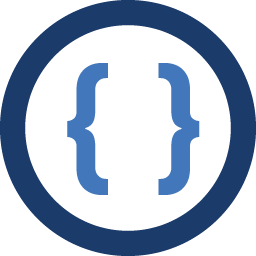
Admin
Updated on June 04, 2022Comments
-
Admin almost 2 years
I was following Ray`s tutorial for making a simple iPhone game (here: http://goo.gl/fwPi) , and decided that i wanted the enemies to be eliminated when they get touched.
My initial approach was to spawn a small CCSprite sprite on the touch location, then use CGRectMake to create a bounding box of said sprite to detect if the enemy sprite was touched. Much like Ray does with the projectile/enemy. But of course, my way of doing it isnt working and i cant dig myself out of this hole.
Here is the relevant code snippet. Any help is appreciated:
- (void)ccTouchesEnded:(NSSet *)touches withEvent:(UIEvent *)event { // Choose one of the touches to work with UITouch *touch = [touches anyObject]; CGPoint location = [self convertTouchToNodeSpace: touch]; location = [[CCDirector sharedDirector] convertToGL:location]; CCSprite *touchedarea = [CCSprite spriteWithFile:@"Icon-72.png" rect:CGRectMake(location.x, location.y, 2, 2)]; touchedarea.tag = 2; [self addChild:touchedarea]; [_touchedareas addObject:touchedarea]; } - (void)update:(ccTime)dt { NSMutableArray *touchedareasToDelete = [[NSMutableArray alloc] init]; for (CCSprite *touchedarea in _touchedareas) { CGRect touchedareaRect = CGRectMake( touchedarea.position.x, touchedarea.position.y, touchedarea.contentSize.width, touchedarea.contentSize.height); NSMutableArray *targetsToDelete = [[NSMutableArray alloc] init]; for (CCSprite *target in _targets) { CGRect targetRect = CGRectMake( target.position.x - (target.contentSize.width/2), target.position.y - (target.contentSize.height/2), target.contentSize.width, target.contentSize.height); if (CGRectIntersectsRect(touchedareaRect, targetRect)) { [targetsToDelete addObject:target]; } } for (CCSprite *target in targetsToDelete) { [_targets removeObject:target]; [self removeChild:target cleanup:YES]; } if (targetsToDelete.count > 0) { [touchedareasToDelete addObject:touchedarea]; } [targetsToDelete release]; } for (CCSprite *touchedarea in touchedareasToDelete) { [_touchedareas removeObject:touchedarea]; [self removeChild:touchedarea cleanup:YES]; } [touchedareasToDelete release]; }