Dev C++ Wininet Upload file using HTTP
Solution 1
I was able to make your code work.
First of all the code on the link you provided and the code you posted is not the same:
InternetConnect(hSession, _T("localhost"), ...
InternetConnect(hSession, _T("http://student114.110mb.com"), ...
You must pass an host name or ip address here so "localhost" is good but "http://student114.110mb.com" isn't. If you pass an URL you will get the 12005 error code [see WinINet error codes on msdn].
Another problem is the frmdata string. You should double the backslash in C:\test.txt or you will get a tab character \t in your string. The \n before and after the delimiters should also be replaced by \r\n because RFC 1521 and most other internet protocols use CRLF as a line delimiter.
Here is the string I have used.
static TCHAR frmdata[] = "-----------------------------7d82751e2bc0858\r\nContent-Disposition: form-data; name=\"uploadedfile\"; filename=\"C:\\test.txt\"\r\nContent-Type: text/plain\r\n\r\nfile contents here\r\n-----------------------------7d82751e2bc0858--\r\n";
Finally the PHP code doesn't work because you use $_FILES["file"] where you should be using $_FILES["uploadedfile"]. "uploadedfile" would typically correspond to the name of an <input type="file"> tag in HTML but in your case it is specified in the name= parameter of the frmdata[] string.
Here's the PHP code I have used to test this
move_uploaded_file($_FILES["uploadedfile"]["tmp_name"], "/files/my_file");
When you work on complex client/server interaction like this it helps to test each part separately. You could for instance.
Write a simple HTML upload form to test your php script
Have your program send its request to netcat and examine the output
Solution 2
you have to send a full request without the WinAPI if you want to have a good request for uploading a file over a php script.
In the past, I also tried to send such a request like you, with all the api functions. But when I sniffed it with wireshark, I got the same solution like you: the header is merged and it didn't work.
So I used winsock again and it worked if the data which you want to send aren't too big.
here's the code:
#include <iostream>
#include <winsock2.h>
#include <string>
using namespace std;
//link libwsock32.a
unsigned long WinsockStart()
{
WSADATA wsa;
unsigned long ulong;
struct hostent *host;
if(WSAStartup(MAKEWORD(2,2), &wsa) < 0)
{
cout << "Error WinsockStart()" << endl;
WSACleanup();
return 1;
}
if((host=gethostbyname("www.example.com"))<0)
{
cout << "Fehler gethostbyname()" << endl;
WSACleanup();
return 2;
}
ulong = *(unsigned long*) host->h_addr;
return ulong;
}
void error_exit(string text)
{
cout << text;
WSACleanup();
exit(EXIT_FAILURE);
}
int main()
{
SOCKET sock;
struct sockaddr_in addr;
unsigned long win=0;
int con = 0, gr=0, send_r=0, rec=0;
char header[2048], puffer[2018];
string to_send="hello world";
string name="test99.txt";
win=WinsockStart();
if(win==1||win==2)
error_exit("Error WinsockStart()");
addr.sin_family=AF_INET;
addr.sin_port=htons(80);
addr.sin_addr.s_addr = win;
sock = socket(AF_INET, SOCK_STREAM, 0);
if(sock<0)
error_exit("Error socket()");
gr = (to_send.size()+name.size()+287);
sprintf(header, "POST /upload.php HTTP/1.1\r\n");
sprintf(header, "%sHost: www.example.com\r\n", header);
sprintf(header, "%sConnection: Keep-Alive\r\n", header);
sprintf(header, "%sContent-Type: multipart/form-data; boundary=---------------------------90721038027008\r\n", header);
sprintf(header, "%sContent-Length: %d\r\n", header, gr);
sprintf(header, "%s\r\n", header);
sprintf(header, "%s-----------------------------90721038027008\r\n", header);
sprintf(header, "%sContent-Disposition: form-data; name=\"upfile\"; filename=\"%s\"\r\n", header, name.c_str());
sprintf(header, "%sContent-Type: text/plain\r\n", header);
sprintf(header, "%s\r\n", header);
sprintf(header, "%s%s\r\n", header, to_send.c_str());
sprintf(header, "%s-----------------------------90721038027008\r\n", header);
sprintf(header, "%sContent-Disposition: form-data; name=\"post\"\r\n", header);
sprintf(header, "%s\r\n", header);
sprintf(header, "%supload\r\n\r\n", header);
sprintf(header, "%s-----------------------------90721038027008--\r\n\r\n\0", header);
con = connect(sock, (SOCKADDR*)&addr, sizeof(addr));
if(con < 0)
error_exit("Error connect()");
if(send_r=send(sock, header, strlen(header), 0)<0)
error_exit("Error send()");
while(rec=recv(sock, puffer, 2048, 0))
{
if(rec==0)
error_exit("Server quit");
printf("%s", puffer);
}
closesocket(sock);
WSACleanup();
return EXIT_SUCCESS;
}
Until now, I've searched and I've tried for other solutions using the WinHttpAPI, but I didn't find anything for my purposes.
Patrone
P.S. Sorry for my bad english, it's not my native language
Solution 3
static TCHAR frmdata[] = "-----------------------------7d82751e2bc0858\r\nContent-Disposition: form-data; name=\"uploadedfile\"; filename=\"C:\\test.txt\"\r\nContent-Type: text/plain\r\n\r\nfile contents here\r\n-----------------------------7d82751e2bc0858--\r\n";
Last "--" characters should be before the "-----------------------------7d82751e2bc0858" boundary, not after it.
Correct code:
static TCHAR frmdata[] = "-----------------------------7d82751e2bc0858\r\nContent-Disposition: form-data; name=\"uploadedfile\"; filename=\"C:\\test.txt\"\r\nContent-Type: text/plain\r\n\r\nfile contents here\r\n-------------------------------7d82751e2bc0858\r\n";
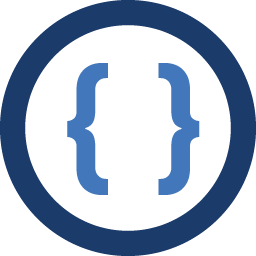
Admin
Updated on June 04, 2022Comments
-
Admin almost 2 years
I want to upload "C:\test.txt" to webserver, when I am running program, file is not uploading and I am not getting any error.
the complete C++ code can be find here
and php code on webserver can be find here: "http://student114.110mb.com/upload.txt" or "http://student114.110mb.com/upload.php"
kindly help me where I am doing wrong
#include <windows.h> #include <wininet.h> #include <tchar.h> #include <iostream> #pragma comment(lib,"wininet.lib") using namespace std; int main() { static TCHAR frmdata[] = "-----------------------------7d82751e2bc0858\nContent-Disposition: form-data; name=\"uploadedfile\"; filename=\"C:\test.txt\"\nContent-Type: text/plain\n\nfile contents here\n-----------------------------7d82751e2bc0858--"; static TCHAR hdrs[] = "Content-Type: multipart/form-data; boundary=---------------------------7d82751e2bc0858"; HINTERNET hSession = InternetOpen("MyAgent",INTERNET_OPEN_TYPE_PRECONFIG, NULL, NULL, 0); if(hSession==NULL) { cout<<"Error: InternetOpen"; } HINTERNET hConnect = InternetConnect(hSession, _T("localhost"),INTERNET_DEFAULT_HTTP_PORT, NULL, NULL, INTERNET_SERVICE_HTTP, 0, 1); if(hConnect==NULL) { cout<<"Error: InternetConnect"; } HINTERNET hRequest = HttpOpenRequest(hConnect, (const char*)"POST",_T("upload.php"), NULL, NULL, (const char**)"*/*\0", 0, 1); if(hRequest==NULL) { cout<<"Error: HttpOpenRequest"; } BOOL sent= HttpSendRequest(hRequest, hdrs, strlen(hdrs), frmdata, strlen(frmdata)); if(!sent) { cout<<"Error: HttpSendRequest"; } //close any valid internet-handles InternetCloseHandle(hSession); InternetCloseHandle(hConnect); InternetCloseHandle(hRequest); return 0; }