Difference between <context:annotation-config> and <context:component-scan>
Solution 1
I found this nice summary of which annotations are picked up by which declarations. By studying it you will find that <context:component-scan/>
recognizes a superset of annotations recognized by <context:annotation-config/>
, namely:
-
@Component
,@Service
,@Repository
,@Controller
,@Endpoint
-
@Configuration
,@Bean
,@Lazy
,@Scope
,@Order
,@Primary
,@Profile
,@DependsOn
,@Import
,@ImportResource
As you can see <context:component-scan/>
logically extends <context:annotation-config/>
with CLASSPATH component scanning and Java @Configuration features.
Solution 2
Spring allows you to do two things:
- Autowiring of beans
- Autodiscovery of beans
1. Autowiring
Usually in applicationContext.xml you define beans and other beans are wired using
constructor or setter methods. You can wire beans using XML or annotations.
In case you use annotations, you need to activate annotations and you have to add
<context:annotation-config />
in applicationContext.xml. This will simplify the
structure of the tag from applicationContext.xml, because you will not have to manually wire beans (constructor or setter). You can use @Autowire
annotation and the beans will be wired by type.
A step forward for escaping the manual XML configuration is
2. Autodiscovery
Autodiscovery is simplifying the XML one step further, in the sense that you don't even need too add the <bean>
tag in applicationContext.xml. You just mark the specific beans with one of the following annotation and Spring will automatically wire the marked beans and their dependencies into the Spring container. The annotations are as follow: @Controller, @Service, @Component, @Repository. By using <context:component-scan>
and pointing the base package, Spring will auto-discover and wire the components into Spring container.
As a conclusion:
-
<context:annotation-config />
is used in order to be able to use @Autowired annotation -
<context:component-scan />
is used to determine the search of specific beans and attempt of autowiring.
Solution 3
<context:annotation-config>
activates many different annotations in beans, whether they are defined in XML or through component scanning.
<context:component-scan>
is for defining beans without using XML
For further information, read:
Solution 4
<context:annotation-config>
: Scanning and activating annotations for already registered beans in spring config xml.
<context:component-scan>
: Bean registration + <context:annotation-config>
@Autowired and @Required are targets property level so bean should register in spring IOC before use these annotations. To enable these annotations either have to register respective beans or include <context:annotation-config />
. i.e. <context:annotation-config />
works with registered beans only.
@Required enables RequiredAnnotationBeanPostProcessor
processing tool
@Autowired enables AutowiredAnnotationBeanPostProcessor
processing tool
Note: Annotation itself nothing to do, we need a Processing Tool, which is a class underneath, responsible for the core process.
@Repository, @Service and @Controller are @Component, and they targets class level.
<context:component-scan>
it scans the package and find and register the beans, and it includes the work done by <context:annotation-config />
.
Solution 5
The difference between the two is really simple!.
<context:annotation-config />
Enables you to use annotations that are restricted to wiring up properties and constructors only of beans!.
Where as
<context:component-scan base-package="org.package"/>
Enables everything that <context:annotation-config />
can do, with addition of using stereotypes eg.. @Component
, @Service
, @Repository
. So you can wire entire beans and not just restricted to constructors or properties!.
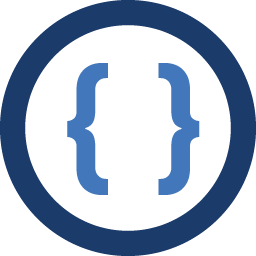
Admin
Updated on July 07, 2021Comments
-
Admin almost 3 years
I'm learning Spring 3 and I don't seem to grasp the functionality behind
<context:annotation-config>
and<context:component-scan>
.From what I've read they seem to handle different annotations (
@Required
,@Autowired
etc vs@Component
,@Repository
,@Service
etc), but also from what I've read they register the same bean post processor classes.To confuse me even more, there is an
annotation-config
attribute on<context:component-scan>
.Can someone shed some light on these tags? What's similar, what's different, is one superseded by the other, they complete each other, do I need one of them, both?
-
Admin over 12 yearsCan you please explain further? If I use
<context:component-scan>
I won't be able to override bean definition using XML? -
Sean Patrick Floyd over 12 years@user938214097 you can define beans either in XML or through annotations with component scanning
-
Admin over 12 yearsIs it enough to use the
<context:component-scan>
? Do I loose something if I don't use the<context:annotation-config>
? -
Sean Patrick Floyd over 12 years@Tomasz seems to have answered that
-
Koray Tugay over 8 yearsIs it possible to use component-scan but not annotation-config somehow?
-
CuriousMind almost 8 yearsThis one helped me to understand that <context:component-scan /> implicitly enables <context:annotation-config/>; that is it scans for bean definitions as well as does needed injection. I experimented with annotation-config="false", and the the injection didn't work unless I explicitly set using <context:annotation-config/>. Finally my understanding is better than before!
-
Sara over 5 yearsUse annotation-config="false" in context: annotation-config tag.
-
Bala almost 5 yearssuperb explanation Thanks. @Manuel Jordan