Difference between "//" and "/" in Python 2
Solution 1
//
is the floored-division operator in Python. The difference is visible when dividing floating point values.
In Python2, dividing two ints uses integer division, which ends up getting you the same thing as floored division. However, you can still use //
to get a floored result of floating point division. live example
# Python 2
>>> 10.0 / 3
3.3333333333333335
>>> 10.0 // 3
3.0
However, in Python3, dividing two ints results in a float, but using //
acts as integer division. live example
# Python3
>>> 10 / 3
3.3333333333333335
>>> 10 // 3
3
If you are (still) working in Python2, but want to someday convert to Python3, you should always use //
when dividing two ints, or use from __future__ import division
to get the Python3 behavior in Python2
Floored division means round towards negative infinity. This is the same as truncation for positive values, but not for negative. 3.3 rounds down to 3, but -3.3 rounds down to -4.
# Python3
>>> -10//3
-4
>>> 10//3
3
Solution 2
In python 2.7, to do real division you'll need to import division from a module named future:
from __future__ import division
Then, the /
will be the real (floating) division, i.e.:
15 / 4 => 3.75
And the //
will be the integer division (the integer part of the real division), i.e.:
15 // 4 => 3
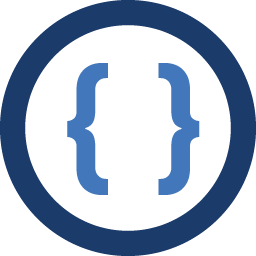
Admin
Updated on July 09, 2022Comments
-
Admin almost 2 years
Python 2 has two division operators:
/
and//
. The following output:========================================= RESTART: Shell ========================================= >>> for x in range(10): for y in range(1, 10): print x,'//',y,'=',x//y print x,'/',y,'=',x/y print 0 // 1 = 0 0 / 1 = 0 0 // 2 = 0 0 / 2 = 0 0 // 3 = 0 0 / 3 = 0 0 // 4 = 0 0 / 4 = 0 0 // 5 = 0 0 / 5 = 0 0 // 6 = 0 0 / 6 = 0 0 // 7 = 0 0 / 7 = 0 0 // 8 = 0 0 / 8 = 0 0 // 9 = 0 0 / 9 = 0 1 // 1 = 1 1 / 1 = 1 1 // 2 = 0 1 / 2 = 0 1 // 3 = 0 1 / 3 = 0 1 // 4 = 0 1 / 4 = 0 1 // 5 = 0 1 / 5 = 0 1 // 6 = 0 1 / 6 = 0 1 // 7 = 0 1 / 7 = 0 1 // 8 = 0 1 / 8 = 0 1 // 9 = 0 1 / 9 = 0 2 // 1 = 2 2 / 1 = 2 2 // 2 = 1 2 / 2 = 1 2 // 3 = 0 2 / 3 = 0 2 // 4 = 0 2 / 4 = 0 2 // 5 = 0 2 / 5 = 0 2 // 6 = 0 2 / 6 = 0 2 // 7 = 0 2 / 7 = 0 2 // 8 = 0 2 / 8 = 0 2 // 9 = 0 2 / 9 = 0 3 // 1 = 3 3 / 1 = 3 3 // 2 = 1 3 / 2 = 1 3 // 3 = 1 3 / 3 = 1 3 // 4 = 0 3 / 4 = 0 3 // 5 = 0 3 / 5 = 0 3 // 6 = 0 3 / 6 = 0 3 // 7 = 0 3 / 7 = 0 3 // 8 = 0 3 / 8 = 0 3 // 9 = 0 3 / 9 = 0 4 // 1 = 4 4 / 1 = 4 4 // 2 = 2 4 / 2 = 2 4 // 3 = 1 4 / 3 = 1 4 // 4 = 1 4 / 4 = 1 4 // 5 = 0 4 / 5 = 0 4 // 6 = 0 4 / 6 = 0 4 // 7 = 0 4 / 7 = 0 4 // 8 = 0 4 / 8 = 0 4 // 9 = 0 4 / 9 = 0 5 // 1 = 5 5 / 1 = 5 5 // 2 = 2 5 / 2 = 2 5 // 3 = 1 5 / 3 = 1 5 // 4 = 1 5 / 4 = 1 5 // 5 = 1 5 / 5 = 1 5 // 6 = 0 5 / 6 = 0 5 // 7 = 0 5 / 7 = 0 5 // 8 = 0 5 / 8 = 0 5 // 9 = 0 5 / 9 = 0 6 // 1 = 6 6 / 1 = 6 6 // 2 = 3 6 / 2 = 3 6 // 3 = 2 6 / 3 = 2 6 // 4 = 1 6 / 4 = 1 6 // 5 = 1 6 / 5 = 1 6 // 6 = 1 6 / 6 = 1 6 // 7 = 0 6 / 7 = 0 6 // 8 = 0 6 / 8 = 0 6 // 9 = 0 6 / 9 = 0 7 // 1 = 7 7 / 1 = 7 7 // 2 = 3 7 / 2 = 3 7 // 3 = 2 7 / 3 = 2 7 // 4 = 1 7 / 4 = 1 7 // 5 = 1 7 / 5 = 1 7 // 6 = 1 7 / 6 = 1 7 // 7 = 1 7 / 7 = 1 7 // 8 = 0 7 / 8 = 0 7 // 9 = 0 7 / 9 = 0 8 // 1 = 8 8 / 1 = 8 8 // 2 = 4 8 / 2 = 4 8 // 3 = 2 8 / 3 = 2 8 // 4 = 2 8 / 4 = 2 8 // 5 = 1 8 / 5 = 1 8 // 6 = 1 8 / 6 = 1 8 // 7 = 1 8 / 7 = 1 8 // 8 = 1 8 / 8 = 1 8 // 9 = 0 8 / 9 = 0 9 // 1 = 9 9 / 1 = 9 9 // 2 = 4 9 / 2 = 4 9 // 3 = 3 9 / 3 = 3 9 // 4 = 2 9 / 4 = 2 9 // 5 = 1 9 / 5 = 1 9 // 6 = 1 9 / 6 = 1 9 // 7 = 1 9 / 7 = 1 9 // 8 = 1 9 / 8 = 1 9 // 9 = 1 9 / 9 = 1 >>>
proves (almost?) all the time
a/b
equalsa//b
. Is there any time that it isn't? If not, why did Python 2 include two operators that do the same thing? -
Admin almost 8 yearsThanks. Now I understand.
-
kindall almost 8 yearsIn Python 2, you can
from __future__ import division
to get the Python 3 behavior of/
.