Difference between thenAccept and thenApply
Solution 1
You need to look at the full method signatures:
CompletableFuture<Void> thenAccept(Consumer<? super T> action)
<U> CompletableFuture<U> thenApply(Function<? super T,? extends U> fn)
thenAccept
takes a Consumer
and returns a T=Void
CF, i.e. one that does not carry a value, only the completion state.
thenApply
on the other hand takes a Function
and returns a CF carrying the return value of the function.
Solution 2
thenApply
returns result of curent stage whereas thenAccept
does not.
Read this article: http://codeflex.co/java-multithreading-completablefuture-explained/
Solution 3
As the8472 clearly explained, they are differentiate by their output value and args and thus what you can do with them
CompletableFuture.completedFuture("FUTURE")
.thenApply(f -> f.toLowerCase())
.thenAccept(f -> System.out.println(f))
.thenAccept(f -> System.out.println(f))
.thenApply(f -> new String("FUTURE"))
.thenAccept(f -> System.out.println(f));
future
null
FUTURE
the Apply functions apply another function and pass a future holding a value
the Accept functions consume this value and returns future holding void
Solution 4
I would answer this question in the way I remember the difference between the two: Consider the following future.
CompletableFuture<String> completableFuture
= CompletableFuture.supplyAsync(() -> "Hello");
ThenAccept
basically takes a consumer and passes it the result of the computation CompletableFuture<Void>
CompletableFuture<Void> future = completableFuture
.thenAccept(s -> System.out.println("Computation returned: " + s));
You can associate this with forEach
in streams
for easy remembering.
Where as thenApply
accepts a Function
instance, uses it to process the result and returns a Future that holds a value returned by a function:
CompletableFuture<String> future = completableFuture
.thenApply(s -> s + " World");
You can associate this with map
in the streams
as it is actually performing a transformation.
Related videos on Youtube
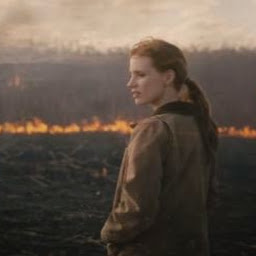
Anqi Lu
Updated on June 25, 2020Comments
-
Anqi Lu almost 4 years
I'm reading the document on
CompletableFuture
and The description forthenAccept()
isReturns a new CompletionStage that, when this stage completes normally, is executed with this stage's result as the argument to the supplied action.
and for
thenApply()
isReturns a new CompletionStage that, when this stage completes normally, is executed with this stage's result as the argument to the supplied function.```
Can anyone explain the difference between the two with some simple examples?
-
Anver Sadhat over 5 yearsIf we take it as compare to Stream, thenAccept() is some what similar to forEach (Consumer ) and theApply is similar to map (Function)