Different between parseInt() and valueOf() in java?
Solution 1
Well, the API for Integer.valueOf(String)
does indeed say that the String
is interpreted exactly as if it were given to Integer.parseInt(String)
. However, valueOf(String)
returns a new
Integer()
object whereas parseInt(String)
returns a primitive int
.
If you want to enjoy the potential caching benefits of Integer.valueOf(int)
, you could also use this eyesore:
Integer k = Integer.valueOf(Integer.parseInt("123"))
Now, if what you want is the object and not the primitive, then using valueOf(String)
may be more attractive than making a new object out of parseInt(String)
because the former is consistently present across Integer
, Long
, Double
, etc.
Solution 2
From this forum:
parseInt()
returns primitive integer type (int), wherebyvalueOf
returns java.lang.Integer, which is the object representative of the integer. There are circumstances where you might want an Integer object, instead of primitive type.Of course, another obvious difference is that intValue is an instance method whereby parseInt is a static method.
Solution 3
Integer.valueOf(s)
is similar to
new Integer(Integer.parseInt(s))
The difference is valueOf()
returns an Integer
, and parseInt()
returns an int
(a primitive type). Also note that valueOf()
can return a cached Integer
instance, which can cause confusing results where the result of ==
tests seem intermittently correct. Before autoboxing there could be a difference in convenience, after java 1.5 it doesn't really matter.
Moreover, Integer.parseInt(s)
can take primitive datatype as well.
Solution 4
Look at Java sources: valueOf
is using parseInt
:
/**
* Parses the specified string as a signed decimal integer value.
*
* @param string
* the string representation of an integer value.
* @return an {@code Integer} instance containing the integer value
* represented by {@code string}.
* @throws NumberFormatException
* if {@code string} cannot be parsed as an integer value.
* @see #parseInt(String)
*/
public static Integer valueOf(String string) throws NumberFormatException {
return valueOf(parseInt(string));
}
parseInt
returns int
(not Integer
)
/**
* Parses the specified string as a signed decimal integer value. The ASCII
* character \u002d ('-') is recognized as the minus sign.
*
* @param string
* the string representation of an integer value.
* @return the primitive integer value represented by {@code string}.
* @throws NumberFormatException
* if {@code string} cannot be parsed as an integer value.
*/
public static int parseInt(String string) throws NumberFormatException {
return parseInt(string, 10);
}
Solution 5
Integer.parseInt can just return int as native type.
Integer.valueOf may actually need to allocate an Integer object, unless that integer happens to be one of the preallocated ones. This costs more.
If you need just native type, use parseInt. If you need an object, use valueOf.
Also, because of this potential allocation, autoboxing isn't actually good thing in every way. It can slow down things.
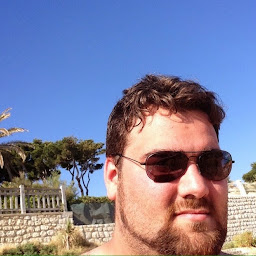
Comments
-
gregory boero.teyssier almost 2 years
How is
parseInt()
different fromvalueOf()
?They appear to do exactly the same thing to me (also goes for
parseFloat()
,parseDouble()
,parseLong()
etc, how are they different fromLong.valueOf(string)
?Also, which one of these is preferable and used more often by convention?
-
Adam Rosenfield over 15 yearsvalueOf() can return the same object for successive calls with the same argument (and is required to for arguments between -128 and 127 inclusive). new Integer() will always create a new object.
-
gregory boero.teyssier over 15 yearsWhich one is used more often? Which one should I use the most?
-
Anne Porosoff over 15 yearsWorth mentioning: valueOf versions will also use an internal reference pool to return the SAME object for a given value, not just another instance with the same internal value. This means that given two Longs returned in this way, a.equals(b) == true and a == b is true
-
palantus over 15 yearsActually, not quite true. I thought so myself at first, but the Javadocs for Integer.valueOf(String) clearly state that it is equivalent to new Integer(Integer.parseInt(String)). Integer.valueOf(int) does indeed cache, though.
-
OscarRyz over 15 years@bassezero. Also, that pool has a limit. I think it was -127 to 127.
-
Donal Fellows almost 14 yearsThe size of the reference pool is a true example of an implementation detail; it could even be increased in size in a patch release, and you should never rely on it for anything.
-
Logan over 12 yearsIs there any Performance or Memory difference between the two approaches?
-
Thomas Eding over 11 years
Integer.valueOf(Integer.parseInt("123"))
has no benefit overInteger.valueOf("123")
orInteger.valueOf(123)
aside from wasting cycles and the size of your program. -
foo almost 11 yearsThere is a difference - the new Object (potentially) allocated by valueOf comes with an overhead (memory for the object, handling, GC), while the plain int is extremely "lightweight". (For the most common values, you'll get references to pre-existing Objects, which helps a tiny bit.)
-
Holger over 9 years
Integer.valueOf(String)
does exactly the same caching asInteger.valueOf(int)
. In fact, it is implemented asInteger.valueOf(Integer.parseInt(…))
… -
Khez over 9 yearsAt least in Java 1.8.0,
Integer.valueOf(123)
returns the primitive int. Testing showed that between[-127, 128]
it returns theprimitive not the object
, which is exactly asigned byte
. -
user207421 almost 9 years@Khez It is impossible for it to return a primitive
int
. The signature says it returns anInteger
, and that is exactly what it does. This answer is also partially incorrect when it says it returns a 'new'Integer
. That's not what it says in the Javadoc. It is free to return a cachedInteger
. -
Jean-François Savard almost 9 years@OscarRyz Actually it's -128 to 127. Note that JVM offer a parameter to set the highest bound higher for the cache. However, you can't re-define the lowest bound : stackoverflow.com/questions/29633158/…
-
Simon Forsberg almost 9 yearsNo one has mentioned
intValue
, why does your answer mentionsintValue
? -
Ciro Santilli OurBigBook.com over 8 yearsDocumentation bug. Love it.
-
MC Emperor about 7 years"
valueOf(String)
returns anew Integer()
object" — Not entirely. At least in theInteger
class implementation in Java 8,Integer
s with a value between -128 and 127 are cached.Integer.valueOf("42") == Integer.valueOf("42")
returnstrue
. -
Zoe stands with Ukraine over 5 yearsPlease don't abuse bold formatting: it degrades the readability of your post.
-
kaya3 over 4 years
Integer k = Integer.valueOf(Integer.parseInt("123"));
is equivalent toInteger k = Integer.parseInt("123");
because autoboxing is done by callingInteger.valueOf
anyway. But as noted,Integer.valueOf(String)
does use the cache too. -
Pratik about 4 years@Joan d Silva from your last line, I think Integer.parseInt(s) can only take as an String whereas Integer.ValueOf(s) can take both int and string as an input argument
-
Torben over 2 years@basszero This is no longer true. At least since Java 8, the
Integer.valueOf(String)
simply combinesInteger.parseInt(String)
andInteger.valueOf(int)
.