Disable AlertDialog button on value change of TextFormField Flutter
878
Copy Paste This Code, Its Working fine, I have used StateFullBuilder
Widget for updating the state
of the AlertDialogBox
.
For More Reference On updating or refreshing the DialogBox
Click HERE
Below Is The Code
import 'dart:async';
import 'package:flutter/material.dart';
class MyTimer extends StatefulWidget {
@override
_MyTimerState createState() => _MyTimerState();
}
class _MyTimerState extends State<MyTimer> {
bool isValidAlert = false;
bool isValidScafold = false;
_displayDialog(BuildContext context) async {
return showDialog(
context: context,
builder: (context) {
return StatefulBuilder(builder: (context, setState) {
return AlertDialog(
title: Text('TextField AlertDemo'),
content: _containerAlert(setState),
actions: <Widget>[
RaisedButton(
onPressed: isValidAlert
? () {
print("ISVALID:");
}
: null,
child: Text("Click Me"),
)
],
);
});
});
}
Widget _container() {
return Container(
margin: EdgeInsets.all(25),
child: Center(
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: [
TextFormField(
onChanged: (text) {
setState(() {
if (text.length > 5) {
isValidScafold = true;
} else {
isValidScafold = false;
}
});
},
decoration: InputDecoration(labelText: 'Enter Text'),
),
SizedBox(height: 15),
RaisedButton(
onPressed: isValidScafold
? () {
print("ISVALID:");
}
: null,
child: Text("Done!"),
)
],
),
),
);
}
Widget _containerAlert(StateSetter setState) {
return Container(
margin: EdgeInsets.all(25),
child: Center(
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: [
TextFormField(
onChanged: (text) {
setState(() {
if (text.length > 5) {
isValidAlert = true;
} else {
isValidAlert = false;
}
print(text);
});
},
decoration: InputDecoration(labelText: 'Enter Text'),
),
SizedBox(height: 15),
RaisedButton(
onPressed: isValidAlert
? () {
print("ISVALID:");
}
: null,
child: Text("Done!"),
)
],
),
),
);
}
@override
Widget build(BuildContext context) {
return Scaffold(
body: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: [
_container(),
RaisedButton(
onPressed: () {
_displayDialog(context);
},
child: Text("Show Alert"),
)
],
),
);
}
}
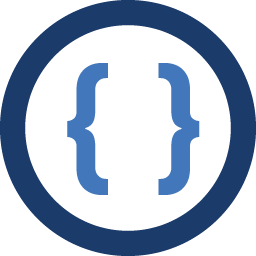
Author by
Admin
Updated on December 26, 2022Comments
-
Admin over 1 year
In My First Flutter Project, I am trying to get value from TextFormField inside a Alert Dialog. I am trying to validate inputs and based on that validation I want to enable/disable button of AlertDialog.
What I have done to display dialog:
_displayDialog(BuildContext context) async { return showDialog( context: context, builder: (context) { return AlertDialog( title: Text('TextField AlertDemo'), content: _container(), actions: <Widget>[ RaisedButton( onPressed: isValid ? () { print("ISVALID:"); } : null, child: Text("Click Me"), ) ], ); }); }
_container() method code:
Widget _container() { return Container( margin: EdgeInsets.all(25), child: Center( child: Column( mainAxisAlignment: MainAxisAlignment.center, children: [ TextFormField( onChanged: (text) { setState(() { if (text.length > 5) { isValid = true; } else { isValid = false; } }); }, decoration: InputDecoration(labelText: 'Enter Text'), ), SizedBox(height: 15), RaisedButton( onPressed: isValid ? () { print("ISVALID:"); } : null, child: Text("Done!"), ) ], ), ), ); }
Unfortunately It is working in body of Scaffold:
@override Widget build(BuildContext context) { return Scaffold( body: Column( mainAxisAlignment: MainAxisAlignment.center, children: [ _container(), RaisedButton( onPressed: (){_displayDialog(context);}, child: Text("Show Alert"), ) ], ), ); }
Can anyone please help me out in this problem. TIA