Disable Tabs in TabLayout
Solution 1
there are 3 methods implemented by the tab click listener, one of them is onTabSelected() put a boolean condition to check if your fragment is initialised. Then if that condition is satisfied then allow transaction to take place. Also initialize the tabs after your fragment code
Solution 2
You can create a util function, my fun in Kotlin:
fun disableTabAt(tablayout: TabLayout?, index: Int) {
(tablayout?.getChildAt(0) as? ViewGroup)?.getChildAt(index)?.isEnabled = false
}
When you want do something with a view, you can debug or click in parent view to know how it's created. By this way you can do anything that you want. For this case, you can go to Tablayout class to understand.
Solution 3
To enable specific tab at position :
LinearLayout tabStrip = ((LinearLayout)tabLayout.getChildAt(0));
tabStrip.getChildAt(position).setOnTouchListener((v,event)->false);
To disable the tab at position :
tabStrip.getChildAt(position).setOnTouchListener((v,event)->true);
Solution 4
Here's 2 helper functions (kotlin) for disabling and enabling a TabItem by passing its name.
Tested using com.google.android.material:material:1.3.0-alpha02
fun disableTabItemAt(tabLayout: TabLayout?, tabText: String) {
(tabLayout?.getChildAt(0) as? ViewGroup)?.children?.iterator()?.forEach {
if((it as TabLayout.TabView).tab?.text == tabText) {
it.isEnabled = false
it.alpha = 0.5f
}
}
}
fun enableTabItemAt(tabLayout: TabLayout?, tabText: String) {
(tabLayout?.getChildAt(0) as? ViewGroup)?.children?.iterator()?.forEach {
if((it as TabLayout.TabView).tab?.text == tabText) {
it.isEnabled = true
it.alpha = 1f
}
}
}
So if your tab names are fixed, then you could do
disableTabItemAt(tabLayout, "Tab1")
// later enable it
enableTabItemAt(tabLayout, "Tab1")
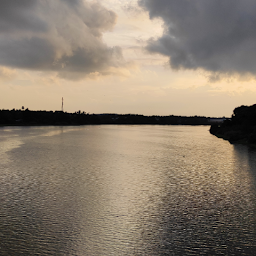
Abhijith Surendran
Updated on July 20, 2022Comments
-
Abhijith Surendran almost 2 years
I have used
TabLayout
from the latest design support library in my app. The tabs are attached to a viewpager which loads the fragments for each tab. I want to disable all the tabs until the viewpager loads the fragment for user selected tab. I am not able to disable the tablayout or make it non-clickable. I had usedsetEnabled(false)
andsetClickable(false)
but it is not working. I am able to make it invisible by usingsetVisiblity(View.GONE)
but I want the tabs to be visible at all times.tabLayout = (TabLayout) findViewById(R.id.tabLayout); tabLayout.setTabGravity(TabLayout.GRAVITY_FILL); tabLayout.setTabMode(TabLayout.MODE_FIXED); tabLayout.addTab(tabLayout.newTab().setIcon(R.drawable.near_me_hover).setTag(1)); tabLayout.addTab(tabLayout.newTab().setIcon(R.drawable.all_hostels).setTag(2)); tabLayout.addTab(tabLayout.newTab().setIcon(R.drawable.top_five).setTag(3)); tabLayout.addTab(tabLayout.newTab().setIcon(R.drawable.advanced_search).setTag(4)); tabLayout.setEnabled(false); tabLayout.setClickable(false);
XML
android.support.design.widget.TabLayout
android:id="@+id/tabLayout" android:layout_width="match_parent"
android:layout_height="0dp"
android:layout_weight="0.15"
android:scrollbars="horizontal"
android:splitMotionEvents="false" >@Override public void onTabSelected(TabLayout.Tab tab) { switch (tab.getPosition()) { case 0: viewPager.setCurrentItem(tab.getPosition()); tab.setIcon(R.drawable.near_me_hover); break; case 1: viewPager.setCurrentItem(tab.getPosition()); tab.setIcon(R.drawable.all_hostels_hover); break; case 2: viewPager.setCurrentItem(tab.getPosition()); tab.setIcon(R.drawable.top_five_hover); break; case 3: viewPager.setCurrentItem(tab.getPosition()); tab.setIcon(R.drawable.advanced_search_hover); break; } } @Override public void onTabUnselected(TabLayout.Tab tab) { switch (tab.getPosition()) { case 0: tab.setIcon(R.drawable.near_me); break; case 1: tab.setIcon(R.drawable.all_hostels); break; case 2: tab.setIcon(R.drawable.top_five); break; case 3: tab.setIcon(R.drawable.advanced_search); break; } } @Override public void onTabReselected(TabLayout.Tab tab) { } }); viewPager.addOnPageChangeListener(new ViewPager.OnPageChangeListener() { @Override public void onPageScrolled(int position, float positionOffset, int positionOffsetPixels) { } @Override public void onPageSelected(int position) { tabLayout.getTabAt(position).select(); } @Override public void onPageScrollStateChanged(int state) { } });