Disable the Excel export option when I generate a report via the ReportViewer
Solution 1
While on the face of it this seems easy, the export options are difficult to get hold of. You can get the toolstrip of the reportviewer by simply doing this:
Dim myToolStrip As ToolStrip = DirectCast(ReportViewer1.Controls.Find("toolStrip1", True)(0), ToolStrip)
...and you can iterate through the .Items collection to do what you like with the buttons, however the DropDownItems collection for the export button always appears empty.
So the easy solution is to get rid of the default export button and add your own with just the functionality you need. So in your form constructor:
//Hide the default export button
ReportViewer1.ShowExportButton = False
//Define a new button
Dim newExportButton As New ToolStripButton("Export PDF", Nothing, AddressOf Me.ExportPDF, "newExport")
//And add it to the toolstrip
DirectCast(ReportViewer1.Controls.Find("toolStrip1", True)(0), ToolStrip).Items.Add(newExportButton)
Then all you need to do is to take care of the actual exporting:
Private Sub ExportPDF()
Dim warnings As Microsoft.Reporting.WinForms.Warning()
Dim streamids As String()
Dim mimeType As String = ""
Dim encoding As String = ""
Dim extension As String = ""
Dim bytes As Byte() = ReportViewer1.LocalReport.Render("PDF", Nothing, mimeType, encoding, extension, streamids, warnings)
Dim fs As New IO.FileStream("C:\export.pdf", IO.FileMode.Create)
fs.Write(bytes, 0, bytes.Length)
fs.Close()
fs.Dispose()
End Sub
Solution 2
This will accomplish what the poster asked (uses a little LINQ for selecting objects of certain types from collections):
private void DisableReportViewerExportExcel()
{
var toolStrip = this.ReportViewer.Controls.Find("toolStrip1", true)[0] as ToolStrip;
if (toolStrip != null)
foreach (var dropDownButton in toolStrip.Items.OfType<ToolStripDropDownButton>())
dropDownButton.DropDownOpened += new EventHandler(dropDownButton_DropDownOpened);
}
void dropDownButton_DropDownOpened(object sender, EventArgs e)
{
if (sender is ToolStripDropDownButton)
{
var ddList = sender as ToolStripDropDownButton;
foreach (var item in ddList.DropDownItems.OfType<ToolStripDropDownItem>())
if (item.Text.Contains("Excel"))
item.Enabled = false;
}
}
Solution 3
//Call This function from Page_Load Event
private void CustomizeRV(System.Web.UI.Control reportControl)
{
foreach (System.Web.UI.Control childControl in reportControl.Controls)
{
if (childControl.GetType() == typeof(System.Web.UI.WebControls.DropDownList))
{
System.Web.UI.WebControls.DropDownList ddList = (System.Web.UI.WebControls.DropDownList)childControl;
ddList.PreRender += new EventHandler(ddList_PreRender);
}
if (childControl.Controls.Count > 0)
{
CustomizeRV(childControl);
}
}
}
//Dropdown prerender event
//You can hide any option from ReportViewer( Excel,PDF,Image )
void ddList_PreRender(object sender, EventArgs e)
{
if (sender.GetType() == typeof(System.Web.UI.WebControls.DropDownList))
{
System.Web.UI.WebControls.DropDownList ddList = (System.Web.UI.WebControls.DropDownList)sender;
System.Web.UI.WebControls.ListItemCollection listItems = ddList.Items;
if ((listItems != null) && (listItems.Count > 0) && (listItems.FindByText("Excel") != null))
{
foreach (System.Web.UI.WebControls.ListItem list in listItems)
{
if (list.Text.Equals("Excel"))
{
list.Enabled = false;
}
}
}
}
}
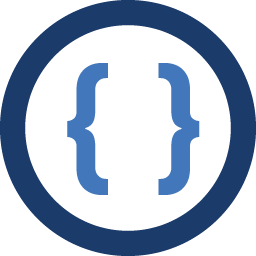
Admin
Updated on June 23, 2022Comments
-
Admin almost 2 years
How can I disable the Excel export option when I generate a report, via the ReportViewer, in my winforms application?
ADDITION: In particular, I want to hide the toolbar button that refers to Excel output/export task, and not .the one that handles to the pdf export option.