Disabling vim visual mode in /etc/vim/vimrc does not work
Solution 1
Debian's /etc/vim/vimrc
contains this comment:
" Vim will load $VIMRUNTIME/defaults.vim if the user does not have a vimrc.
" This happens after /etc/vim/vimrc(.local) are loaded, so it will override
" any settings in these files.
" If you don't want that to happen, uncomment the below line to prevent
" defaults.vim from being loaded.
" let g:skip_defaults_vim = 1
As :verbose set mouse?
says, that was set by /usr/share/vim/vim81/defaults.vim
mentioned above ($VIMRUNTIME
on Debian would be /usr/share/vim/vim<version>
).
So, you can either create a ~/.vimrc
(or ~/.vim/vimrc
) for your user (even an empty one will do), or uncomment let g:skip_defaults_vim = 1
in /etc/vim/vimrc
.
Solution 2
Instead of muru's answer, I just straight edited the default at /usr/share/vim/vim81/defaults.vim
(or whatever your vim version is).
Comment out set mouse=a
by changing it to "set mouse=a
"
is how you comment out stuff in vim config files
This has the benefit of keeping useful stuff in the defaults like syntax highlighting but removing that annoying visual mode
I'm probably gonna get replies that I shouldn't do this because it'll be overwritten when vim updates but it's a quick fix for me now!
Solution 3
$ cat >.vimrc
source $VIMRUNTIME/defaults.vim
set mouse=
^D
$
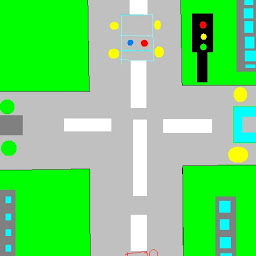
clavlav12
Updated on September 18, 2022Comments
-
clavlav12 over 1 year
The full code:
import glob import pygame import os os.environ['SDL_VIDEO_WINDOW_POS'] = "%d,%d" % (100, 40) class Man(pygame.sprite.Sprite): # just taking random image to get height and width after trans scale to be able to crop later (see lines 23/36) idle_images_list = glob.glob(r"C:\Users\aviro\Desktop\מחשבים\python projects\platform game\animation\fighter\PNG\PNG Sequences\Firing"+'\*') random_image = pygame.image.load(idle_images_list[0]) new_img_width = int(random_image.get_width()/2.5) new_img_height = int(random_image.get_height()/2.5) random_image_after_crop = random_image.subsurface((70, 35, new_img_width-125, new_img_height-45)) # to be able to use needed images indexes # getting idle animations list right_idle_list = [] left_idle_list = [] for images in idle_images_list: img = pygame.image.load(images) wid = img.get_width() hei = img.get_height() img = pygame.transform.scale(img, (int(wid/2.5), int(hei/2.5))) img = img.subsurface((70, 35, new_img_width-125, new_img_height-45)) right_idle_list.append(img) left_idle_list.append(pygame.transform.flip(img, True, False)) # getting movement animations list walk_animation_list = glob.glob(r"C:\Users\aviro\Desktop\מחשבים\python projects\platform game\animation\fighter\PNG\PNG Sequences\Run Firing"+'\*') walk_right_list = [] walk_left_list = [] for files in walk_animation_list: # setting the animation list img = pygame.image.load(files) wid = img.get_width() hei = img.get_height() img = pygame.transform.scale(img, (int(wid/2.5), int(hei/2.5))) # chaging scale img = img.subsurface((70, 35, new_img_width-125, new_img_height-45)) walk_right_list.append(img) walk_left_list.append(pygame.transform.flip(img, True, False)) # mirror list def __init__(self, x, y,): super(Man, self).__init__() self.walk_left_list = Man.walk_left_list self.walk_right_list = Man.walk_right_list self.width = Man.new_img_width self.height = Man.new_img_height self.hitbox = (x+55, y+35, self.width-125, self.height-45) # nothing special on those num, just Trial and error self.rect = Man.random_image_after_crop.get_rect() self.rect.x = x self.rect.y = y def game_redraw(): # print things in end for main loop global right global left screen.blit(bg_image, (0, 0)) if right: screen.blit(man.walk_right_list[frame_count//3], (man.rect.x, man.rect.y)) elif left: screen.blit(man.walk_left_list[frame_count//3], (man.rect.x, man.rect.y)) else: if last_action == "right": screen.blit(man.right_idle_list[frame_count//13], (man.rect.x, man.rect.y)) if last_action == "left": screen.blit(man.left_idle_list[frame_count//13], (man.rect.x, man.rect.y)) else: # just for the first move screen.blit(man.right_idle_list[frame_count//13], (man.rect.x, man.rect.y)) pygame.draw.rect(screen, RED, man.rect, 4) pygame.display.flip() right = False left = False def input_process(key): global right global left global last_action global man global frame_count global is_jump global neg global jump_count # if is_jump: if jump_count >= -10: # check if jumping if jump_count < 0: neg = -1 man.rect.y -= (jump_count ** 2) * neg * 0.5 jump_count -= 1 else: neg = 1 is_jump = False # if key[pygame.K_RIGHT] and man.rect.right + speed < w: if left: frame_count = 0 right = True left = False last_action = "right" man.rect.x += speed # if key[pygame.K_LEFT] and man.rect.left + speed > 0: if right: frame_count = 0 right = False left = True last_action = "left" man.rect.x -= speed # if not is_jump: if key[pygame.K_UP]: jump_count = 10 is_jump = True w = 1728 h = 972 pygame.init() RED = (255, 0, 0) images_folder = r'C:\Users\aviro\Desktop\מחשבים\python projects\platform game\images'+'\\' bg_image = images_folder+"background.png" # setting background image ground_height = h-143 # the high of the image ground man = Man(200, ground_height) man.rect.bottom = ground_height clock = pygame.time.Clock() Refresh_Rate = 54 speed = 5 right = False left = False frame_count = 0 finish = False last_action = "" # jumping ver is_jump = False jump_count = 10 neg = 1 # screen = pygame.display.set_mode((w, h)) bg_image = pygame.image.load(bg_image).convert() # can do convert only after setting surface. # main loop while not finish: if frame_count >= 51: frame_count = 0 for events in pygame.event.get(): if events.type == pygame.QUIT: finish = True key = pygame.key.get_pressed() input_process(key) game_redraw() frame_count += 1 clock.tick(Refresh_Rate)
I'm trying to create a simple shooting-platform game, using pygame module. Everything works fine except the jumping. See in line 88. The player is jumping like an x^2 parabola. I added the *0.5 at the end of the line to make the player jump slower and lower, but when I do it this is what happened.
Before jumping:
After jumping:
Look in the second picture. There's a distance between the floor and the player. When I remove the *0.5 everything works fine. Why?
-
muru over 4 yearsWhat is the output of
:verbose set mouse?
in Vim, when you haveset mouse=-a
in/etc/vim/vimrc
but not in~/.vimrc
? Also, does the output of:scriptnames
include/etc/vim/vimrc
? -
Thorian93 over 4 yearsHi, the first command tells me, that it ignores my /etc/vim/vimrc:
mouse=a Last set from /usr/share/vim/vim81/defaults.vim line 79
. For the secont part: No it does not.
-
-
Thorian93 over 4 yearsThat's exactly what I needed, thanks a lot! This has been bugging me for some time and know I understand the behaviour. An interesting way of configuration though.
-
Thorian93 over 3 yearsI might miss something, but why don't you do that in
/etc/vim/vimrc
? -
Khaled Mousa over 3 years@Thorian93 I tried that, then I lost all the options from the default file like syntax highlighting. I could very easily be doing something wrong.
-
Thorian93 over 3 yearsI see. From what I know up to now the clean way would be to write your own
.vimrc
file with the settings you want. That way you can be sure your settings are correct and they won't be overwritten by package upgrades. -
Paul Wieland about 3 yearsThank you for this. By adding a ~/.vimrc all the defaults provided by defaults.vim are lost (color coding for example).
-
muru over 2 yearsSuch a change will be overwritten when the package is updated.