Display and render only a specific object in wireframe in Unity3D
Solution 1
Use Layers. Change the layer of the game object (dropdown in the top right of the Inspector window) from Default to another layer (you can create a new one choosing Add Layer... in the dropdown menu).
Then, create a new camera (or select the main camera, depending on what you want to achieve), and change its Culling Mask to the layer you are using in the game object.
For drawing the wireframe, you post this script in the camera that's supposed to draw that game object:
// from http://docs.unity3d.com/ScriptReference/GL-wireframe.html
using UnityEngine;
using System.Collections;
public class ExampleClass : MonoBehaviour {
void OnPreRender() {
GL.wireframe = true;
}
void OnPostRender() {
GL.wireframe = false;
}
}
You may have to use 2 cameras depending on what you want to achieve (one camera for the wireframe object, another camera to draw the rest of the scene), in this case you would set the Clear Flags of one of the cameras to Don't Clear. Make sure the Depth value of both cameras is the same.
The Clear Flags of a camera indicates what's going to happen with the pixels where there's nothing to be drawn (the empty space) of that camera, and also what happens when there are multiple cameras drawing to the same pixel.
In the case where Clear Flags is Don't Clear, it will not do anything with the empty space, leaving it for the other camera to fill with an object or a background. For the pixels where it should draw something, it will let the depth of the object decide what is going to be drawn, that is, the objects that are nearer the camera will be drawn on the top of the others.
Solution 2
There is an asset on the Unity asset store containing several shader materials which do exactly what you need. You can download it, analyze and write the shaders yourself, or simply use the asset provided.
You can then attach the shader to a material and that to a mesh renderer to attain the desired effect.
Related videos on Youtube
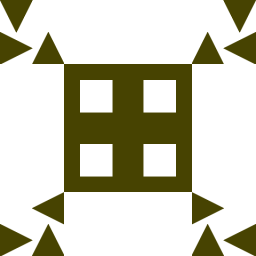
Tak
Apparently, this user prefers to keep an air of mystery about them
Updated on June 04, 2022Comments
-
Tak 12 months
I want to know how to view and render a specific game object (mesh) in wireframe, not the whole scene. I can change the scene to wireframe using
GL.wireframe
but the problem I want to view and render only a certain object (not the whole scene) in wireframe. Any advice please? -
Tak almost 8 yearsThanks for your answer. I already did that but this change the whole scene to wireframe, I only want a certain object or mesh.
-
Tak almost 8 yearsI don't mind having two cameras. And I did exactly what you said but the camera who is responsible for drawing the scene is the only one that renders, the camera which is responsible for the wireframe object doesn't display the object. Any thoughts please? I will upvote your answer anyway for your kind assistance.
-
Tak almost 8 yearsand both cameras are on the same position by the way. But
camera2
is set to view a certain object andcamera1
is set to view the rest of the scene. But when rendering only the rest of the scene appears usingcamera1
. -
MickyD almost 8 years
-
Martin Rončka almost 8 yearsThat is no longer true. Unity 5 free supports shaders. I tested this myself. And even if so, you still can analyse the shaders :). Anyway, this is the solution in case of
gl.wireframe
not being supported. -
Roberto almost 8 years@shepherd Did you see my comment?