Display GPU Usage While Code is Running in Colab
Solution 1
Used wandb
to log system metrics:
!pip install wandb
import wandb
wandb.init()
Which outputs a URL in which you can view various graphs of different system metrics.
Solution 2
If you have Colab Pro, can open Terminal, located on the left side, indicated as '>_' with a black background.
You can run commands from there even when some cell is running
Write command to see GPU usage in real-time:
watch nvidia-smi
Solution 3
There is another way to see gpu usage but this method only works for seeing the memory usage. Go to click runtime -> manage sessions. This allows you to see how much memory it takes so that you can increase your batch size.
Solution 4
A little more clear explaination.
- Go to weights and bias and create your account.
- Run the following commands.
!pip install wandb import wandb wandb.init()
- Go to the link in your notebook for authorization - copy the API key.
- Paste the key in notebook input field.
- After Authorization you will find another link in notebook - see your Model + System matrices there.
Solution 5
You can run a script in background to track GPU usage.
Step 1: Create a file to monitor GPU usage in a jupyter cell.
%%writefile gpu_usage.sh
#! /bin/bash
#comment: run for 10 seconds, change it as per your use
end=$((SECONDS+10))
while [ $SECONDS -lt $end ]; do
nvidia-smi --format=csv --query-gpu=power.draw,utilization.gpu,memory.used,memory.free,fan.speed,temperature.gpu >> gpu.log
#comment: or use below command and comment above using #
#nvidia-smi dmon -i 0 -s mu -d 1 -o TD >> gpu.log
done
Step 2: Execute the above script in the background in another cell.
%%bash --bg
bash gpu_usage.sh
Step 3: Run the inference.
Note that the script will record GPU usage for first 10 seconds, change it as per your model running time.
The GPU utilization results will be saved in gpu.log
file.
Related videos on Youtube
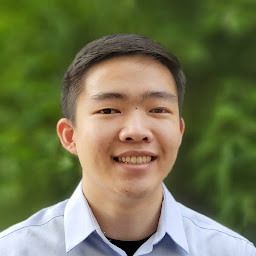
Matthew So
Updated on July 05, 2022Comments
-
Matthew So almost 2 years
I have a program running on Google Colab in which I need to monitor GPU usage while it is running. I am aware that usually you would use
nvidia-smi
in a command line to display GPU usage, but since Colab only allows one cell to run at once at any one time, this isn't an option. Currently, I am usingGPUtil
and monitoring GPU and VRAM usage withGPUtil.getGPUs()[0].load
andGPUtil.getGPUs()[0].memoryUsed
but I can't find a way for those pieces of code to execute at the same time as the rest of my code, thus the usage numbers are much lower than they actually should be. Is there any way to print the GPU usage while other code is running? -
Vinay Verma over 3 yearsA little more clear explaination. Go to weights and bias and create your account Run the above commands Go to the link in your notebook for authorization - copy the API key Paste the key in notebook input field Then you will find a link go to that - and see your Model + System matrices.
-
stason about 3 yearsIt isn't normally available, says: Sign up for Colab Pro to gain access to terminal functionality
-
climbest about 3 yearsYes, @stason , u r right, and sorry I didn't clarify this condition bc I haven't tested it on Colab Free