Display json data from Ajax and display on JQuery Dialog with in MVC3 View
10,724
Solution 1
$.post("/echo/json/",function(data){
//in actuality the json will be parsed here
var d = '{"id":"1","name":"john","age":26}';
var json = $.parseJSON(d);
$("<div/>",{text:json.name+" "+json.age}).appendTo("body");
$("div").dialog();
},'json')
Solution 2
There are a bunch of ways of doing that. Here is an example: http://geekswithblogs.net/michelotti/archive/2008/06/28/mvc-json---jsonresult-and-jquery.aspx
Basically, you have to access the properties of the data
parameter of the success
handler.
...
success: function (data) {
alert(data.property);
}
...
One thing to note is to add the dataType: "json"
option on the AJAX call, so you don't have to parse the data after receiving it.
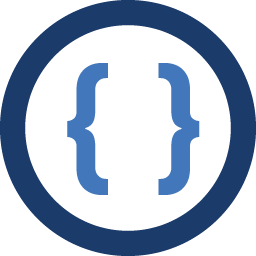
Author by
InCommand
Updated on June 06, 2022Comments
-
InCommand almost 2 years
I have the Following ActionResult in a Controller. it returns a row of data(such as id, name, city etc) from database depending on the ID
[HttpPost] public ActionResult Get(Guid Id) { Ref imp = ReadRepository.GetById(refImpId); var ijson = new JsonResult() { Data = imp.ToJson() }; return ijson; }
the Following is the JQuery and Ajax for Jquery Dialog.
$(".ImpList").click(function (e) { // get the imp id var refImpId = $(this).next('#impId').val(); var impgeturl = $('#impgeturl').val(); var imptoedit = null; $.ajax({ type: 'post', url: impgeturl, data: '{ "refImpId": "' + refImpId + '" }', contentType: "application/json; charset=utf-8", traditional: true, success: function (data) { imptoedit = jQuery.parseJSON(data); $("#editImpDialog").dialog({ width: 350, height: 220, modal: true, draggable: false, resizable: false, }); }, error: function (request, status, error) { alert(e); // TODO: need to discuss ajax error handling and form reset strategy. } }); }); $("#cancelEditImpBtn").click(function (e) { e.preventDefault(); $('#editImpDialog').dialog("close"); }); $("#saveEditImpBtn").click(function (e) { e.preventDefault(); $("form").submit(); });
I have a Dialog in my View. I need to Display the Json Data into the Jquery dialog. How can i do that?